In c++ Please. Given main(), complete class RandomNumbers (in files RandomNumbers.h and RandomNumbers.cpp) that has three integer data members: var1, var2, and var3. Write a getter function for each variable: getVar1(), getVar2() and getVar3(). Then write the following 2 member functions: SetRandomValues( ) - accepts a low and high integer values as parameters, and sets var1, var2, and var3 to random numbers (generated using the random function) within the range of the low and high input values (inclusive). GetRandomValues( ) - prints out the 3 random numbers in the format: "Random values: var1 var2 var3" Ex: If the input is: 15 20 the output is: Random values: 17 15 19 where 17, 15, 19 can be any random numbers within 15 and 20 (inclusive). Note: When submitted, your program will be tested against different input range to verify if the three randomly generated values are within range. RandomNumbers.h #ifndef RANDOMNUMBERS_H_ #define RANDOMNUMBERS_H_ #include using namespace std; class RandomNumbers { public: void SetRandomValues(int, int); void GetRandomValues(); int GetVar1(); int GetVar2(); int GetVar3(); private: int var1; int var2; int var3; }; #endif /* RANDOMNUMBERS_H_ */ Main.cpp #include #include "RandomNumbers.h" using namespace std; int main() { int low; int high; cin >> low; cin >> high; RandomNumbers rn = RandomNumbers(); rn.SetRandomValues(low, high); rn.GetRandomValues(); return 0; } RandomNumbers.cpp #include #include "RandomNumbers.h" using namespace std; // to do
In c++ Please.
Given main(), complete class RandomNumbers (in files RandomNumbers.h and RandomNumbers.cpp) that has three integer data members: var1, var2, and var3. Write a getter function for each variable: getVar1(), getVar2() and getVar3(). Then write the following 2 member functions:
- SetRandomValues( ) - accepts a low and high integer values as parameters, and sets var1, var2, and var3 to random numbers (generated using the random function) within the range of the low and high input values (inclusive).
- GetRandomValues( ) - prints out the 3 random numbers in the format: "Random values: var1 var2 var3"
Ex: If the input is:
15 20
the output is:
Random values: 17 15 19
where 17, 15, 19 can be any random numbers within 15 and 20 (inclusive).
Note: When submitted, your program will be tested against different input range to verify if the three randomly generated values are within range.
RandomNumbers.h
#ifndef RANDOMNUMBERS_H_
#define RANDOMNUMBERS_H_
#include <iostream>
using namespace std;
class RandomNumbers {
public:
void SetRandomValues(int, int);
void GetRandomValues();
int GetVar1();
int GetVar2();
int GetVar3();
private:
int var1;
int var2;
int var3;
};
#endif /* RANDOMNUMBERS_H_ */
Main.cpp
#include <iostream>
#include "RandomNumbers.h"
using namespace std;
int main() {
int low;
int high;
cin >> low;
cin >> high;
RandomNumbers rn = RandomNumbers();
rn.SetRandomValues(low, high);
rn.GetRandomValues();
return 0;
}
RandomNumbers.cpp
#include <iostream>
#include "RandomNumbers.h"
using namespace std;
// to do

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

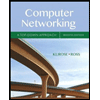
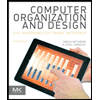
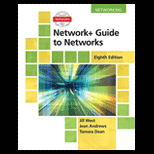
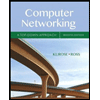
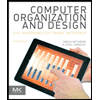
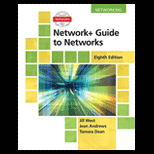
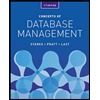
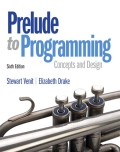
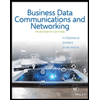