I know it is a lot but can someone explain (with comments) line by line what is happening in the below Java program. It assists in my learning/gives me a deeper understanding. Please and thank you! Source code: import javax.swing.*; import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.SwingUtilities; class ProductCalculator { public static int calculateProduct(int[] numbers, int index) { if (index == numbers.length - 1) { return numbers[index]; } else { return numbers[index] * calculateProduct(numbers, index + 1); } } } class RecursiveProductCalculatorView extends JFrame { private JTextField[] numberFields; private JButton calculateButton; private JLabel resultLabel; public RecursiveProductCalculatorView() { setTitle("Recursive Product Calculator"); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setLayout(new GridLayout(7, 1)); numberFields = new JTextField[5]; for (int i = 0; i < 5; i++) { JPanel panel = new JPanel(); panel.setLayout(new FlowLayout()); JLabel label = new JLabel("Number " + (i + 1) + ": "); numberFields[i] = new JTextField(10); panel.add(label); panel.add(numberFields[i]); add(panel); } calculateButton = new JButton("Calculate"); calculateButton.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { int[] numbers = new int[5]; for (int i = 0; i < 5; i++) { // Perform validation for each input field if (!numberFields[i].getText().matches("-?\\d+")) { JOptionPane.showMessageDialog(null, "Invalid input in Number " + (i + 1) + "."); return; } numbers[i] = Integer.parseInt(numberFields[i].getText()); } int product = ProductCalculator.calculateProduct(numbers, 0); resultLabel.setText("Product: " + product); } }); add(calculateButton); resultLabel = new JLabel("Product: "); add(resultLabel); pack(); setVisible(true); } } public class RecursiveProductCalculator { public static void main(String[] args) { SwingUtilities.invokeLater(new Runnable() { @Override public void run() { new RecursiveProductCalculatorView(); } }); } }
I know it is a lot but can someone explain (with comments) line by line what is happening in the below Java program. It assists in my learning/gives me a deeper understanding. Please and thank you!
Source code:
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.SwingUtilities;
class ProductCalculator {
public static int calculateProduct(int[] numbers, int index) {
if (index == numbers.length - 1) {
return numbers[index];
} else {
return numbers[index] * calculateProduct(numbers, index + 1);
}
}
}
class RecursiveProductCalculatorView extends JFrame {
private JTextField[] numberFields;
private JButton calculateButton;
private JLabel resultLabel;
public RecursiveProductCalculatorView() {
setTitle("Recursive Product Calculator");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new GridLayout(7, 1));
numberFields = new JTextField[5];
for (int i = 0; i < 5; i++) {
JPanel panel = new JPanel();
panel.setLayout(new FlowLayout());
JLabel label = new JLabel("Number " + (i + 1) + ": ");
numberFields[i] = new JTextField(10);
panel.add(label);
panel.add(numberFields[i]);
add(panel);
}
calculateButton = new JButton("Calculate");
calculateButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int[] numbers = new int[5];
for (int i = 0; i < 5; i++) {
// Perform validation for each input field
if (!numberFields[i].getText().matches("-?\\d+")) {
JOptionPane.showMessageDialog(null, "Invalid input in Number " + (i + 1) + ".");
return;
}
numbers[i] = Integer.parseInt(numberFields[i].getText());
}
int product = ProductCalculator.calculateProduct(numbers, 0);
resultLabel.setText("Product: " + product);
}
});
add(calculateButton);
resultLabel = new JLabel("Product: ");
add(resultLabel);
pack();
setVisible(true);
}
}
public class RecursiveProductCalculator {
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
new RecursiveProductCalculatorView();
}
});
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

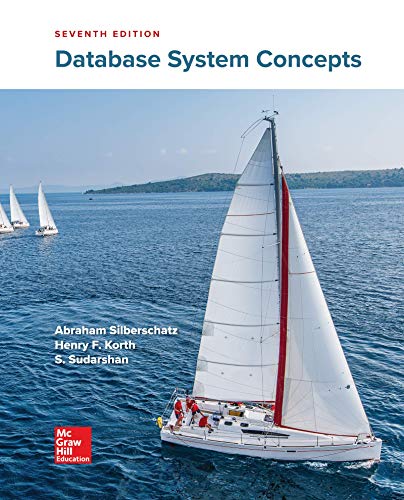
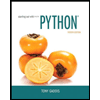
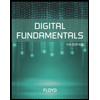
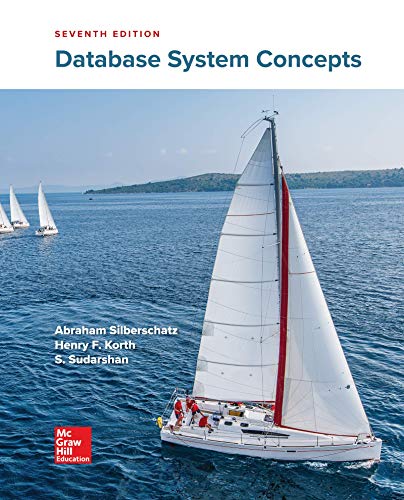
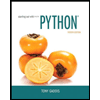
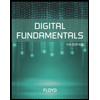
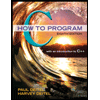
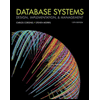
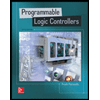