Here is the code provided. I'm confused on the premise of these questions. Main(). #include #include "flexArray.h" using namespace std; int main() { flexArray f; f.SetLength(5); f.SetElement("Hello", 0); f.SetElement("World", 1); f.SetElement("THIS SHOULD FAIL", 6); for (int i = 0; i < f.GetLength(); i++) { cout << "Element " << i << " = " << f.GetElement(i) << endl; } f.SetLength(10); f.SetElement("THIS SHOULD NOT FAIL", 6); for (int i = 0; i < f.GetLength(); i++) { cout << "Element " << i << " = " << f.GetElement(i) << endl; } return 0; header file. #ifndef __FLEXARRAY_H__ #define __FLEXARRAY_H__ #include // This is an array of strings class flexArray { public: flexArray(); // Default constructor flexArray(unsigned int arrayLen); // Constructor with a length param ~flexArray(); // Destructor: cleans up dynamically-allocated memory unsigned int GetLength(); void SetLength(unsigned int updatedLength); std::string GetElement(unsigned int position); void SetElement(std::string update, unsigned int position); private: unsigned int _length; // this is the current length of the array std::string* _array; // this is where we put the actual values }; #endif /* __FLEXARRAY_H__ */ flexArray.cpp #include "flexArray.h" flexArray::flexArray() { // Default constructor // _length and _array _length = 100; // Setting the length to the default 100 _array = new std::string[_length]; // Dynamically allocate the array } flexArray::flexArray(unsigned int arrayLen) { // Constructor with a length param // _length and _array _length = arrayLen; // Setting the length to the parameter _array = new std::string[_length]; // Dynamically allocate the array } flexArray::~flexArray() { // Destructor: cleans up dynamically-allocated memory delete [] _array; // Deallocating the array before closing } unsigned int flexArray::GetLength() { return _length; } void flexArray::SetLength(unsigned int updatedLength) { // Step 1: Allocate space // Step 2: Copy over elements // Step 3: Delete old array // Step 4: Update with new array } std::string flexArray::GetElement(unsigned int position) { } void flexArray::SetElement(std::string update, unsigned int position) { }
Here is the code provided. I'm confused on the premise of these questions.
Main().
#include <iostream>
#include "flexArray.h"
using namespace std;
int main() {
flexArray f;
f.SetLength(5);
f.SetElement("Hello", 0);
f.SetElement("World", 1);
f.SetElement("THIS SHOULD FAIL", 6);
for (int i = 0; i < f.GetLength(); i++) {
cout << "Element " << i << " = " << f.GetElement(i) << endl;
}
f.SetLength(10);
f.SetElement("THIS SHOULD NOT FAIL", 6);
for (int i = 0; i < f.GetLength(); i++) {
cout << "Element " << i << " = " << f.GetElement(i) << endl;
}
return 0;
header file.
#ifndef __FLEXARRAY_H__
#define __FLEXARRAY_H__
#include <string>
// This is an array of strings
class flexArray {
public:
flexArray(); // Default constructor
flexArray(unsigned int arrayLen); // Constructor with a length param
~flexArray(); // Destructor: cleans up dynamically-allocated memory
unsigned int GetLength();
void SetLength(unsigned int updatedLength);
std::string GetElement(unsigned int position);
void SetElement(std::string update, unsigned int position);
private:
unsigned int _length; // this is the current length of the array
std::string* _array; // this is where we put the actual values
};
#endif /* __FLEXARRAY_H__ */
flexArray.cpp
#include "flexArray.h"
flexArray::flexArray() { // Default constructor
// _length and _array
_length = 100; // Setting the length to the default 100
_array = new std::string[_length]; // Dynamically allocate the array
}
flexArray::flexArray(unsigned int arrayLen) { // Constructor with a length param
// _length and _array
_length = arrayLen; // Setting the length to the parameter
_array = new std::string[_length]; // Dynamically allocate the array
}
flexArray::~flexArray() { // Destructor: cleans up dynamically-allocated memory
delete [] _array; // Deallocating the array before closing
}
unsigned int flexArray::GetLength() {
return _length;
}
void flexArray::SetLength(unsigned int updatedLength) {
// Step 1: Allocate space
// Step 2: Copy over elements
// Step 3: Delete old array
// Step 4: Update with new array
}
std::string flexArray::GetElement(unsigned int position) {
}
void flexArray::SetElement(std::string update, unsigned int position) {
}


Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 4 images

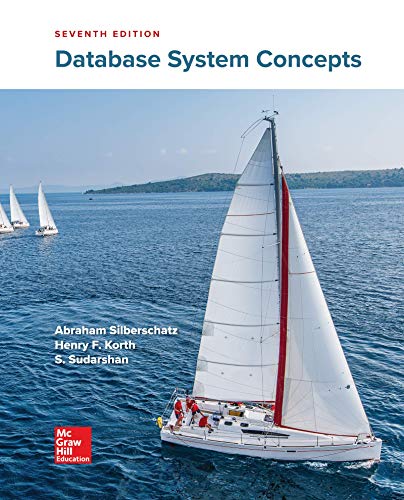
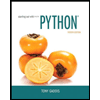
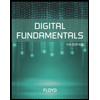
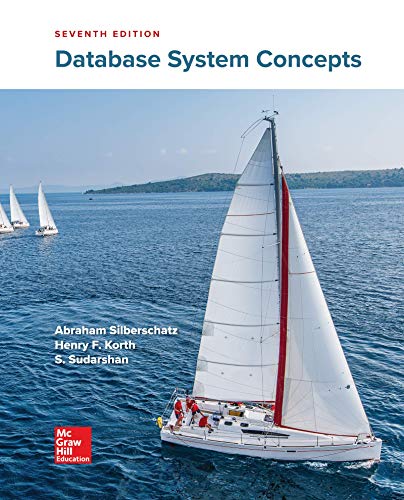
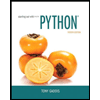
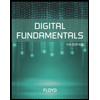
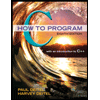
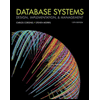
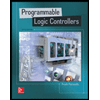