Hi guys, I need help correcting my code. It's supposed to create a slideshow however doesn't seem to be working. Any advice or tweaks? Would be appreciated. import java.awt.BorderLayout; import java.awt.CardLayout; import java.awt.EventQueue; import java.awt.FlowLayout; import java.awt.HeadlessException; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel; import java.awt.Color; public class SlideShow extends JFrame { //Declare Variables private JPanel slidePane; private JPanel textPane; private JPanel buttonPane; private CardLayout card; private CardLayout cardText; private JButton btnPrev; private JButton btnNext; private JLabel lblSlide; private JLabel lblTextArea; /** * Create the application. */ public SlideShow() throws HeadlessException { initComponent(); } /** * Initialize the contents of the frame. */ private void initComponent() { //Initialize variables to empty objects card = new CardLayout(); cardText = new CardLayout(); slidePane = new JPanel(); textPane = new JPanel(); textPane.setBackground(Color.BLUE); textPane.setBounds(5, 470, 790, 50); textPane.setVisible(true); buttonPane = new JPanel(); btnPrev = new JButton(); btnNext = new JButton(); lblSlide = new JLabel(); lblTextArea = new JLabel(); //Setup frame attributes setSize(800, 600); setLocationRelativeTo(null); setTitle("Top 5 Destinations SlideShow"); getContentPane().setLayout(new BorderLayout(10, 50)); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //Setting the layouts for the panels slidePane.setLayout(card); textPane.setLayout(cardText); //logic to add each of the slides and text for (int i = 1; i <= 5; i++) { lblSlide = new JLabel(); lblTextArea = new JLabel(); lblSlide.setText(getResizeIcon(i)); lblTextArea.setText(getTextDescription(i)); slidePane.add(lblSlide, "card" + i); textPane.add(lblTextArea, "cardText" + i); } getContentPane().add(slidePane, BorderLayout.CENTER); getContentPane().add(textPane, BorderLayout.SOUTH); buttonPane.setLayout(new FlowLayout(FlowLayout.CENTER, 20, 10)); btnPrev.setText("Previous"); btnPrev.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { goPrevious(); } }); buttonPane.add(btnPrev); btnNext.setText("Next"); btnNext.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { goNext(); } }); buttonPane.add(btnNext); getContentPane().add(buttonPane, BorderLayout.SOUTH); } /** * Previous Button Functionality */ private void goPrevious() { card.previous(slidePane); cardText.previous(textPane); } /** * Next Button Functionality */ private void goNext() { card.next(slidePane); cardText.next(textPane); } /** * Method to get the images */ private String getResizeIcon(int i) { String image = ""; if (i==1){ image = ""; } else if (i==2){ image = ""; } else if (i==3){ image = ""; } else if (i==4){ image = ""; } else if (i==5){ image = ""; } return image; } /** * Method to get the text values */ private String getTextDescription(int i) { String text = ""; if (i==1){ text = "#1 Agrivilla i pini - Italy A perfect detox retret in the rolling hills of Tuscany," + "surronded by olive groves and vineyards."; } else if (i==2){ text = "#2 Ananda in the Himalayas - India Famous for meditation and yoga!"; } else if (i==3){ text = "#3 Cala Luna Hotel and Villa - Costa Rica What better way to detox then a tropical paradise?" + ""; } else if (i==4){ text = "#4 Sedona Resort at Bell Rock - United States Take your mind out of the stress with" + "these breathingtaking canyons and spa!"; } else if (i==5){ text = "#5 Spa Eastman - Canada Wind down in these beautiful Nordic spas, famous" + "for its stress relief and eliminating toxins!"; } return text; } /** * Launch the application. */ public static void main(String[] args) { EventQueue.invokeLater(new Runnable() { @Override public void run() { SlideShow ss = new SlideShow(); ss.setVisible(true); } }); } }
