Create a program using java data structures That has the user enter their pay rate; the program will require the employee to enter hours for two days ( they work two days a week); and the conversion must be done. via a recursive function (all calculation and display happen in a single function). Include an additional function to prompt the user for their numbers. Do NOT USE Stack ADT, ANY ADTs, or structures that we created in class. Employee - hoursWorked : int - payRate : double -Employee(void) Default constructor - has NO parameters and must NOT return anything. Sets both attributes to 0. -AddHours(hoursToAdd : int) : void Adds the given hours to add to the hoursWorked attribute. -SetPayRate(newPayRate : double) : void Changes the payrate attribute to the given new pay rate. -GetHoursWorked(void) : int {hoursWorked} Gives back the hoursWorked attribute -+ GetPayRate(void) : double {payRate} Gives back the payRate attribute. -GetGrossPay(void) : double {grossPay} Computes and returns the gross pay DeterminePay -main(args : String[ ]) : void Gets the pay rate, gets the hours worked for two different days, and displays a pay summary. Placing the information into the object at the correct moments also occurs in here. - GetPayRate(void) : double {newPayRate} Prompts the user for the new pay rate and returns it. -GetHoursWorked(void) : int {hoursToAdd} Prompts the user for the number of hours worked on a single day and returns it. -DisplayPaySummary(anEmployee : Employee) : void Displays the hours worked, payrate, and gross pay to the user
Create a
That has the user enter their pay rate; the program will require the employee to enter hours for two days ( they work two days a week); and the conversion must be done. via a recursive function (all calculation and display happen in a single function). Include an additional function to prompt the user for their numbers. Do NOT USE Stack ADT, ANY ADTs, or structures that we created in class.
Employee
- hoursWorked : int
- payRate : double
-Employee(void)
Default constructor - has NO parameters and must NOT return anything. Sets both attributes to 0.
-AddHours(hoursToAdd : int) : void
Adds the given hours to add to the hoursWorked attribute.
-SetPayRate(newPayRate : double) : void
Changes the payrate attribute to the given new pay rate.
-GetHoursWorked(void) : int {hoursWorked}
Gives back the hoursWorked attribute
-+ GetPayRate(void) : double {payRate}
Gives back the payRate attribute.
-GetGrossPay(void) : double {grossPay}
Computes and returns the gross pay
DeterminePay
-main(args : String[ ]) : void
Gets the pay rate, gets the hours worked for two different days, and displays a pay summary. Placing the information into the object at the correct moments also occurs in here.
- GetPayRate(void) : double {newPayRate}
Prompts the user for the new pay rate and returns it.
-GetHoursWorked(void) : int {hoursToAdd}
Prompts the user for the number of hours worked on a single day and returns it.
-DisplayPaySummary(anEmployee : Employee) : void
Displays the hours worked, payrate, and gross pay to the user

Step by step
Solved in 4 steps with 3 images

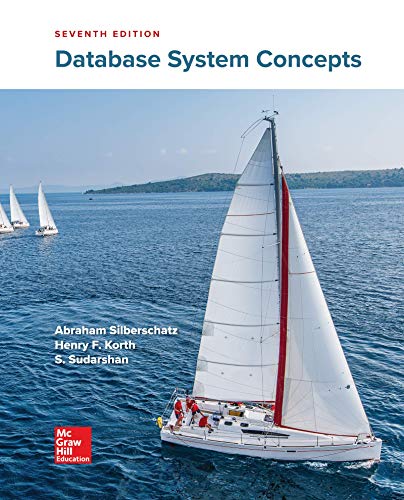
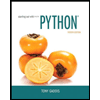
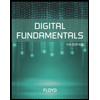
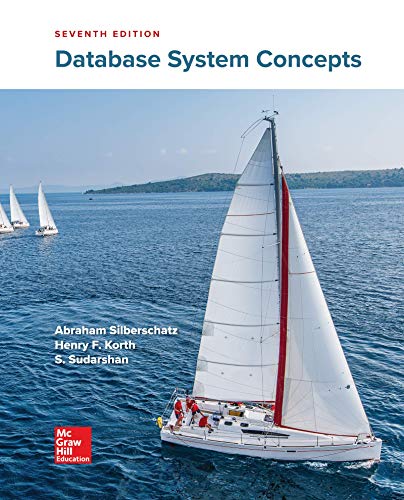
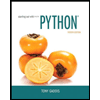
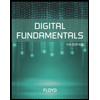
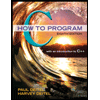
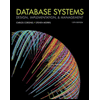
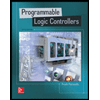