MyFrame Demo Left Middle - Right X Implement Java code in the constructor of MyFrame below to create a GUI like an image above. No event listeners and handlers are required. Class MyFrame extends JFrame { private JButton] buttons;
MyFrame Demo Left Middle - Right X Implement Java code in the constructor of MyFrame below to create a GUI like an image above. No event listeners and handlers are required. Class MyFrame extends JFrame { private JButton] buttons;
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
![## MyFrame Demo
The image demonstrates a simple Java Swing application named "MyFrame Demo." At the top of the application window, there are three buttons labeled "Left," "Middle," and "Right." Below is the Java code snippet that creates a GUI similar to what is shown in the image.
### Code Explanation:
```java
// Implement Java code in the constructor of MyFrame below to create a GUI like the image above.
// No event listeners and handlers are required.
class MyFrame extends JFrame {
private JButton[] buttons;
public MyFrame() {
super("MyFrame Demo");
// Add the implementation for the GUI here
}
}
```
### Detailed Code Breakdown:
- **Class Declaration:**
- The class `MyFrame` extends `JFrame`, indicating it is a frame/window in a Swing application.
- **Private Member:**
- `private JButton[] buttons;` is declared but not initialized. This will store the array of buttons.
- **Constructor:**
- The constructor `MyFrame()` calls the superclass constructor with the title "MyFrame Demo".
- Placeholders are given for adding the necessary GUI implementation to match the image above.
### Creating the GUI:
To create the GUI as shown in the image, you would need to:
1. Initialize the `buttons` array with three `JButton` components.
2. Set the layout of the frame, likely using a `FlowLayout` to align the buttons horizontally.
3. Add the buttons to the frame.
Here is a potential implementation:
```java
import javax.swing.*;
public class MyFrame extends JFrame {
private JButton[] buttons;
public MyFrame() {
super("MyFrame Demo");
buttons = new JButton[3];
buttons[0] = new JButton("Left");
buttons[1] = new JButton("Middle");
buttons[2] = new JButton("Right");
setLayout(new FlowLayout());
for (JButton button : buttons) {
add(button);
}
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(300, 100);
setVisible(true);
}
public static void main(String[] args) {
new MyFrame();
}
}
```
### Explanation of Implementation:
1. **Initialization:**
- Create three `JButton` instances labeled "Left," "Middle," and "Right."
2. **Layout Setting:**](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F03256047-c4d6-4acb-b3bb-18185f2c131a%2F5908976b-914d-4664-bd25-a5cf6230623d%2F729s9y8_processed.png&w=3840&q=75)
Transcribed Image Text:## MyFrame Demo
The image demonstrates a simple Java Swing application named "MyFrame Demo." At the top of the application window, there are three buttons labeled "Left," "Middle," and "Right." Below is the Java code snippet that creates a GUI similar to what is shown in the image.
### Code Explanation:
```java
// Implement Java code in the constructor of MyFrame below to create a GUI like the image above.
// No event listeners and handlers are required.
class MyFrame extends JFrame {
private JButton[] buttons;
public MyFrame() {
super("MyFrame Demo");
// Add the implementation for the GUI here
}
}
```
### Detailed Code Breakdown:
- **Class Declaration:**
- The class `MyFrame` extends `JFrame`, indicating it is a frame/window in a Swing application.
- **Private Member:**
- `private JButton[] buttons;` is declared but not initialized. This will store the array of buttons.
- **Constructor:**
- The constructor `MyFrame()` calls the superclass constructor with the title "MyFrame Demo".
- Placeholders are given for adding the necessary GUI implementation to match the image above.
### Creating the GUI:
To create the GUI as shown in the image, you would need to:
1. Initialize the `buttons` array with three `JButton` components.
2. Set the layout of the frame, likely using a `FlowLayout` to align the buttons horizontally.
3. Add the buttons to the frame.
Here is a potential implementation:
```java
import javax.swing.*;
public class MyFrame extends JFrame {
private JButton[] buttons;
public MyFrame() {
super("MyFrame Demo");
buttons = new JButton[3];
buttons[0] = new JButton("Left");
buttons[1] = new JButton("Middle");
buttons[2] = new JButton("Right");
setLayout(new FlowLayout());
for (JButton button : buttons) {
add(button);
}
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(300, 100);
setVisible(true);
}
public static void main(String[] args) {
new MyFrame();
}
}
```
### Explanation of Implementation:
1. **Initialization:**
- Create three `JButton` instances labeled "Left," "Middle," and "Right."
2. **Layout Setting:**
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Recommended textbooks for you
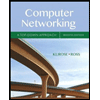
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
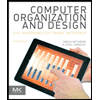
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
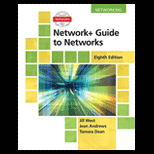
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
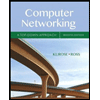
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
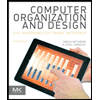
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
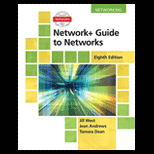
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
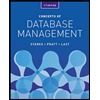
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
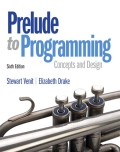
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
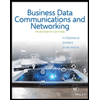
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY