In this assignment you will write a program that shows the valid moves of chess pieces. Your program will draw a board with 64 squares using the traditional layout, next ask the user to choose a move, and then, depending on the user's choice, redraw the board with the selected chess piece and its valid moves. Please see the examples of valid moves of chess pieces and the traditional chess board layout below:
In this assignment you will write a program that shows the valid moves of chess pieces. Your program will draw a board with 64 squares using the traditional layout, next ask the user to choose a move, and then, depending on the user's choice, redraw the board with the selected chess piece and its valid moves. Please see the examples of valid moves of chess pieces and the traditional chess board layout below:
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In this assignment you will write a program that shows the valid moves of chess pieces. Your program will draw a board with 64 squares using the traditional layout, next ask the user to choose a move, and then, depending on the user's choice, redraw the board with the selected chess piece and its valid moves. Please see the examples of valid moves of chess pieces and the traditional chess board layout below:

Transcribed Image Text:## Chess Programming - Move Representation and Board Display
### Introduction to Chess Programming
At the beginning, your program should draw an empty chess board and prompt the user to enter a move:
```
Welcome to the Chess Game!
a b c d e f g h
8 +--+--+--+--+--+--+--+--+ 8
7 +--+--+--+--+--+--+--+--+ 7
6 +--+--+--+--+--+--+--+--+ 6
5 +--+--+--+--+--+--+--+--+ 5
4 +--+--+--+--+--+--+--+--+ 4
3 +--+--+--+--+--+--+--+--+ 3
2 +--+--+--+--+--+--+--+--+ 2
1 +--+--+--+--+--+--+--+--+ 1
a b c d e f g h
Enter a chess piece and its position or type X to exit:
```
### Move Representation
A move is represented as a three-letter string where the first letter corresponds to a chess piece initial:
- `K` for king
- `Q` for queen
- `B` for bishop
- `N` for knight
- `R` for rook
**Note**: Pawns do not have initials, and you will not be tested on their implementation.
The next letter in the move is the position on the board that corresponds to one of eight columns (called files). The columns (files) are labeled as `a, b, c, d, e, f, g, h`.
The third symbol is a digit that corresponds to one of eight rows (called ranks). The rows (ranks) are labeled as `1, 2, 3, 4, 5, 6, 7, 8`. For example, if the user enters `Re6`, your program should generate the following output where `R` is the initial for a rook, and all possible moves of the rook are marked by `x`:
```
a b c d e f g h
8 +--+--+--+--+
![### Chess Programming Assignment
#### User Input
If the user does not enter a valid move that is included in all possible combinations of chess piece initials (K, Q, B, N, R), files (a, b, c, d, e, f, g, h), and ranks (1, 2, 3, 4, 5, 6, 7, 8), then the program should reprint the prompt:
```
Enter a chess piece and its position or type X to exit:
```
If the user enters X (or x), then the program should print 'Goodbye!' and terminate:
```
Goodbye!
```
##### Note:
The user can use either upper or lowercase letters, for example X or x should result in the termination of the program, Ke3, KE3, kE3, or ke3 means the same move.
#### Programming Approaches
In this assignment, you need to create a class `Board` and a class for each chess piece: King, Queen, Bishop, Knight, and Rook. They all should be located in a module called `chess.py`. All chess piece classes should be derived from the superclass `Chess_Piece` given to you.
Your class `Board` should have four methods: `init`, `empty`, `set`, and `draw`. Three of the methods are already implemented for you. You only need to implement the method `draw()` that should print the board layout and label squares accordingly to the current chess piece positioned on the board. You can type or copy and paste the following code into your file `chess.py`.
The given class `Board` has only one attribute that is also called `board`, and it is implemented as a dictionary. The method `empty()` generates an empty board (a dictionary with keys corresponding to chess board squares, and their values are white spaces ' '). The method `set()` updates the label of a given square by assigning the dictionary key value to its new value.
