Please help and thank you in advance. The language is Java. I have to write a program that takes and validates a date but it won't output the full date. Here is my current code: import java.util.Scanner; public class P1 { publicstaticvoidmain(String[]args) { // project 1 // date input // date output // Create a Scanner object to read from the keyboard. Scannerkeyboard=newScanner(System.in); // Identifier declarations charmonth; intday; intyear; booleanisLeapyear; StringmonthName; intmaxDay; // Asks user to input date System.out.println("Please enter month: "); month=(char)keyboard.nextInt(); System.out.println("Please enter day: "); day=keyboard.nextInt(); System.out.println("Please enter year: "); year=keyboard.nextInt(); // Formating months switch(month){ case1: monthName="January"; break; case2: monthName="February"; break; case3: monthName="March"; break; case4: monthName="April"; break; case5: monthName="May"; break; case6: monthName="June"; break; case7: monthName="July"; break; case8: monthName="August"; break; case9: monthName="September"; break; case10: monthName="October"; break; case11: monthName="November"; break; case12: monthName="December"; break; default:monthName="Invalid month"; System.out.println("The month is " +monthName); // The second version must accept any integer between 1 and 12 if(month>=1&&month<=12) {break; }else{System.out.println("The month you have enertred in is invalid," +"please try again"); } // Formating days if(month==1||month==3||month==5||month==7|| month==8||month==10||month==12) maxDay=31; elseif(month==4||month==6||month==9||month==11) maxDay=30; elseif(month==2); maxDay=28; // validate input if(maxDay>=1&&maxDay<=31) System.out.println("That date is valid"); else System.out.println("That number is invalid."); System.out.print("Enter a number in the " + "range of 1 through 31: "); maxDay=keyboard.nextInt(); // Formating year if(year>=1&&year<=99){ if(month==2&&day==29){ if((year+2000)%4==0){ break; }else{ System.out.println("Leap year, Cannot contain 29 days. Enter valid year again!"); } }else{ break; } } // Leap year isLeapyear=(year%4==0); isLeapyear=isLeapyear&&(year%100!=0); isLeapyear=isLeapyear||(year%400==0); System.out.println(isLeapyear); // Output of full date System.out.println(month + " " + day + ", " + year); } } }
Please help and thank you in advance.
The language is Java.
I have to write a program that takes and validates a date but it won't output the full date.
Here is my current code:
import java.util.Scanner;
public class P1
{
publicstaticvoidmain(String[]args)
{
// project 1
// date input
// date output
// Create a Scanner object to read from the keyboard.
Scannerkeyboard=newScanner(System.in);
// Identifier declarations
charmonth;
intday;
intyear;
booleanisLeapyear;
StringmonthName;
intmaxDay;
// Asks user to input date
System.out.println("Please enter month: ");
month=(char)keyboard.nextInt();
System.out.println("Please enter day: ");
day=keyboard.nextInt();
System.out.println("Please enter year: ");
year=keyboard.nextInt();
// Formating months
switch(month){
case1:
monthName="January";
break;
case2:
monthName="February";
break;
case3:
monthName="March";
break;
case4:
monthName="April";
break;
case5:
monthName="May";
break;
case6:
monthName="June";
break;
case7:
monthName="July";
break;
case8:
monthName="August";
break;
case9:
monthName="September";
break;
case10:
monthName="October";
break;
case11:
monthName="November";
break;
case12:
monthName="December";
break;
default:monthName="Invalid month";
System.out.println("The month is "
+monthName);
// The second version must accept any integer between 1 and 12
if(month>=1&&month<=12)
{break;
}else{System.out.println("The month you have enertred in is invalid,"
+"please try again");
}
// Formating days
if(month==1||month==3||month==5||month==7||
month==8||month==10||month==12)
maxDay=31;
elseif(month==4||month==6||month==9||month==11)
maxDay=30;
elseif(month==2);
maxDay=28;
// validate input
if(maxDay>=1&&maxDay<=31)
System.out.println("That date is valid");
else
System.out.println("That number is invalid.");
System.out.print("Enter a number in the " + "range of 1 through 31: ");
maxDay=keyboard.nextInt();
// Formating year
if(year>=1&&year<=99){
if(month==2&&day==29){
if((year+2000)%4==0){
break;
}else{
System.out.println("Leap year, Cannot contain 29 days. Enter valid year again!");
}
}else{
break;
}
}
// Leap year
isLeapyear=(year%4==0);
isLeapyear=isLeapyear&&(year%100!=0);
isLeapyear=isLeapyear||(year%400==0);
System.out.println(isLeapyear);
// Output of full date
System.out.println(month + " " + day + ", " + year);
}
}
}


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

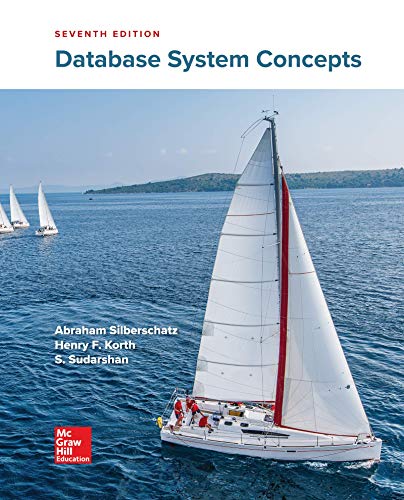
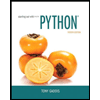
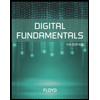
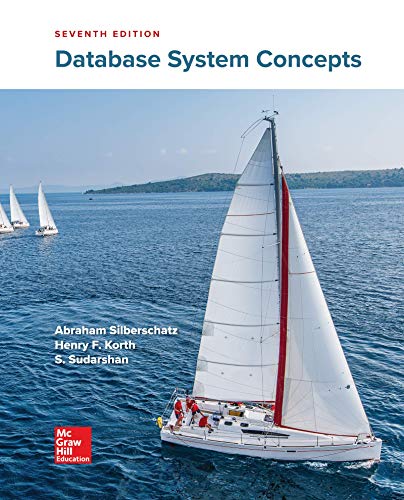
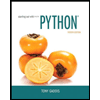
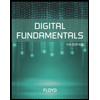
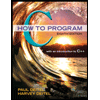
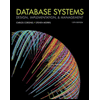
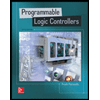