I would like you to create a program that keeps track of the inventory (by make - e.g., Toyota) of cars on a used car lot. you will have to create two classes. The first is a simple Car class that consists of an inventory, make, and model. The second class you need to create for this challenge is the Lot. A Lot can contain many cars. Part 1 Write the Car constructor, get methods, and toString method. Part 2 Write the Car's increase and decrease inventory methods. Part 3 Edit the Car so it implements Comparable and compares Car instances based on their inventory in descending order.
I would like you to create a program that keeps track of the inventory (by make - e.g., Toyota) of cars on a used car lot.
you will have to create two classes. The first is a simple Car class that consists of an inventory, make, and model.
The second class you need to create for this challenge is the Lot. A Lot can contain many cars.
- Part 1 Write the Car constructor, get methods, and toString method.
- Part 2 Write the Car's increase and decrease inventory methods.
- Part 3 Edit the Car so it implements Comparable and compares Car instances based on their inventory in descending order.
- Part 4 Write the getCars and getCarsSorted methods of the Lot class.
- Part 5 Write the getInventory method of the Lot class.
- Part 6 Use a try/catch block in the Car's constructor so that it sets the inventory to 0 if the inventory passed to the constructor is not a valid integer.
this is the output that should be produced by main:
Make: [Toyota Camry (10), Toyota Prius (4), Toyota Rav4 (16)]
[Hyundai Kona Electric (4)]
[Honda Civic (8), Honda CRV (3)]
[]
Make sorted by inventory:
[Toyota Rav4 (16), Toyota Camry (10), Toyota Prius (4)]
[Hyundai Kona Electric (4)]
[Honda Civic (8), Honda CRV (3)]
[]
class Main
{
public static void main(String[] args)
{
Car c1 = new Car("10", "Toyota", "Camry");
Car c2 = new Car("4", "Toyota", "Prius");
Car c3 = new Car("16", "Toyota", "Rav4");
Car c4 = new Car("4", "Hyundai", "Kona Electric");
Car c5 = new Car("8", "Honda", "Civic");
Car c6 = new Car("3", "Honda", "CRV");
Lot lot1 = new Lot();
lot1.addCar(c1);
lot1.addCar(c2);
lot1.addCar(c3);
lot1.addCar(c4);
lot1.addCar(c5);
lot1.addCar(c6);
System.out.println("Make:");
System.out.println(lot1.getCars("Toyota"));
System.out.println(lot1.getCars("Hyundai"));
System.out.println(lot1.getCars("Honda"));
System.out.println(lot1.getCars("Tesla"));
System.out.println();
System.out.println("Make sorted by inventory:");
System.out.println(lot1.getCarsSorted("Toyota"));
System.out.println(lot1.getCarsSorted("Hyundai"));
System.out.println(lot1.getCarsSorted("Honda"));
System.out.println(lot1.getCarsSorted("Tesla"));
System.out.println();
System.out.println("Inventory by make:");
System.out.println(lot1.getInventory("Toyota") + " Toyotas");
System.out.println(lot1.getInventory("Hyundai") + " Hyundais");
System.out.println(lot1.getInventory("Honda") + " Hondas");
System.out.println(lot1.getInventory("Tesla") + " Teslas");
System.out.println();
}
}
import java.util.*;
public class Lot
{
// Given a make, this map should return a list of
// all of the cars on the lot of that make.
private HashMap<String, ArrayList<Car>> m_cars;
// Creates an empty lot.
public Lot()
{
m_cars = new HashMap<String, ArrayList<Car>>();
}
// Adds a car to the lot.
public void addCar(Car c)
{
if (m_cars.get(c.getMake()) == null)
m_cars.put(c.getMake(), new ArrayList<Car>());
m_cars.get(c.getMake()).add(c);
}
// Return a list of cars on the lot of
// the specified make.
public ArrayList<Car> getCars(String make)
{
// TODO: Part 4
}
// Return a list of cars on the lot of
// the specified make. The list should be sorted
// by inventory in descending order.
public ArrayList<Car> getCarsSorted(String make)
{
// TODO: Part 4
}
// Return the total inventory of cars on the lot
// of the specified make.
public int getInventory(String make)
{
// TODO: Part 5
}
}
import java.util.*;
public class Car implements Comparable<Car>
{
private int m_inventory;
private String m_make;
private String m_model;
public Car(String inventory, String make, String model)
{
m_inventory = Integer.parseInt(inventory);
m_make = make;
m_model = model;
}
public int getInventory()
{
// TODO: Part 1
}
public void increaseInventory()
{
// TODO Part 2
}
// Decreases the inventory by one.
// Don't let the inventory get less than 0.
public void decreaseInventory()
{
// TODO: Part 2
}
// Returns the make.
public String getMake()
{
// TODO: Part 1
}
// Returns the model.
public String getModel()
{
// TODO: Part 1
}
@Override
// Returns a string in the following format:
// make model (inventory)
// For example: Toyota Prius (5)
public String toString()
{
// TODO: Part 1
}
@Override
// Compares two cars based on their inventory (descending order).
public int compareTo(Car other)
{
// TODO: Part 3
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

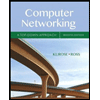
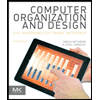
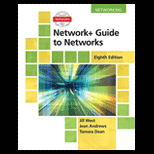
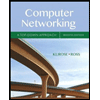
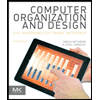
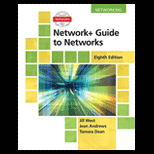
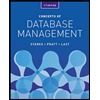
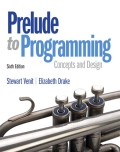
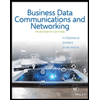