Given this class definition: // This class implements a simplified form of the native C++ string. (Essentially a c-String) class newString { //Overload the stream insertion and extraction operators. friend ostream& operator << (ostream&, const newString&); friend istream& operator >> (istream&, newString&); public: // assignment overload here // * (multiplication) overload here // Constructors and destructor here private: char *strPtr; //pointer to the char array that holds the string int strLength; //variable to store the length of the string }; 1)Extend the above definition to include prototypes for the default and copy constructors, and the destructor, as well as overloads for the assignment and * operators. (See step 2 for example of the * operator.) 2) Write the definitions for the functions added in step 1. Note: The * operator will create a string with x concatenated copies of the first operand as shown here: newString s1 = "Hello"; newString s2 = s1 * 3; cout << s2; Output: HelloHelloHello 3)Provide a test program demonstrating the working class. Note: You can assume all code is contained in a single file and the definitions of any other functions shown have been provide
Given this class definition:
// This class implements a simplified form of the native C++ string. (Essentially a c-String)
class newString {
//Overload the stream insertion and extraction operators.
friend ostream& operator << (ostream&, const newString&);
friend istream& operator >> (istream&, newString&);
public:
// assignment overload here
// * (multiplication) overload here
// Constructors and destructor here
private:
char *strPtr; //pointer to the char array that holds the string
int strLength; //variable to store the length of the string
};
1)Extend the above definition to include prototypes for the default and copy constructors, and the destructor, as well as overloads for the assignment and * operators. (See step 2 for example of the * operator.)
2) Write the definitions for the functions added in step 1. Note: The * operator will create a string with x concatenated copies of the first operand as shown here:
newString s1 = "Hello";
newString s2 = s1 * 3;
cout << s2;
Output: HelloHelloHello
3)Provide a test program demonstrating the working class.
Note: You can assume all code is contained in a single file and the definitions of any other functions shown have been provided.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

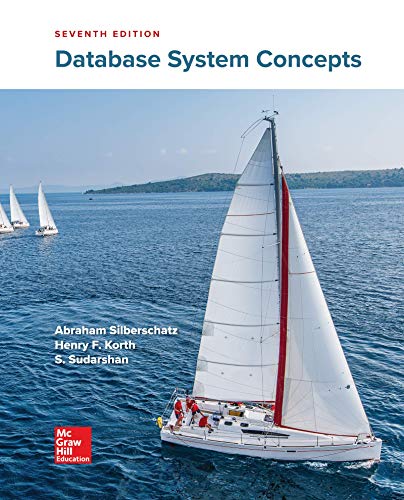
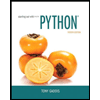
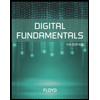
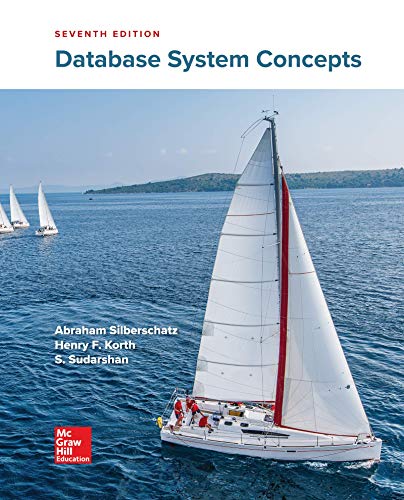
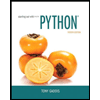
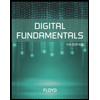
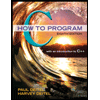
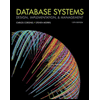
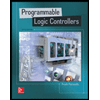