(User Guessing Game) Scanner sc=new int num, guess, tries = 0; int max=100,min=1; num=(int) (Math.floor(Math.random()*(max-min+1)+min)); System.out.println("Guess My Number Game"); System.out.println("'-- --"); do { System.out.println("Enter a guess between 1 and 100: "); while(true){ try{ Scanner(System.in); guess-Integer.parseInt(sc.next()); if(guess>0 && guess<=100) break; else System.out.println("Please enter a number in the range 1-100"); } catch(NumberFormatException ex) System.out.println("Please enter a number"); { } tries++; if (guess > num){ System.out.println("Too high!"); } else if (guess < num){ System.out.println("Too low!"); } else System.out.println("\nCorrect! You got it in "+ tries+ "guesses!"); } while (guess != num); }
(User Guessing Game) Scanner sc=new int num, guess, tries = 0; int max=100,min=1; num=(int) (Math.floor(Math.random()*(max-min+1)+min)); System.out.println("Guess My Number Game"); System.out.println("'-- --"); do { System.out.println("Enter a guess between 1 and 100: "); while(true){ try{ Scanner(System.in); guess-Integer.parseInt(sc.next()); if(guess>0 && guess<=100) break; else System.out.println("Please enter a number in the range 1-100"); } catch(NumberFormatException ex) System.out.println("Please enter a number"); { } tries++; if (guess > num){ System.out.println("Too high!"); } else if (guess < num){ System.out.println("Too low!"); } else System.out.println("\nCorrect! You got it in "+ tries+ "guesses!"); } while (guess != num); }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Use Java please
How can I implement a console selection menu that prompts a user to select between playing (7 guesses) or (User guesses)? My code for 7 guesses and User guesses is in the photos (I don't need help with those, just the console selection menu).

Transcribed Image Text:(User Guessing Game)
Scanner sc-new
int num, guess, tries = 0;
int max=100,min=1;
num=(int) (Math.floor(Math.random()*(max-min+1)+min));
System.out.println("Guess My Number Game");
System.out.println("----
-");
do
{
System.out.println("Enter a guess between 1 and 100: ");
while(true){
try{
Scanner(System.in);
guess-Integer.parseInt(sc.next());
if(guess>0 && guess<=100)
break;
else System.out.println("Please enter a number in the range 1-100");
}
catch (NumberFormatException ex)
{
System.out.println("Please enter a number");
}
}
tries++;
if (guess > num){
System.out.println("Too high!");
}
else if (guess < num){
System.out.println("Too low!");
}
else
System.out.println("\nCorrect! You got it in "+ tries+ " guesses!");
} while (guess != num);
}

Transcribed Image Text:(7 Guesses)
Scanner sc=new Scanner(System.in);
int num, guess, tries = 0;
int max=100,min=1;
System.out.println("Enter a number between 1 and 100 you want computer to guess:");
while(true){
try
{
}
catch (NumberFormatException ex)
{
System.out.println("Please enter a number");
}
}
System.out.println("Guess My Number Game");
System.out.println("---
--");
num=Integer.parseInt( sc.next());
if(num>0 && num<=100)
break;
else System.out.println("Please enter a number in the range 1-100");
do
{
guess-(min+max)/2;
//(int)(Math.floor(Math.random()*(max-min+1)+min));
System.out.println("Computer guessed "+guess);
tries++;
if (guess > num){
System.out.println("Too high!");
max-guess;
}
else if (guess < num){
System.out.println("Too low!");
min-guess+1;
}
}
else
System.out.println("\nCorrect! You got it in "+ tries+ " guesses!");
} while (guess != num);
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
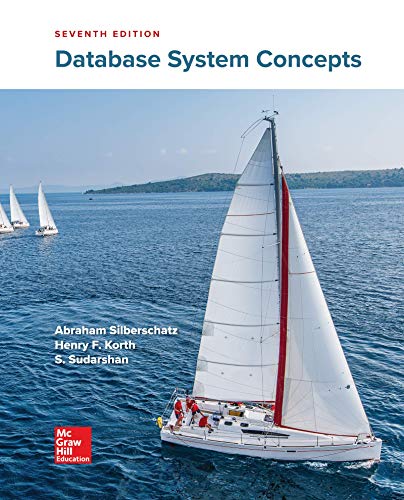
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
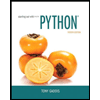
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
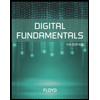
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
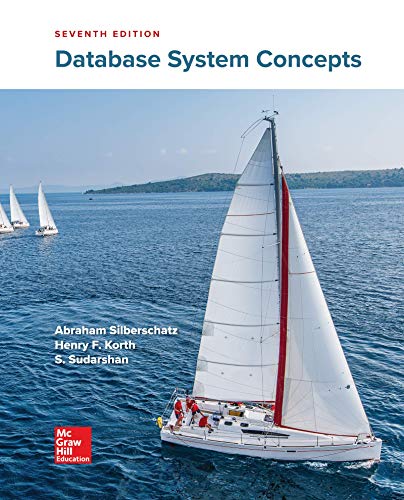
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
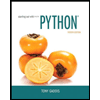
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
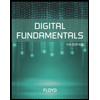
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
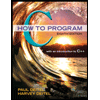
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
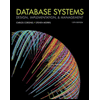
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
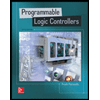
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education