eate a matching game using java and 2D arrays. Create a reset button and title
eate a matching game using java and 2D arrays. Create a reset button and title
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please help me create a matching game using java and 2D arrays. Create a reset button and title
![### Transcription and Explanation for Educational Use
#### Images and Grid Layout
**Image Descriptions:**
- The scene presents a grid and a series of icon images designed for a memory game application with a Halloween theme.
- The grid on the left displays a 4x3 arrangement of Halloween-themed icons with different facial expressions and designs, such as a pumpkin and various monsters or characters.
- To the right, we see the back-facing side of the cards, nine in total, which are uniformly marked with a red pattern.
- Below these grids are individual icons labeled `back.png`, `hal1.png`, `hal2.png`, `hal3.png`, `hal4.png`, `hal5.png`, and `hal6.png`, corresponding to the images used in the game.
#### Starter Code (Java)
The following is a starter code for implementing a memory game applet using Java's AWT and Swing libraries. This example demonstrates setting up a grid layout of images:
```java
import javax.swing.*;
import java.applet.*;
import java.awt.event.*;
import java.awt.*;
public class halloween extends Applet implements ActionListener {
int hallow[] [] = {};
int rows = 4;
int cols = 3;
JButton pics[] = new JButton [rows * cols];
public void init () {
Panel grid = new Panel (new GridLayout (rows, cols));
int m = 0;
for (int i = 0; i < rows ; i++) {
for (int j = 0 ; j < cols ; j++) {
pics [m] = new JButton (createImageIcon ("back.png"));
pics [m].addActionListener (this);
pics [m].setActionCommand (m + "");
pics [m].setPreferredSize (new Dimension (128, 128));
grid.add (pics [m]);
}
}
}
}
```
**Explanation:**
- **Imports:** The code imports necessary classes for GUI components and event handling (`javax.swing`, `java.awt`, and others).
- **Class Definition:** A class `halloween` extends `Applet` and implements `ActionListener` for handling button clicks.
- **Variables:** Initializes a 2D integer array `hallow` and sets up the grid dimensions (`rows` and `cols`). An array of `JButton` is declared to hold the buttons representing the game cards.
- **](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd298be25-ffcb-47a1-b62e-d3a828be4885%2Fe312b098-af17-41c2-bc69-da9bcdb73093%2Fm591y8j_processed.jpeg&w=3840&q=75)
Transcribed Image Text:### Transcription and Explanation for Educational Use
#### Images and Grid Layout
**Image Descriptions:**
- The scene presents a grid and a series of icon images designed for a memory game application with a Halloween theme.
- The grid on the left displays a 4x3 arrangement of Halloween-themed icons with different facial expressions and designs, such as a pumpkin and various monsters or characters.
- To the right, we see the back-facing side of the cards, nine in total, which are uniformly marked with a red pattern.
- Below these grids are individual icons labeled `back.png`, `hal1.png`, `hal2.png`, `hal3.png`, `hal4.png`, `hal5.png`, and `hal6.png`, corresponding to the images used in the game.
#### Starter Code (Java)
The following is a starter code for implementing a memory game applet using Java's AWT and Swing libraries. This example demonstrates setting up a grid layout of images:
```java
import javax.swing.*;
import java.applet.*;
import java.awt.event.*;
import java.awt.*;
public class halloween extends Applet implements ActionListener {
int hallow[] [] = {};
int rows = 4;
int cols = 3;
JButton pics[] = new JButton [rows * cols];
public void init () {
Panel grid = new Panel (new GridLayout (rows, cols));
int m = 0;
for (int i = 0; i < rows ; i++) {
for (int j = 0 ; j < cols ; j++) {
pics [m] = new JButton (createImageIcon ("back.png"));
pics [m].addActionListener (this);
pics [m].setActionCommand (m + "");
pics [m].setPreferredSize (new Dimension (128, 128));
grid.add (pics [m]);
}
}
}
}
```
**Explanation:**
- **Imports:** The code imports necessary classes for GUI components and event handling (`javax.swing`, `java.awt`, and others).
- **Class Definition:** A class `halloween` extends `Applet` and implements `ActionListener` for handling button clicks.
- **Variables:** Initializes a 2D integer array `hallow` and sets up the grid dimensions (`rows` and `cols`). An array of `JButton` is declared to hold the buttons representing the game cards.
- **
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
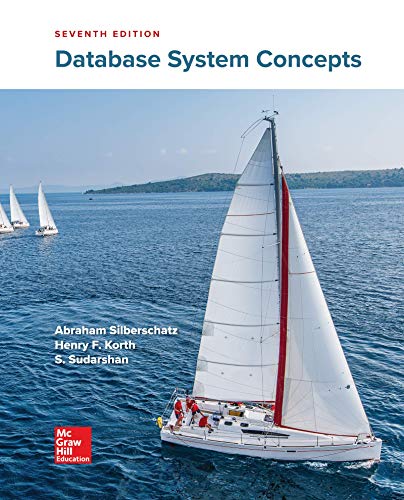
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
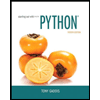
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
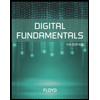
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
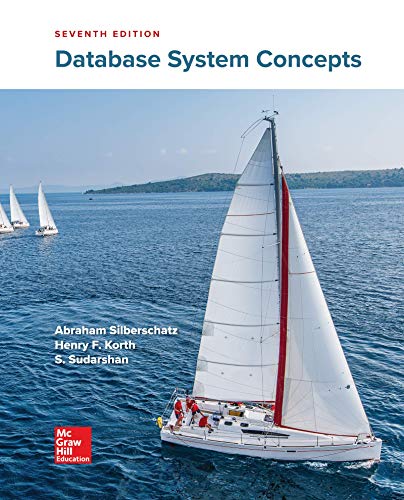
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
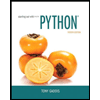
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
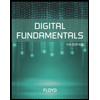
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
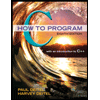
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
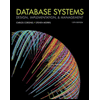
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
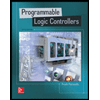
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education