can someone help me resize the picture in Java. This is my code: import java.awt.*; import java.awt.image.BufferedImage; import java.io.File; import java.io.IOException; import javax.imageio.ImageIO; class ImageDisplayGUI { public static void main(String[] args) { SwingUtilities.invokeLater(() -> { createAndShowGUI(); }); } private static void createAndShowGUI() { JFrame frame = new JFrame("Bears"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(800, 600); frame.setLayout(new BorderLayout()); // Load and display the JPG image try { BufferedImage image = ImageIO.read(new File("two bears.png")); // Replace with your image path ImageIcon icon = new ImageIcon(image); JLabel imageLabel = new JLabel(icon); frame.add(imageLabel, BorderLayout.CENTER); } catch (IOException e) { // Handle image loading error e.printStackTrace(); } frame.setVisible(true); } }
can someone help me resize the picture in Java. This is my code:
import java.awt.*;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
class ImageDisplayGUI {
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
createAndShowGUI();
});
}
private static void createAndShowGUI() {
JFrame frame = new JFrame("Bears");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(800, 600);
frame.setLayout(new BorderLayout());
// Load and display the JPG image
try {
BufferedImage image = ImageIO.read(new File("two bears.png")); // Replace with your image path
ImageIcon icon = new ImageIcon(image);
JLabel imageLabel = new JLabel(icon);
frame.add(imageLabel, BorderLayout.CENTER);
} catch (IOException e) {
// Handle image loading error
e.printStackTrace();
}
frame.setVisible(true);
}
}



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

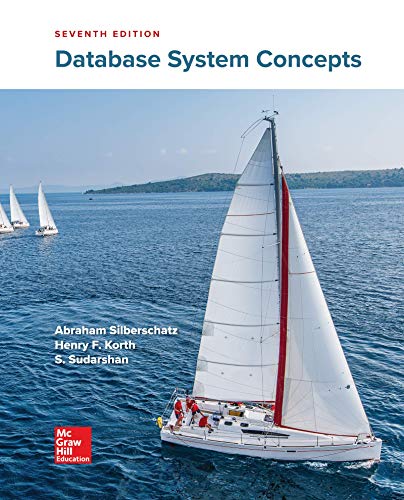
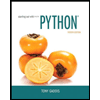
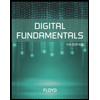
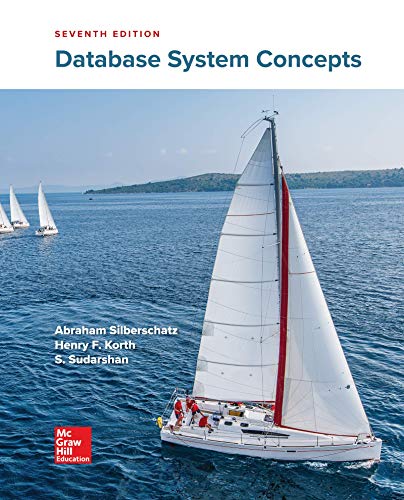
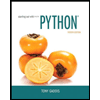
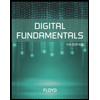
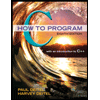
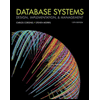
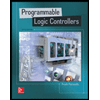