Hi guys, I need help correcting my code. It's supposed to create a slideshow however doesn't seem to be working. Any advice or tweaks? Would be appreciated. import java.awt.BorderLayout; import java.awt.CardLayout; import java.awt.EventQueue; import java.awt.FlowLayout; import java.awt.HeadlessException; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel; import java.awt.Color; public class SlideShow extends JFrame { //Declare Variables private JPanel slidePane; private JPanel textPane; private JPanel buttonPane; private CardLayout card; private CardLayout cardText; private JButton btnPrev; private JButton btnNext; private JLabel lblSlide; private JLabel lblTextArea; /** * Create the application. */ public SlideShow() throws HeadlessException { initComponent(); } /** * Initialize the contents of the frame. */ private void initComponent() { //Initialize variables to empty objects card = new CardLayout(); cardText = new CardLayout(); slidePane = new JPanel(); textPane = new JPanel(); textPane.setBackground(Color.BLUE); textPane.setBounds(5, 470, 790, 50); textPane.setVisible(true); buttonPane = new JPanel(); btnPrev = new JButton(); btnNext = new JButton(); lblSlide = new JLabel(); lblTextArea = new JLabel(); //Setup frame attributes setSize(800, 600); setLocationRelativeTo(null); setTitle("Top 5 Destinations SlideShow"); getContentPane().setLayout(new BorderLayout(10, 50)); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //Setting the layouts for the panels slidePane.setLayout(card); textPane.setLayout(cardText); //logic to add each of the slides and text for (int i = 1; i <= 5; i++) { lblSlide = new JLabel(); lblTextArea = new JLabel(); lblSlide.setText(getResizeIcon(i)); lblTextArea.setText(getTextDescription(i)); slidePane.add(lblSlide, "card" + i); textPane.add(lblTextArea, "cardText" + i); } getContentPane().add(slidePane, BorderLayout.CENTER); getContentPane().add(textPane, BorderLayout.SOUTH); buttonPane.setLayout(new FlowLayout(FlowLayout.CENTER, 20, 10)); btnPrev.setText("Previous"); btnPrev.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { goPrevious(); } }); buttonPane.add(btnPrev); btnNext.setText("Next"); btnNext.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { goNext(); } }); buttonPane.add(btnNext); getContentPane().add(buttonPane, BorderLayout.SOUTH); } /** * Previous Button Functionality */ private void goPrevious() { card.previous(slidePane); cardText.previous(textPane); } /** * Next Button Functionality */ private void goNext() { card.next(slidePane); cardText.next(textPane); } /** * Method to get the images */ private String getResizeIcon(int i) { String image = ""; if (i==1){ image = ""; } else if (i==2){ image = ""; } else if (i==3){ image = ""; } else if (i==4){ image = ""; } else if (i==5){ image = ""; } return image; } /** * Method to get the text values */ private String getTextDescription(int i) { String text = ""; if (i==1){ text = "#1 Agrivilla i pini - Italy A perfect detox retret in the rolling hills of Tuscany," + "surronded by olive groves and vineyards."; } else if (i==2){ text = "#2 Ananda in the Himalayas - India Famous for meditation and yoga!"; } else if (i==3){ text = "#3 Cala Luna Hotel and Villa - Costa Rica What better way to detox then a tropical paradise?" + ""; } else if (i==4){ text = "#4 Sedona Resort at Bell Rock - United States Take your mind out of the stress with" + "these breathingtaking canyons and spa!"; } else if (i==5){ text = "#5 Spa Eastman - Canada Wind down in these beautiful Nordic spas, famous" + "for its stress relief and eliminating toxins!"; } return text; } /** * Launch the application. */ public static void main(String[] args) { EventQueue.invokeLater(new Runnable() { @Override public void run() { SlideShow ss = new SlideShow(); ss.setVisible(true); } }); } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Hi guys, I need help correcting my code. It's supposed to create a slideshow however doesn't seem to be working. Any advice or tweaks? Would be appreciated.
import java.awt.BorderLayout;
import java.awt.CardLayout;
import java.awt.EventQueue;
import java.awt.FlowLayout;
import java.awt.HeadlessException;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import java.awt.Color;
public class SlideShow extends JFrame {
//Declare Variables
private JPanel slidePane;
private JPanel textPane;
private JPanel buttonPane;
private CardLayout card;
private CardLayout cardText;
private JButton btnPrev;
private JButton btnNext;
private JLabel lblSlide;
private JLabel lblTextArea;
/**
* Create the application.
*/
public SlideShow() throws HeadlessException {
initComponent();
}
/**
* Initialize the contents of the frame.
