Implement a data structure called RunningTotal supporting the following API: a) RunningTotal() - creates an empty running-total data structure b) void add(int value) - adds the given value to the data structure c) void remove() - removes the least-recently added value d) int sum() - returns the sum of values in the data structure e) double average() - returns the average of values in the data structure
*Java code*
Implement a data structure called RunningTotal supporting the following API:
a) RunningTotal() - creates an empty running-total data structure
b) void add(int value) - adds the given value to the data structure
c) void remove() - removes the least-recently added value
d) int sum() - returns the sum of values in the data structure
e) double average() - returns the average of values in the data structure
Here's a Example:
RunningTotal rt = new RunningTotal ();
rt . add (1); // 1 ( adds 1 )
rt . add (2); // 1 2 ( adds 2 )
rt . add (3); // 1 2 3 ( adds 3 )
rt . sum (); // 1 2 3 ( returns 6 )
rt . add (4); // 1 2 3 4 ( adds 4 )
rt . remove (); // 2 3 4 ( removes 1 )
rt . sum (); // 2 3 4 ( returns 9 )
rt . average (); // 2 3 4 ( returns 3.0 )

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

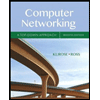
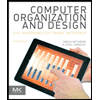
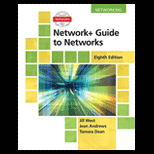
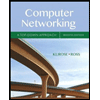
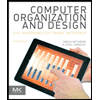
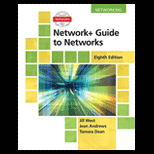
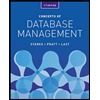
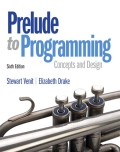
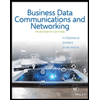