• You are given a class called Shape, an enumeration called ShapeType, and a starting point to the main function. • Create one class per shape required O Each class should be split into header/implementation files as we described in class o Each class should publicly inherit from the Shape class o Each class should have a fully parametrized constructor The number of parameters in the constructor depend on the values needed to calculate the shape's perimeter and area (one double for circle, two doubles for rectangle, etc.) It should only contain parameters needed for the parameter and area
This assignment is not graded, I just need to understand how to do it. Please help, thank you!
Language: C++
Given:
Main.cpp
#include
#include "Shape.h"
using namespace std;
void main() {
/////// Untouchable Block #1 //////////
Shape* shape;
/////// End of Untouchable Block #1 //////////
/////// Untouchable Block #2 //////////
if (shape == nullptr) {
cout << "What shape is this?! Good bye!";
return;
}
cout << "The perimeter of your " << shape->getShapeName() << ": " <<
shape->getPerimeter() << endl;
cout << "The area of your " << shape->getShapeName() << ": " <<
shape->getArea() << endl;
/////// End of Untouchable Block #2 //////////
}
Shape.cpp
string Shape::getShapeName() {
switch (mShapeType) {
case ShapeType::CIRCLE:
return "circle";
case ShapeType::SQUARE:
return "square";
case ShapeType::RECTANGLE:
return "rectangle";
case ShapeType::TRIANGLE:
return "triangle";
default:
return "unknown shape";
}
return "unknown shape";
}
ShapeType.h
#pragma once
enum class ShapeType {
CIRCLE,
SQUARE,
RECTANGLE,
TRIANGLE
};
Shape.h
#pragma once
#include
#include "ShapeType.h"
using namespace std;
class Shape {
private:
ShapeType mShapeType;
double mPerimenter;
double mArea;
public:
Shape(ShapeType shapeType);
~Shape();
protected:
void setPerimenter(double perimeter);
void setArea(double area);
public:
double getPerimeter();
double getArea();
ShapeType getShapeType();
string getShapeName();
};



Step by step
Solved in 2 steps

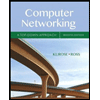
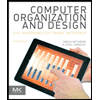
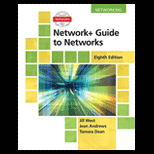
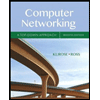
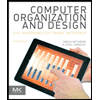
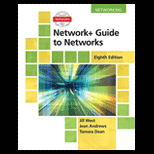
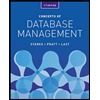
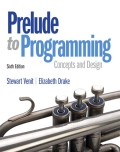
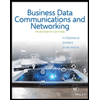