*/
private void initComponent() {
//Initialize variables to empty objects
card = new CardLayout();
cardText = new CardLayout();
slidePane = new JPanel();
textPane = new JPanel();
textPane.setBackground(Color.BLUE);
textPane.setBounds(5, 470, 790, 50);
textPane.setVisible(true);
buttonPane = new JPanel();
btnPrev = new JButton();
btnNext = new JButton();
lblSlide = new JLabel();
lblTextArea = new JLabel();
//Setup frame attributes
setSize(800, 600);
setLocationRelativeTo(null);
setTitle("Top 5 Destinations SlideShow");
getContentPane().setLayout(new BorderLayout(10, 50));
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
//Setting the layouts for the panels
slidePane.setLayout(card);
textPane.setLayout(cardText);
//logic to add each of the slides and text
for (int i = 1; i <= 5; i++) {
lblSlide = new JLabel();
lblTextArea = new JLabel();
lblSlide.setText(getResizeIcon(i));
lblTextArea.setText(getTextDescription(i));
slidePane.add(lblSlide, "card" + i);
textPane.add(lblTextArea, "cardText" + i);
}
getContentPane().add(slidePane, BorderLayout.CENTER);
getContentPane().add(textPane, BorderLayout.SOUTH);
buttonPane.setLayout(new FlowLayout(FlowLayout.CENTER, 20, 10));
btnPrev.setText("Previous");
btnPrev.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
goPrevious();
}
});
buttonPane.add(btnPrev);
btnNext.setText("Next");
btnNext.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
goNext();
}
});
buttonPane.add(btnNext);
getContentPane().add(buttonPane, BorderLayout.SOUTH);
}
/**
* Previous Button Functionality
*/
private void goPrevious() {
card.previous(slidePane);
cardText.previous(textPane);
}
/**
* Next Button Functionality
*/
private void goNext() {
card.next(slidePane);
cardText.next(textPane);
}
/**
* Method to get the images
*/
private String getResizeIcon(int i) {
String image = "";
if (i==1){
image = "<html><body><img width= '800' height='500' src='" + getClass().getResource("/resources/Agrivilla.jpg") + "'</body></html>";
} else if (i==2){
image = "<html><body><img width= '800' height='500' src='" + getClass().getResource("/resources/Anada.jpg") + "'</body></html>";
} else if (i==3){
image = "<html><body><img width= '800' height='500' src='" + getClass().getResource("/resources/Cala Luna.jpg") + "'</body></html>";
} else if (i==4){
image = "<html><body><img width= '800' height='500' src='" + getClass().getResource("/resources/Sedona Restort.jpg") + "'</body></html>";
} else if (i==5){
image = "<html><body><img width= '800' height='500' src='" + getClass().getResource("/resources/Spa Eastman.jpg") + "'</body></html>";
}
return image;
}
/**
* Method to get the text values
*/
private String getTextDescription(int i) {
String text = "";
if (i==1){
text = "<html><body><font size='5'>#1 Agrivilla i pini - Italy</font> <br>A perfect detox retret in the rolling hills of Tuscany,"
+ "surronded by olive groves and vineyards.</body></html>";
} else if (i==2){
text = "<html><body><font size='5'>#2 Ananda in the Himalayas - India</font> <br>Famous for meditation and yoga!</body></html>";
} else if (i==3){
text = "<html><body><font size='5'>#3 Cala Luna Hotel and Villa - Costa Rica</font> <br>What better way to detox then a tropical paradise?"
+ "</body></html>";
} else if (i==4){
text = "<html><body><font size='5'>#4 Sedona Resort at Bell Rock - United States</font> <br>Take your mind out of the stress with"
+ "these breathingtaking canyons and spa!</body></html>";
} else if (i==5){
text = "<html><body><font size='5'>#5 Spa Eastman - Canada</text> <br>Wind down in these beautiful Nordic spas, famous"
+ "for its stress relief and eliminating toxins!</body></html>";
}
return text;
}
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
SlideShow ss = new SlideShow();
ss.setVisible(true);
}
});
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
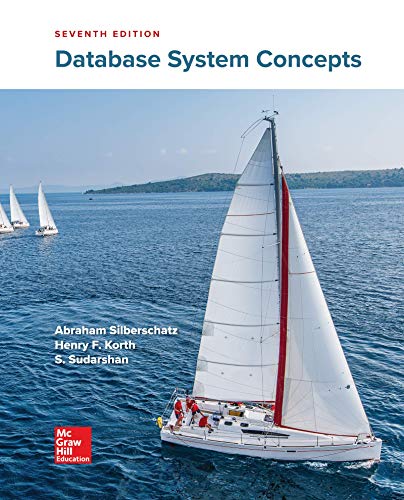
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
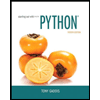
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
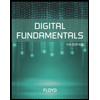
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
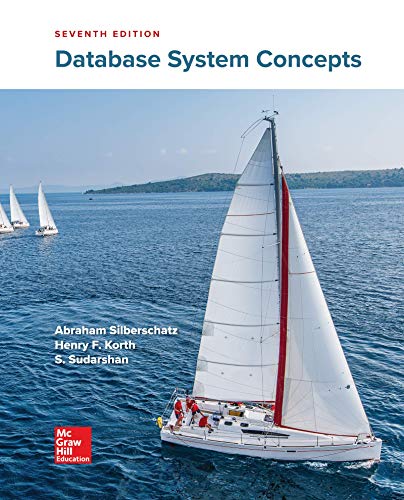
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
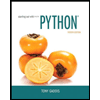
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
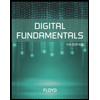
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
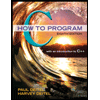
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
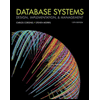
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
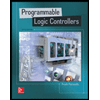
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education