PLEASE HELP ME MY CANCEL BUTTON AND TRY BUTTON DO THE SAME THING I WANT MY CANCEL BUTTON TO CLOSE MY PROGRAM/FRAME import java.awt.*; import javax.swing.*; import java.awt.event.*; import java.util.Scanner; public class oopfr implements MouseListener, KeyListener, ActionListener { private JPanel jp; private JFrame jf; private JTextField tf, z; private JLabel nm, jl, n, v, c; private JButton button, button2, button3; oopfr() { jp = new JPanel(); jp.setBackground(Color.YELLOW); jf = new JFrame("Consonant and Vowel Count"); jf.setSize(500, 500); jf.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); jf.add(jp); jf.addKeyListener(this); jp.setLayout(null); nm = new JLabel("Name: "); nm.setBounds(10, 20, 55, 20); jp.add(nm); z = new JTextField(); z.setBounds(100, 20, 130, 20); z.setBackground(Color.WHITE); z.addKeyListener(this); jp.add(z); button = new JButton("Try"); button.setBackground(Color.ORANGE); button.setBounds(260, 20, 80, 20); button.addActionListener(this); jp.add(button); button2 = new JButton("Retry"); button2.setBackground(Color.PINK); button2.setBounds(330, 20, 80, 20); button2.addMouseListener(this); jp.add(button2); button3 = new JButton("Cancel"); button3.setBackground(Color.MAGENTA); button3.setBounds(400, 20, 80, 20); button3.addActionListener(this); jp.add(button3); n = new JLabel("Name: "); n.setBounds(10, 40, 150, 50); jp.add(n); v = new JLabel("Vowels: "); v.setBounds(10, 60, 150, 50); jp.add(v); c = new JLabel("Consonants: "); c.setBounds(10, 80, 150, 50); jp.add(c); jf.setVisible(true); } public static void main(String args[]) { new oopfr(); } @Override public void actionPerformed(ActionEvent e) { // TODO Auto-generated method stub String str = z.getText(); str = str.toLowerCase(); int vowels = 0; int consonants = 0; n.setText("Name: " + str.toLowerCase()); str = str.toUpperCase(); for (int i = 0; i < str.length(); i++) { if (str.charAt(i) == 'A' || str.charAt(i) == 'E' || str.charAt(i) == 'I' || str.charAt(i) == 'O' || str.charAt(i) == 'U') { vowels++; } else if (str.charAt(i) >= 'A' && str.charAt(i) <= 'Z') { consonants++; } } v.setText("Vowels:" + vowels); c.setText("Consonants: " + consonants); } @Override public void keyTyped(KeyEvent e) { // TODO Auto-generated method stub System.out.println(" Key Typed: " + e.getKeyChar()); } @Override public void keyPressed(KeyEvent e) { // TODO Auto-generated method stub } @Override public void keyReleased(KeyEvent e) { // TODO Auto-generated method stub nm.setBackground(Color.WHITE); } @Override public void mouseClicked(MouseEvent e) { // TODO Auto-generated method stub n.setText("Name: "); v.setText("Vowels: "); c.setText("Consonants: "); } @Override public void mousePressed(MouseEvent e) { // TODO Auto-generated method stub System.out.println("Mouse Pressed"); z.setText(""); nm.setText("Name: "); button.setText("Try"); button2.setText("Retry"); button3.setText("Cancel"); } @Override public void mouseReleased(MouseEvent e) { // TODO Auto-generated method stub } @Override public void mouseEntered(MouseEvent e) { // TODO Auto-generated method stub } @Override public void mouseExited(MouseEvent e) { // TODO Auto-generated method stub } }
