AirlineDriver.java X AirlineDriver1.java 7/Scanner class for user input Scanner sc = new Scanner(System.in); //String seat = ""; System.out.println("Enter a row and a seat, please: "); String seat= sc.nextLine(); int r = 0; char c = do { seat = getUserInput (sc); 6000 if (seat. length() ==3){ r = seat.charAt(0)-"0"; c = seat.charAt(2); } if(Character.isLowerCase(c)) { c = Character.toUpperCase(c ); checkAndBookSeat1 (r, c, layout); displaySeats (layout); } } else { 1 if { } (!seat.equals("-1")) I System.out.println("Invalid!"); System.out.println("Row value [1-5] and col[A-D]"); //tem.out.println("You have reached end of the program."); while (!seat.equals("-1"));
AirlineDriver.java X AirlineDriver1.java 7/Scanner class for user input Scanner sc = new Scanner(System.in); //String seat = ""; System.out.println("Enter a row and a seat, please: "); String seat= sc.nextLine(); int r = 0; char c = do { seat = getUserInput (sc); 6000 if (seat. length() ==3){ r = seat.charAt(0)-"0"; c = seat.charAt(2); } if(Character.isLowerCase(c)) { c = Character.toUpperCase(c ); checkAndBookSeat1 (r, c, layout); displaySeats (layout); } } else { 1 if { } (!seat.equals("-1")) I System.out.println("Invalid!"); System.out.println("Row value [1-5] and col[A-D]"); //tem.out.println("You have reached end of the program."); while (!seat.equals("-1"));
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Why do I have so many errors (syntax errors) ? It definitely doesn't work in mine. Can someone fix the problem?
![This image displays a section of Java code from a file named `AirlineDriver.java`, which is part of a project for an educational course, likely related to programming. The code involves seat booking functionality in an airline seating system. Below is the transcription of the code visible in the image:
```java
private static void checkAndBookSeat1(int row, char col, char[] seat) {
// check for seat availability
if (row >= 1 && row <= 5) {
if (col >= 'A' && col <= 'D') {
int asciiA = 'A';
int asciiCol = col;
if (seat[row-1][asciiCol-asciiA] == 'O') {
seat[row-1][asciiCol-asciiA] = 'X';
} else {
System.out.println("This seat is not available.");
}
}
}
}
private static String getUserInput(Scanner sc) {
System.out.print("Enter a row and seat: ");
String seat = sc.nextLine();
return seat;
}
private static void checkAndBookSeat(int r, char c, char[][] layout) {
// TODO Auto-generated method stub
}
```
### Explanation:
- **`checkAndBookSeat1` Method:**
- This method checks if a seat is available and books it if it is.
- It takes three parameters: `row` (an integer representing the row number), `col` (a character representing the seat column), and `seat` (an array representing the seating layout).
- The method first checks if the row is between 1 and 5. If the column is between 'A' and 'D', it proceeds to check if the seat is available (represented by 'O'). If available, it marks it as booked ('X'). If not available, it prints a message.
- **`getUserInput` Method:**
- This method prompts the user to enter a row and seat.
- It uses a `Scanner` object to read the user's input from the console and returns it as a string.
- **`checkAndBookSeat` Method:**
- This is an auto-generated method stub that is not yet complete. It is intended to take three parameters similar to `checkAndBookSeat1`, but the functionality has not been implemented.
There are no graphs or diagrams in the image.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fafd3eb79-3297-4398-8445-49c2030e1595%2F63e2936c-554e-442a-82b7-3379650eeef1%2F7s59mys_processed.jpeg&w=3840&q=75)
Transcribed Image Text:This image displays a section of Java code from a file named `AirlineDriver.java`, which is part of a project for an educational course, likely related to programming. The code involves seat booking functionality in an airline seating system. Below is the transcription of the code visible in the image:
```java
private static void checkAndBookSeat1(int row, char col, char[] seat) {
// check for seat availability
if (row >= 1 && row <= 5) {
if (col >= 'A' && col <= 'D') {
int asciiA = 'A';
int asciiCol = col;
if (seat[row-1][asciiCol-asciiA] == 'O') {
seat[row-1][asciiCol-asciiA] = 'X';
} else {
System.out.println("This seat is not available.");
}
}
}
}
private static String getUserInput(Scanner sc) {
System.out.print("Enter a row and seat: ");
String seat = sc.nextLine();
return seat;
}
private static void checkAndBookSeat(int r, char c, char[][] layout) {
// TODO Auto-generated method stub
}
```
### Explanation:
- **`checkAndBookSeat1` Method:**
- This method checks if a seat is available and books it if it is.
- It takes three parameters: `row` (an integer representing the row number), `col` (a character representing the seat column), and `seat` (an array representing the seating layout).
- The method first checks if the row is between 1 and 5. If the column is between 'A' and 'D', it proceeds to check if the seat is available (represented by 'O'). If available, it marks it as booked ('X'). If not available, it prints a message.
- **`getUserInput` Method:**
- This method prompts the user to enter a row and seat.
- It uses a `Scanner` object to read the user's input from the console and returns it as a string.
- **`checkAndBookSeat` Method:**
- This is an auto-generated method stub that is not yet complete. It is intended to take three parameters similar to `checkAndBookSeat1`, but the functionality has not been implemented.
