I'm stuck on my code and need help rewriting it so there are no syntax errors. Here's my code. I've also included two pictures - one of my syntax errors (there are 11) and another of what the output of the code should look like. Please try to keep the code as similar to the original as possible.
I'm stuck on my code and need help rewriting it so there are no syntax errors. Here's my code. I've also included two pictures - one of my syntax errors (there are 11) and another of what the output of the code should look like. Please try to keep the code as similar to the original as possible.
CODE
//Importing scanner
import java.util.Scanner;
public class CarMaintanenceAssignment
{
//Method 1
public static double carMaintenance(String carMake)
{
Scanner input = new Scanner(System.in);
double accum = 0;
char yn;
System.out.println(" Services: Oil Change, Tune up, Brake Job, Transmission Service, respectively: 59.99, 111.99, 189.99, 149.99");
System.out.println(" ");
//Prompting user for the make of their car
System.out.println("Please select the service that you want for your " + carMake);
//Asking user if they want an oil change
if(yn.equalsIgnoreCase('Y'))
{
accum += 59.99;
}
System.out.println("Coolant Flush : Please enter Y or N =");
yn = sc.next().charAt(0);
if(yn.equalsIgnoreCase('Y'))
{
accum += 111.99;
}
System.out.println("Brake Job : Please enter Y or N =");
yn = sc.next().charAt(0);
if(yn.equalsIgnoreCase('Y'))
{
accum += 189.99;
}
System.out.println(" Tune Up : Please enter Y or N =");
yn = sc.next().charAt(0);
if(yn.equalsIgnoreCase('Y'))
{
carMaintanancePrice += 149.99;
}
return accum;
//Method 2
public static void calcFinalPrice(double accum, Boolean carImport)
{
double labor = 0.25 * accum;
accum += labor;
//If statement for car import
if(carImport)
{
accum += 0.06 * accum;
}
accum += 0.07 * accum;
//Outputting final price
System.out.println("The Final Price with Taxes and Labor = " +accum);
}
public static void main(String [] args)
{
Scanner input = new Scanner(System.in);
String carMake;
String answer;
boolean carImport = false;
//Prompting user to enter the make of their car
System.out.println("Enter the Make of your Car?");
carMake = input.nextLine();
//Asking user if their car is an import or not
System.out.println("Is your Car an Import Model?");
answer = input.nextLine();
if(answer.equalsIgnoreCase("yes"))
{
carImport = true;
}
//Calling method 1 for accum
double accum = carMaintenance(carMake);
System.out.println("Total price before Tax and Labor = " + accum);
//Calling method 2 for final price
calcFinalPrice(accum, carImport);
}
}
}
- END OF CODE -
![CarMaintanenceAssignment.java:49: error: illegal start of expression
public static void calcfinalPrice(double accum, Boolean carImport)
CarMaintanenceAssignment.java:49: error: illegal start of expression
public static void calcfinalPrice(double accum, Boolean carImport)
CarMaintanenceAssignment.java:49: error: ';' expected
public static void calcfinalPrice(double accum, Boolean carImport)
.class' expected
CarMaintanenceAssignment.java:49: error:
public static void calcfinalPrice(double accum, Boolean carImport)
CarMaintanenceAssignment.java:49: error: ';' expected
public static void calcfinalPrice(double accum, Boolean carImport)
CarMaintanenceAssignment.java: 49: error: ';' expected
public static void calcfinalPrice(double accum, Boolean carImport)
CarMaintanenceAssignment.java:66: error: illegal start of expression
public static void main(String [] args)
CarMaintanenceAssignment.java:66: error: illegal start of expression
public static void main(String [] args)
CarMaintanenceAssignment.java: 66: error: ';' expected
public static void main(String [] args)
.class' expected
CarMaintanenceAssignment.java:66: error:
public static void main(String [] args)
CarMaintanenceAssignment.java:66: error: ';' expected
public static void main(String [] args)
11 errorS](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7a3705a7-be95-49e3-a651-a8d1e003632f%2F4d1e89b9-4fd0-4f94-9eec-1416f161c1b8%2Fbfejyag_processed.jpeg&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

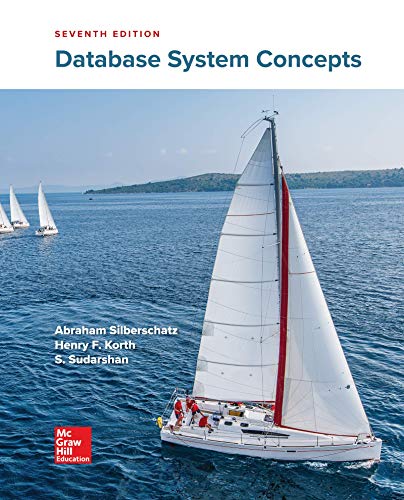
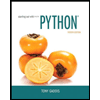
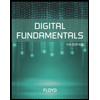
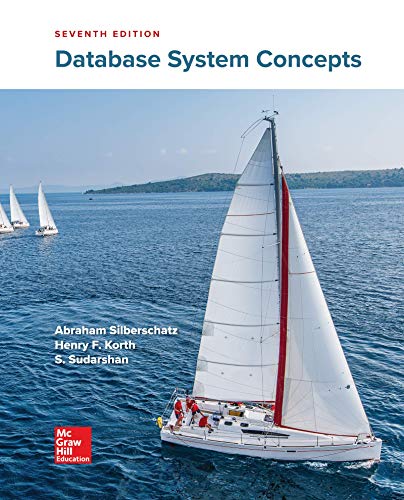
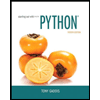
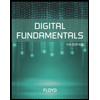
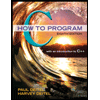
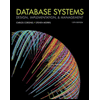
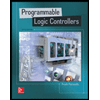