PLEASE HELP ME MY CANCEL BUTTON AND TRY BUTTON DO THE SAME THING I WANT MY CANCEL BUTTON TO CLOSE MY PROGRAM/FRAME import java.awt.*; import javax.swing.*; import java.awt.event.*; import java.util.Scanner; public class oopfr implements MouseListener, KeyListener, ActionListener { private JPanel jp; private JFrame jf; private JTextField tf, z; private JLabel nm, jl, n, v, c; private JButton button, button2, button3; oopfr() { jp = new JPanel(); jp.setBackground(Color.YELLOW); jf = new JFrame("Consonant and Vowel Count"); jf.setSize(500, 500); jf.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); jf.add(jp); jf.addKeyListener(this); jp.setLayout(null); nm = new JLabel("Name: "); nm.setBounds(10, 20, 55, 20); jp.add(nm); z = new JTextField(); z.setBounds(100, 20, 130, 20); z.setBackground(Color.WHITE); z.addKeyListener(this); jp.add(z); button = new JButton("Try"); button.setBackground(Color.ORANGE); button.setBounds(260, 20, 80, 20); button.addActionListener(this); jp.add(button); button2 = new JButton("Retry"); button2.setBackground(Color.PINK); button2.setBounds(330, 20, 80, 20); button2.addMouseListener(this); jp.add(button2); button3 = new JButton("Cancel"); button3.setBackground(Color.MAGENTA); button3.setBounds(400, 20, 80, 20); button3.addActionListener(this); jp.add(button3); n = new JLabel("Name: "); n.setBounds(10, 40, 150, 50); jp.add(n); v = new JLabel("Vowels: "); v.setBounds(10, 60, 150, 50); jp.add(v); c = new JLabel("Consonants: "); c.setBounds(10, 80, 150, 50); jp.add(c); jf.setVisible(true); } public static void main(String args[]) { new oopfr(); } @Override public void actionPerformed(ActionEvent e) { // TODO Auto-generated method stub String str = z.getText(); str = str.toLowerCase(); int vowels = 0; int consonants = 0; n.setText("Name: " + str.toLowerCase()); str = str.toUpperCase(); for (int i = 0; i < str.length(); i++) { if (str.charAt(i) == 'A' || str.charAt(i) == 'E' || str.charAt(i) == 'I' || str.charAt(i) == 'O' || str.charAt(i) == 'U') { vowels++; } else if (str.charAt(i) >= 'A' && str.charAt(i) <= 'Z') { consonants++; } } v.setText("Vowels:" + vowels); c.setText("Consonants: " + consonants); } @Override public void keyTyped(KeyEvent e) { // TODO Auto-generated method stub System.out.println(" Key Typed: " + e.getKeyChar()); } @Override public void keyPressed(KeyEvent e) { // TODO Auto-generated method stub } @Override public void keyReleased(KeyEvent e) { // TODO Auto-generated method stub nm.setBackground(Color.WHITE); } @Override public void mouseClicked(MouseEvent e) { // TODO Auto-generated method stub n.setText("Name: "); v.setText("Vowels: "); c.setText("Consonants: "); } @Override public void mousePressed(MouseEvent e) { // TODO Auto-generated method stub System.out.println("Mouse Pressed"); z.setText(""); nm.setText("Name: "); button.setText("Try"); button2.setText("Retry"); button3.setText("Cancel"); } @Override public void mouseReleased(MouseEvent e) { // TODO Auto-generated method stub } @Override public void mouseEntered(MouseEvent e) { // TODO Auto-generated method stub } @Override public void mouseExited(MouseEvent e) { // TODO Auto-generated method stub } }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
PLEASE HELP ME MY CANCEL BUTTON AND TRY BUTTON DO THE SAME THING I WANT MY CANCEL BUTTON TO CLOSE MY PROGRAM/FRAME
import java.awt.*;
import javax.swing.*;
import java.awt.event.*;
import java.util.Scanner;
public class oopfr implements MouseListener, KeyListener, ActionListener {
private JPanel jp;
private JFrame jf;
private JTextField tf, z;
private JLabel nm, jl, n, v, c;
private JButton button, button2, button3;
oopfr() {
jp = new JPanel();
jp.setBackground(Color.YELLOW);
jf = new JFrame("Consonant and Vowel Count");
jf.setSize(500, 500);
jf.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
jf.add(jp);
jf.addKeyListener(this);
jp.setLayout(null);
