Build an application that will generate a random number and then have the user guess it. hints below : First line of your program: import java.util.Scanner; To use math symbols: T: Math.Pl e" : Math.pow(Math.E,n) To read input from the keyboard: Scanner userlnput = new Scanner(System.in); x = userlnput.nextInt(); If you can't seem to read the next int, try adding this after the nextInt: (there may be a “\n" left on the input buffer) x=userlnput.nextLine(); To write to the console: System.out.printIn(x); System.out.println(“something to print");
Build an application that will generate a random number and then have the user guess it. hints below : First line of your program: import java.util.Scanner; To use math symbols: T: Math.Pl e" : Math.pow(Math.E,n) To read input from the keyboard: Scanner userlnput = new Scanner(System.in); x = userlnput.nextInt(); If you can't seem to read the next int, try adding this after the nextInt: (there may be a “\n" left on the input buffer) x=userlnput.nextLine(); To write to the console: System.out.printIn(x); System.out.println(“something to print");
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:### How to Build a Number Guessing Application
In this guide, we will provide you with the steps to create a simple Java application that generates a random number for the user to guess.
#### Step-by-Step Instructions
Below are some hints that will help you build this application.
### First line of your program
Start with importing the necessary utility for reading user input.
```java
import java.util.Scanner;
```
### To use math symbols
You may need to use some mathematical constants and functions. Here’s how:
- **π (Pi) Constant:**
```java
Math.PI
```
- **e^n (Exponential Function):**
```java
Math.pow(Math.E, n)
```
### To read input from the keyboard
To capture user input, use the `Scanner` class.
```java
Scanner userInput = new Scanner(System.in);
```
To get the next integer input from the user, write:
```java
int x = userInput.nextInt();
```
If you encounter issues reading the next integer, it may be due to a newline character left in the input buffer. You can fix this by adding:
```java
x = userInput.nextLine();
```
### To write output to the console
To display output on the console, use the `System.out.println()` method.
```java
System.out.println(x);
System.out.println("something to print");
```
By following these instructions, you will be able to create a functional number guessing game in Java. Don't forget to handle user guesses and provide feedback on whether the guess is too high, too low, or correct!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
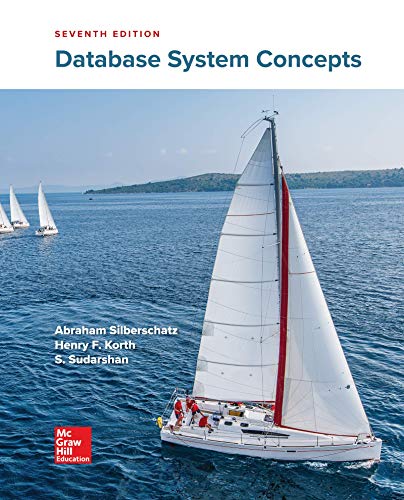
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
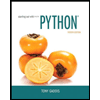
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
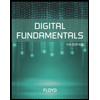
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
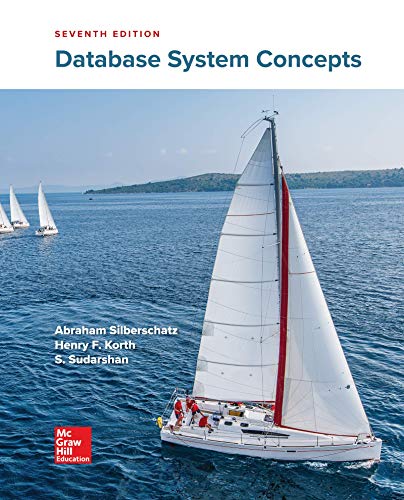
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
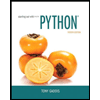
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
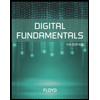
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
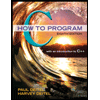
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
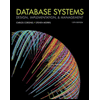
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
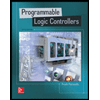
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education