The encryption and decryption for the Substitution is not working please fix that. #include #include using namespace std; class Cipher { public: virtual string encode() = 0; virtual string decode() = 0; }; class SubstitutionCipher : Cipher { // int key; string key; string input; // static string _encode(string input, int key); string _getValidKey(); static bool isKeyValid(string key); public: //constructor SubstitutionCipher(string msg, string k) { this->input = msg; if (isKeyValid(k)) { this->key = k; } else { this->key = _getValidKey(); } } //the function for encryption/decryption (CaesarCipher algorithm) string encode(); string decode(); }; class CaesarCipher : Cipher { int key; string input; static string _encode(string input, int key); public: //constructor CaesarCipher(string msg, int k) : input(msg), key(k) {} //the function for encryption/decryption (CaesarCipher algorithm) string encode(); string decode(); }; /////////////////////////////////////////////////////////////////////// //the function for encryption/decryption (Substitution algorithm) // Encryption bool SubstitutionCipher::isKeyValid(string key) { if (key.length() != 26) { return false; } const int ARRAY_SIZE = 255; bool *arr = new bool[ARRAY_SIZE]; for (int i = 0; i < ARRAY_SIZE; i++) { arr[i] = false; } for (auto character : key) { if (arr[character] == true || tolower(character) < 97 || toupper(character) > 122) { delete arr; return false; } } return true; } string SubstitutionCipher::_getValidKey() { string key; cout << "Enter key: "; getline(cin, key); if (isKeyValid(key)) { return key; } return SubstitutionCipher::_getValidKey(); } string SubstitutionCipher::encode() { char ch; string output(input.length(), '\0'); for (int i = 0; i < input.length(); i++) { ch = tolower(input[i]); if (ch < 97 || ch > 122) { output[i] = ch; } else { output[i] = key[ch - 97]; } } return output; } string SubstitutionCipher::decode() { char ch; string output(input.length(), '\0'); for (int i = 0; i < input.length(); i++) { ch = tolower(input[i]); if (ch < 97 || ch > 122) { output[i] = ch; } else { int index = key.find(ch); output[i] = 'a' + index; // output[i] = key[ch - 97]; } } return output; } /////////////////////////////////////////////////////////////////////// //the function for encryption/decryption (CaesarCipher algorithm) string CaesarCipher::_encode(string input, int key) { string output = ""; for (int i = 0; i < input.size(); ++i) { if (isalpha(input[i])) //if letter { //offset='A' for upper letter, offset='a' for lowel letter char offset = isupper(input[i]) ? 'A' : 'a'; //convert letter (shift letter) char ch = (char)(((input[i] + key - offset) % 26) + offset); output += ch; } else { //non letter output += input[i]; } } return output; } string CaesarCipher::encode() { return CaesarCipher::_encode(this->input, this->key % 26); } string CaesarCipher::decode() { return CaesarCipher::_encode(this->input, 26 - (this->key % 26)); } /////////////////////////////////////////////////////////////////////// int main() { /* Encrypt a string entered by the user Choose between two different encryption methods Decrypt a string entered by the user Choose between two different decryptions methods Decrypt without knowing the encryption method (provide all possible outputs) */ string source = ""; int option; int caesarKey; string substitutionKey; do { cout << "\nEnter the text>> " << flush; getline(cin, source); cin.ignore(); // cin.ignore(256, '\n'); cout << "Make your choice" << endl; cout << "\t1. Mono-alphabetic substitution cipher, encrypt" << endl; cout << "\t2. Mono-alphabetic substitution cipher, decrypt" << endl; cout << "\t3. Caesar cipher, encrypt" << endl; cout << "\t4. Caesar cipher, decrypt" << endl; cout << "\t5. Decrypt without knowing the encryption method (provide all possible outputs)" << endl; cout << "\t6. Exit" << endl; cout << ">> "; cin >> option; cin.ignore(); string result; switch (option) { case 1: { cout << "Enter key for substitution cipher: "; getline(cin, substitutionKey); cin.ignore(); SubstitutionCipher s(source, substitutionKey); result = s.encode(); cout << endl << "The encryption string:\n" << result << "\n"; break; } case 2: { cout << "Enter key for substitution cipher: "; getline(cin, substitutionKey); cin.ignore(); SubstitutionCipher s(source, substitutionKey); result = s.decode(); cout << endl << "The decryption string:\n" << result << "\n"; break; } case 3: { cout << "Enter key for caesar cipher: "; cin >> caesarKey; cin.ignore(); CaesarCipher t(source, caesarKey); result = t.encode(); cout << endl << "The encryption string:\n" << result << "\n"; break; } case 4: { cout << "Enter key for caesar cipher: "; cin >> caesarKey; cin.ignore(); CaesarCipher t(source, caesarKey); result = t.decode(); cout << endl << "The decryption string:\n" << result << "\n"; break; } case 5: { cout << "All permutations using Caesar cipher" << endl; for (int i = 0; i < 25; i++) { CaesarCipher t(source, i); result = t.decode(); cout << result << endl; } // You can try all permutations of the string "abcdefghijklmnopqrstuvwxyz" // for the mono-alphabetic substitution cipher break; } case 6: cout << endl << "Goodbye!" << endl; break; } } while (option != 6); return 0; }
The encryption and decryption for the Substitution is not working please fix that.