nm = new JLabel("Name: ");
nm.setBounds(10, 20, 55, 20);
jp.add(nm);
z = new JTextField();
z.setBounds(100, 20, 130, 20);
z.setBackground(Color.WHITE);
z.addKeyListener(this);
jp.add(z);
button = new JButton("Try");
button.setBackground(Color.ORANGE);
button.setBounds(260, 20, 80, 20);
button.addActionListener(this);
jp.add(button);
button2 = new JButton("Retry");
button2.setBackground(Color.PINK);
button2.setBounds(330, 20, 80, 20);
button2.addMouseListener(this);
jp.add(button2);
button3 = new JButton("Cancel");
button3.setBackground(Color.MAGENTA);
button3.setBounds(400, 20, 80, 20);
button3.addActionListener(this);
jp.add(button3);
n = new JLabel("Name: ");
n.setBounds(10, 40, 150, 50);
jp.add(n);
v = new JLabel("Vowels: ");
v.setBounds(10, 60, 150, 50);
jp.add(v);
c = new JLabel("Consonants: ");
c.setBounds(10, 80, 150, 50);
jp.add(c);
jf.setVisible(true);
}
public static void main(String args[]) {
new oopfr();
}
@Override
public void actionPerformed(ActionEvent e) {
// TODO Auto-generated method stub
String str = z.getText();
str = str.toLowerCase();
int vowels = 0;
int consonants = 0;
n.setText("Name: " + str.toLowerCase());
str = str.toUpperCase();
for (int i = 0; i < str.length(); i++) {
if (str.charAt(i) == 'A' || str.charAt(i) == 'E' || str.charAt(i) == 'I' || str.charAt(i) == 'O'
|| str.charAt(i) == 'U') {
vowels++;
} else if (str.charAt(i) >= 'A' && str.charAt(i) <= 'Z') {
consonants++;
}
}
v.setText("Vowels:" + vowels);
c.setText("Consonants: " + consonants);
}
@Override
public void keyTyped(KeyEvent e) {
// TODO Auto-generated method stub
System.out.println(" Key Typed: " + e.getKeyChar());
}
@Override
public void keyPressed(KeyEvent e) {
// TODO Auto-generated method stub
}
@Override
public void keyReleased(KeyEvent e) {
// TODO Auto-generated method stub
nm.setBackground(Color.WHITE);
}
@Override
public void mouseClicked(MouseEvent e) {
// TODO Auto-generated method stub
n.setText("Name: ");
v.setText("Vowels: ");
c.setText("Consonants: ");
}
@Override
public void mousePressed(MouseEvent e) {
// TODO Auto-generated method stub
System.out.println("Mouse Pressed");
z.setText("");
nm.setText("Name: ");
button.setText("Try");
button2.setText("Retry");
button3.setText("Cancel");
}
@Override
public void mouseReleased(MouseEvent e) {
// TODO Auto-generated method stub
}
@Override
public void mouseEntered(MouseEvent e) {
// TODO Auto-generated method stub
}
@Override
public void mouseExited(MouseEvent e) {
// TODO Auto-generated method stub
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-engineering and related others by exploring similar questions and additional content below.Recommended textbooks for you
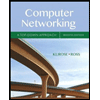
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
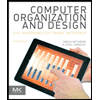
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
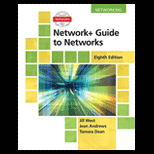
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
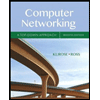
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
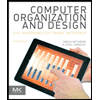
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
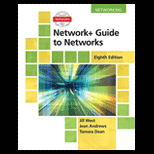
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
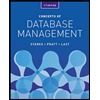
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
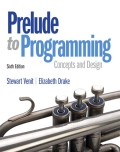
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
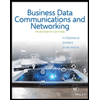
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY