Use Java please. At the beginning of the program, implement a selection menu that prompts the user to select between two options (the two options being "7 guesses" and "user guesses" shown in the two pictures below).
Use Java please. At the beginning of the program, implement a selection menu that prompts the user to select between two options (the two options being "7 guesses" and "user guesses" shown in the two pictures below).
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Use Java please.
At the beginning of the program, implement a selection menu that prompts the user to select between two options (the two options being "7 guesses" and "user guesses" shown in the two pictures below).

Transcribed Image Text:## Number Guessing Game Code Explanation
### Overview
The following Java code implements a simple number guessing game. The program prompts the user to select a number between 1 and 100. It then uses a binary search strategy to guess the number, displaying whether each guess is too high or too low until the correct number is identified.
### Code Breakdown
1. **Initialization:**
```java
Scanner sc = new Scanner(System.in);
int num, guess, tries = 0;
int max = 100, min = 1;
```
- **Scanner Creation:** A `Scanner` object is created to read user input.
- **Variables:** `num` to store the user's number, `guess` for the computer's guess, and `tries` to count the number of guesses. `max` and `min` set the range for guessing.
2. **User Input:**
```java
System.out.println("Enter a number between 1 and 100 you want computer to guess:");
while (true) {
num = Integer.parseInt(sc.next());
if (num > 0 && num <= 100)
break;
else
System.out.println("Please enter a number in the range 1-100");
}
```
- **Prompt User:** Asks the user to enter a number between 1 and 100.
- **Validation Loop:** Ensures the number is within the specified range. Continues prompting if the input is invalid.
3. **Guessing Logic:**
```java
System.out.println("Guess My Number Game");
System.out.println("-------------------");
do {
guess = (min + max) / 2;
System.out.println("Computer guessed " + guess);
tries++;
if (guess > num) {
System.out.println("Too high!");
max = guess;
} else if (guess < num) {
System.out.println("Too low!");
min = guess + 1;
} else {
System.out.println("\nCorrect! You got it in " + tries + " guesses!");
}
} while (guess != num);
```
- **Binary Search Approach:** The guess is calculated as the midpoint of the current `min` and `max`.
- **Guess Feedback:** Prints whether the guess is "Too high" or "Too low" and

Transcribed Image Text:# User Guessing Game
This Java program is designed as a simple number-guessing game. It challenges users to guess a randomly generated number between 1 and 100. The program provides feedback if the user's guess is too high, too low, or correct.
### Code Explanation:
- **Initialization:**
- A `Scanner` object `sc` is created for capturing user input.
- Variables `num`, `guess`, and `tries` are initialized, with `num` storing the randomly generated number, `guess` capturing user inputs, and `tries` counting the number of attempts made.
- **Random Number Generation:**
- The random number is generated using `Math.random()` and stored in `num`. The range is set between `min` (1) and `max` (100).
- **Game Loop:**
- The program starts by displaying "Guess My Number Game" and prompts the user to enter a guess between 1 and 100.
- A `do-while` loop ensures the game continues until the correct number is guessed.
- **Input Handling:**
- Inside a `while(true)` loop, user inputs are taken and parsed as integers.
- A `try-catch` block manages exceptions if the input is not a number.
- **Condition Checks:**
- If the guess is out of range (not between 1 and 100), the user is asked to enter a valid number.
- After every valid input, `tries` is incremented.
- **Feedback:**
- If the user’s guess is higher than the generated number, "Too high!" is printed.
- If the guess is lower, "Too low!" is printed.
- If the guess is correct, the program congratulates the user and displays the number of guesses made.
This structure provides a simple yet instructive example of loop control, input validation, and exception handling in Java.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
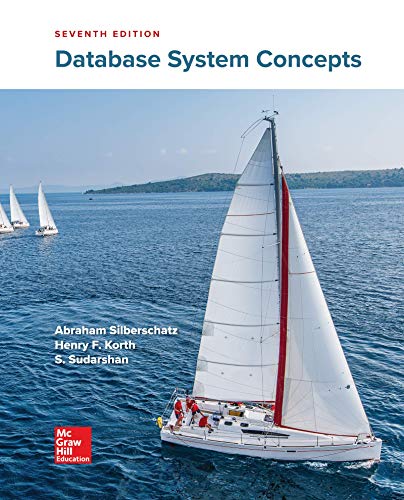
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
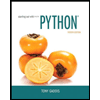
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
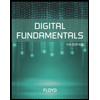
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
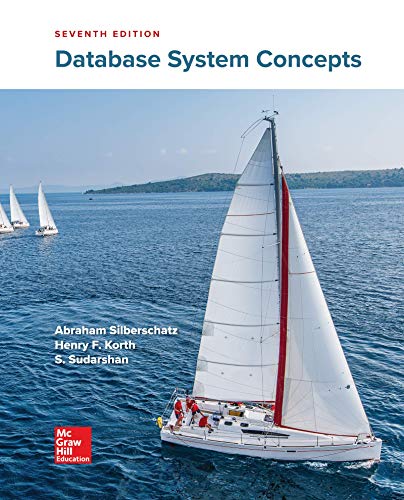
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
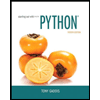
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
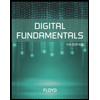
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
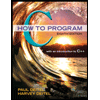
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
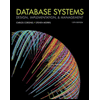
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
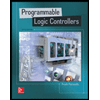
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education