Please you can complete this part of my code almost done, I could not get the output. Please help me to fix this and add part 4 and 5. Part 4 instructions (please see image attached): Implement the relationship between interface and the classes as shown in the diagram below. You must correctly implement appropriate methods in your classes based on abstraction and interface principles. Part 5 instructions: . Inside the main() method of the Watch class, do the following: a) Create a new instance of the “AnalogWatch” class using the overloaded constructor and assign it to the object reference of type “Watch”. b) Create a new instance of the “DigitalWatch” class using the overloaded constructor and assign it to the object reference of type “Watch”. c) Display the content of both the objects. d) Display the current time using the two objects created above respectively Code: public abstract class Watch { //Data Fields private String brand; private String material; private double price; //Default Constructor Watch(){ } //constructor Watch object with the specified objects:brand: String, material: String, price: double. Watch(String brand, String material, double price){ this.brand=brand; this.material=material; this.price=price; } //Setter & getters public void setBrand(String brand) { this.brand = brand; } public String getBrand() { return brand; } public void setMaterial(String material) { this.material = material; } public String getMaterial() { return material; } public void setPrice(double price) { this.price = price; } public double getPrice() { return price; } /*//Method Abstract public abstract boolean isWaterProof();*/ //toString @Override public String toString() { return "\nWatch: "+"\nBrand: "+ brand +"\nMaterial: "+ material + "\nPrice: "+ price; } // Please help me with this part too-->Part 5 (Still Missing) //////////////////Main Method inside Parent Class //////////////////////////////// public static void main(String[] args) { } } public class AnalogWatch extends Watch { //Data Fields private int numbOfHands; //Default Constructor AnalogWatch (){ } ///Constructor AnalogWathc object with specified brand, material, price, // numbOfHands. AnalogWatch (String brand, String material, double price, int numbOfHands){ super(brand, material, price); this.numbOfHands=numbOfHands; } //Setters & getters public void setNumbOfHands(int numbOfHands) { this.numbOfHands = numbOfHands; } public int getNumbOfHands() { return numbOfHands; } //toString @Override public String toString(){ return "\nWatch: "+"\nBrand: "+ super.getBrand() +"\nMaterial: "+ super.getMaterial() + "\nPrice: "+ super.getPrice()+"\nNumOfHands: "+ getNumbOfHands(); } } public class DigitalWatch extends Watch { //Data Fields private int hourFormat; //Default Constructor DigitalWatch (){ super(); } //Constructor DigitalWatch object with specified specified brand, material, price, //hourFormat. DigitalWatch (String brand, String material, double price, int hourFormat){ super(brand, material, price); this.hourFormat=hourFormat; } //Setters &Getters public void setHourFormat(int hourFormat) { this.hourFormat = hourFormat; } public int getHourFormat() { return hourFormat; } //ToString @Override public String toString() { return "\nDigitalWatch: "+"\nBrand: "+ super.getBrand() +"\nMaterial: "+ super.getMaterial() + "\nPrice: "+ super.getPrice()+"\nHourFormat: "+ getHourFormat(); } } --->This is the interface name Timer Code: import java.time.LocalDateTime; import java.time.format.DateTimeFormatter; public interface Timer { public String howtoChangeWatchTime(); public default String getCurrentTime() { LocalDateTime now= LocalDateTime.now(); DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss"); return now.format(formatter); } } UML Diagram only for reference: Watch -brand: String -material: String -price: double +Watch() +Watch(brand: String, material: String, price: double) +setBrand(brand: String): void +getBrand(): String +setMaterial(material: String): void +getMaterial(): String +setPrice(brand: double): void +getPrice(): double +isWaterProof(): boolean +toString():String -->SecondUML: Child Class AnalogWatch -numOfHands: int +AnalogWatch() +AnalogWatch(brand: String, material: String, price: double, numOfHands: int) +setNumOfHands(numOfHands: int): void +getNumOfHands(): int +toString():String -->Third UML only for reference: Child class DigitalWatch -hourFormat: int +DigitalWatch() +DigitalWatch(brand: String, material: String, price: double, hourFormat: int) +sethourFormat(hourFormat: int): void +gethourFormat(): int +toString(): String
Please you can complete this part of my code almost done, I could not get the output. Please help me to fix this and add part 4 and 5.
Part 4 instructions (please see image attached): Implement the relationship between interface and the classes as shown in the diagram below. You must correctly implement appropriate methods in your classes
based on abstraction and interface principles.
Part 5 instructions: . Inside the main() method of the Watch class, do the following:
a) Create a new instance of the “AnalogWatch” class using the overloaded constructor
and assign it to the object reference of type “Watch”.
b) Create a new instance of the “DigitalWatch” class using the overloaded constructor
and assign it to the object reference of type “Watch”.
c) Display the content of both the objects.
d) Display the current time using the two objects created above respectively
Code:
public abstract class Watch {
//Data Fields
private String brand;
private String material;
private double price;
//Default Constructor
Watch(){
}
//constructor Watch object with the specified objects:brand: String, material: String, price: double.
Watch(String brand, String material, double price){
this.brand=brand;
this.material=material;
this.price=price;
}
//Setter & getters
public void setBrand(String brand) {
this.brand = brand;
}
public String getBrand() {
return brand;
}
public void setMaterial(String material) {
this.material = material;
}
public String getMaterial() {
return material;
}
public void setPrice(double price) {
this.price = price;
}
public double getPrice() {
return price;
}
/*//Method Abstract
public abstract boolean isWaterProof();*/
//toString
@Override
public String toString() {
return "\nWatch: "+"\nBrand: "+ brand +"\nMaterial: "+ material
+ "\nPrice: "+ price;
}
// Please help me with this part too-->Part 5 (Still Missing)
//////////////////Main Method inside Parent Class ////////////////////////////////
public static void main(String[] args) {
}
}
public class AnalogWatch extends Watch {
//Data Fields
private int numbOfHands;
//Default Constructor
AnalogWatch (){
}
///Constructor AnalogWathc object with specified brand, material, price,
// numbOfHands.
AnalogWatch (String brand, String material, double price, int numbOfHands){
super(brand, material, price);
this.numbOfHands=numbOfHands;
}
//Setters & getters
public void setNumbOfHands(int numbOfHands) {
this.numbOfHands = numbOfHands;
}
public int getNumbOfHands() {
return numbOfHands;
}
//toString
@Override
public String toString(){
return "\nWatch: "+"\nBrand: "+ super.getBrand() +"\nMaterial: "+ super.getMaterial()
+ "\nPrice: "+ super.getPrice()+"\nNumOfHands: "+ getNumbOfHands();
}
}
public class DigitalWatch extends Watch {
//Data Fields
private int hourFormat;
//Default Constructor
DigitalWatch (){
super();
}
//Constructor DigitalWatch object with specified specified brand, material, price,
//hourFormat.
DigitalWatch (String brand, String material, double price, int hourFormat){
super(brand, material, price);
this.hourFormat=hourFormat;
}
//Setters &Getters
public void setHourFormat(int hourFormat) {
this.hourFormat = hourFormat;
}
public int getHourFormat() {
return hourFormat;
}
//ToString
@Override
public String toString() {
return "\nDigitalWatch: "+"\nBrand: "+ super.getBrand() +"\nMaterial: "+ super.getMaterial()
+ "\nPrice: "+ super.getPrice()+"\nHourFormat: "+ getHourFormat();
}
}
--->This is the interface name Timer Code:
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public interface Timer {
public String howtoChangeWatchTime();
public default String getCurrentTime() {
LocalDateTime now= LocalDateTime.now();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
return now.format(formatter);
}
}
UML Diagram only for reference:
Watch
-brand: String
-material: String
-price: double
+Watch()
+Watch(brand: String, material: String, price: double)
+setBrand(brand: String): void
+getBrand(): String
+setMaterial(material: String): void
+getMaterial(): String
+setPrice(brand: double): void
+getPrice(): double
+isWaterProof(): boolean
+toString():String
-->SecondUML: Child Class
AnalogWatch
-numOfHands: int
+AnalogWatch()
+AnalogWatch(brand: String, material: String, price: double, numOfHands: int)
+setNumOfHands(numOfHands: int): void
+getNumOfHands(): int
+toString():String
-->Third UML only for reference: Child class
DigitalWatch
-hourFormat: int
+DigitalWatch()
+DigitalWatch(brand: String, material: String, price: double, hourFormat: int)
+sethourFormat(hourFormat: int): void
+gethourFormat(): int
+toString(): String


Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 1 images

This method does not work, please you can help me to add to this code Watch.java and how to add to the other codes AnalogWatch. java and DigitalWatch.java
//Method Abstract
public abstract boolean isWaterProof();
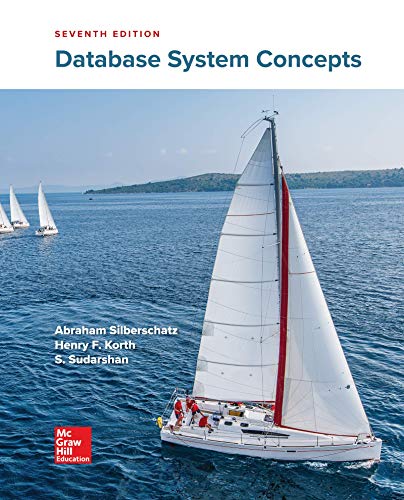
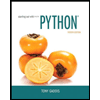
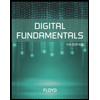
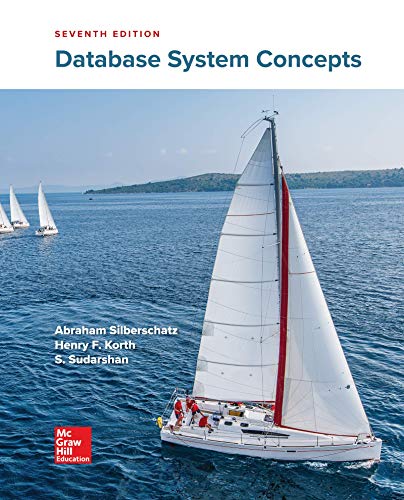
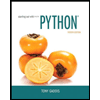
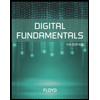
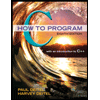
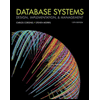
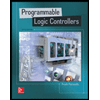