There are no graphs or diagrams in the image.
![Below is the transcription of the code from the image, suitable for an educational website:
```java
// Scanner class for user input
Scanner sc = new Scanner(System.in);
// String seat = "";
System.out.println("Enter a row and a seat, please: ");
String seat = sc.nextLine();
int r = 0;
char c = '';
do {
seat = getUserInput(sc);
if (seat.length() == 3) {
r = seat.charAt(0) - '0';
c = seat.charAt(2);
if (Character.isLowerCase(c)) {
c = Character.toUpperCase(c);
}
checkAndBookSeat1(r, c, layout);
displaySeats(layout);
}
else {
if (!seat.equals("-1")) {
System.out.println("Invalid!");
System.out.println("Row value [1-5] and col [A-D]");
}
}
} while (!seat.equals("-1"));
```
### Explanation
This is a snippet of Java code, part of a program related to booking airline seats. Here's a breakdown of its functionality:
1. **User Input:**
- Uses `Scanner` to read user input for selecting a seat in the format of a row number followed by a seat letter.
2. **Main Loop:**
- Continuously prompts the user to enter seat details until they input `"-1"` to exit.
3. **Seat Validation:**
- Checks if the user input is three characters long.
- Extracts the row number and seat letter; converts lowercase letters to uppercase.
4. **Seat Processing:**
- Calls `checkAndBookSeat1` to verify and book the seat based on the user's input.
- Displays the current seating layout after booking.
5. **Error Handling:**
- If the input format is not valid and not equal to `"-1"`, it prints error messages guiding the user on valid input format.
This code snippet is likely part of a larger project for an airline seat booking system, aimed at teaching object-oriented programming concepts and user input handling in Java.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fafd3eb79-3297-4398-8445-49c2030e1595%2F63e2936c-554e-442a-82b7-3379650eeef1%2F5fjyqsi_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Below is the transcription of the code from the image, suitable for an educational website:
```java
// Scanner class for user input
Scanner sc = new Scanner(System.in);
// String seat = "";
System.out.println("Enter a row and a seat, please: ");
String seat = sc.nextLine();
int r = 0;
char c = '';
do {
seat = getUserInput(sc);
if (seat.length() == 3) {
r = seat.charAt(0) - '0';
c = seat.charAt(2);
if (Character.isLowerCase(c)) {
c = Character.toUpperCase(c);
}
checkAndBookSeat1(r, c, layout);
displaySeats(layout);
}
else {
if (!seat.equals("-1")) {
System.out.println("Invalid!");
System.out.println("Row value [1-5] and col [A-D]");
}
}
} while (!seat.equals("-1"));
```
### Explanation
This is a snippet of Java code, part of a program related to booking airline seats. Here's a breakdown of its functionality:
1. **User Input:**
- Uses `Scanner` to read user input for selecting a seat in the format of a row number followed by a seat letter.
2. **Main Loop:**
- Continuously prompts the user to enter seat details until they input `"-1"` to exit.
3. **Seat Validation:**
- Checks if the user input is three characters long.
- Extracts the row number and seat letter; converts lowercase letters to uppercase.
4. **Seat Processing:**
- Calls `checkAndBookSeat1` to verify and book the seat based on the user's input.
- Displays the current seating layout after booking.
5. **Error Handling:**
- If the input format is not valid and not equal to `"-1"`, it prints error messages guiding the user on valid input format.
This code snippet is likely part of a larger project for an airline seat booking system, aimed at teaching object-oriented programming concepts and user input handling in Java.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
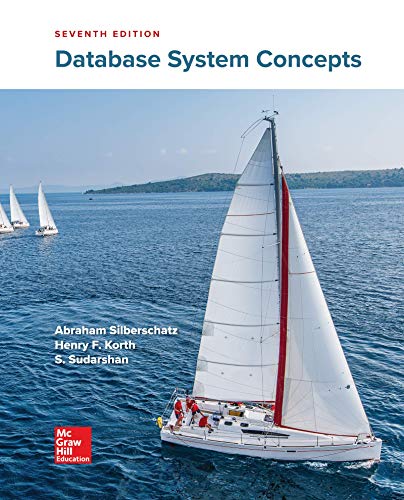
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
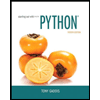
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
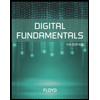
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
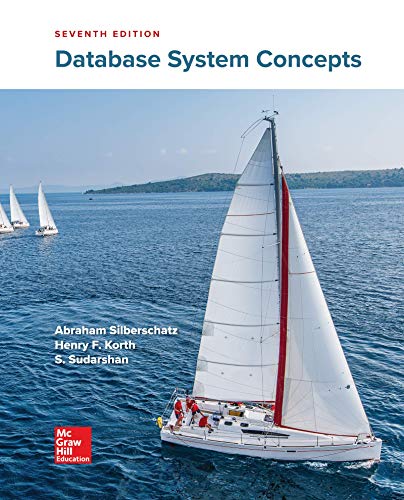
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
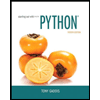
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
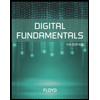
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
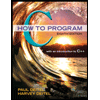
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
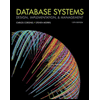
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
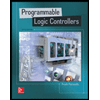
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education