```python
class Board:
def __init__(self):
self.board = {}
self.empty()
def empty(self):
for col in 'abcdefgh':
for row in '12345678':
self.board[col+row] = ' '
def set(self, pos, piece): # pos is a square label (a1, a2, ..., h8)
if pos in self.board.keys():](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdd3d3176-2cf4-4554-91a8-a12c09f2d35b%2F6884ab95-ede7-4f15-9f3d-0f298d7faa18%2Fxh45yvt_processed.png&w=3840&q=75)
Transcribed Image Text:### Chess Programming Assignment
#### User Input
If the user does not enter a valid move that is included in all possible combinations of chess piece initials (K, Q, B, N, R), files (a, b, c, d, e, f, g, h), and ranks (1, 2, 3, 4, 5, 6, 7, 8), then the program should reprint the prompt:
```
Enter a chess piece and its position or type X to exit:
```
If the user enters X (or x), then the program should print 'Goodbye!' and terminate:
```
Goodbye!
```
##### Note:
The user can use either upper or lowercase letters, for example X or x should result in the termination of the program, Ke3, KE3, kE3, or ke3 means the same move.
#### Programming Approaches
In this assignment, you need to create a class `Board` and a class for each chess piece: King, Queen, Bishop, Knight, and Rook. They all should be located in a module called `chess.py`. All chess piece classes should be derived from the superclass `Chess_Piece` given to you.
Your class `Board` should have four methods: `init`, `empty`, `set`, and `draw`. Three of the methods are already implemented for you. You only need to implement the method `draw()` that should print the board layout and label squares accordingly to the current chess piece positioned on the board. You can type or copy and paste the following code into your file `chess.py`.
The given class `Board` has only one attribute that is also called `board`, and it is implemented as a dictionary. The method `empty()` generates an empty board (a dictionary with keys corresponding to chess board squares, and their values are white spaces ' '). The method `set()` updates the label of a given square by assigning the dictionary key value to its new value.
```python
class Board:
def __init__(self):
self.board = {}
self.empty()
def empty(self):
for col in 'abcdefgh':
for row in '12345678':
self.board[col+row] = ' '
def set(self, pos, piece): # pos is a square label (a1, a2, ..., h8)
if pos in self.board.keys():
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
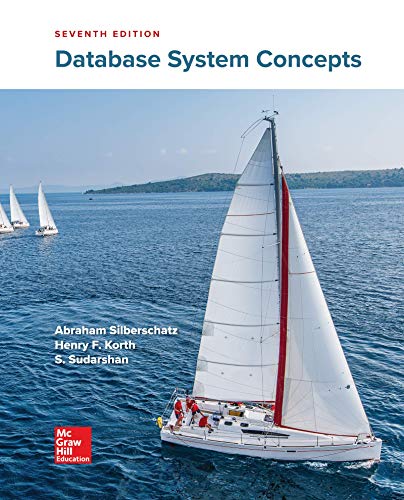
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
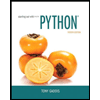
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
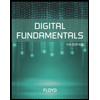
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
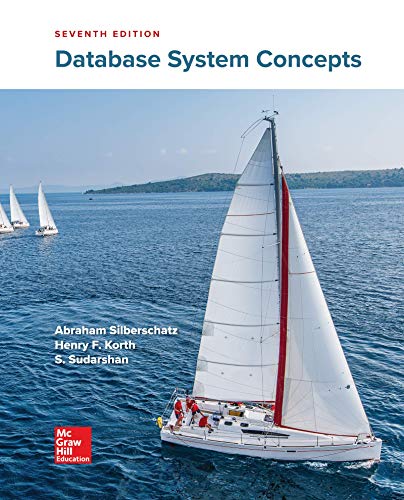
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
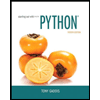
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
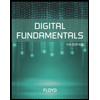
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
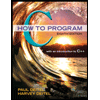
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
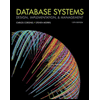
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
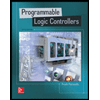
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education