#include <iostream>
#include <cstring>
using namespace std;
class Cipher
{
public:
virtual string encode() = 0;
virtual string decode() = 0;
};
class SubstitutionCipher : Cipher
{
// int key;
string key;
string input;
// static string _encode(string input, int key);
string _getValidKey();
static bool isKeyValid(string key);
public:
//constructor
SubstitutionCipher(string msg, string k)
{
this->input = msg;
if (isKeyValid(k))
{
this->key = k;
}
else
{
this->key = _getValidKey();
}
}
//the function for encryption/decryption (CaesarCipher
string encode();
string decode();
};
class CaesarCipher : Cipher
{
int key;
string input;
static string _encode(string input, int key);
public:
//constructor
CaesarCipher(string msg, int k) : input(msg), key(k) {}
//the function for encryption/decryption (CaesarCipher algorithm)
string encode();
string decode();
};
///////////////////////////////////////////////////////////////////////
//the function for encryption/decryption (Substitution algorithm)
// Encryption
bool SubstitutionCipher::isKeyValid(string key)
{
if (key.length() != 26)
{
return false;
}
const int ARRAY_SIZE = 255;
bool *arr = new bool[ARRAY_SIZE];
for (int i = 0; i < ARRAY_SIZE; i++)
{
arr[i] = false;
}
for (auto character : key)
{
if (arr[character] == true || tolower(character) < 97 || toupper(character) > 122)
{
delete arr;
return false;
}
}
return true;
}
string SubstitutionCipher::_getValidKey()
{
string key;
cout << "Enter key: ";
getline(cin, key);
if (isKeyValid(key))
{
return key;
}
return SubstitutionCipher::_getValidKey();
}
string SubstitutionCipher::encode()
{
char ch;
string output(input.length(), '\0');
for (int i = 0; i < input.length(); i++)
{
ch = tolower(input[i]);
if (ch < 97 || ch > 122)
{
output[i] = ch;
}
else
{
output[i] = key[ch - 97];
}
}
return output;
}
string SubstitutionCipher::decode()
{
char ch;
string output(input.length(), '\0');
for (int i = 0; i < input.length(); i++)
{
ch = tolower(input[i]);
if (ch < 97 || ch > 122)
{
output[i] = ch;
}
else
{
int index = key.find(ch);
output[i] = 'a' + index;
// output[i] = key[ch - 97];
}
}
return output;
}
///////////////////////////////////////////////////////////////////////
//the function for encryption/decryption (CaesarCipher algorithm)
string CaesarCipher::_encode(string input, int key)
{
string output = "";
for (int i = 0; i < input.size(); ++i)
{
if (isalpha(input[i])) //if letter
{
//offset='A' for upper letter, offset='a' for lowel letter
char offset = isupper(input[i]) ? 'A' : 'a';
//convert letter (shift letter)
char ch = (char)(((input[i] + key - offset) % 26) + offset);
output += ch;
}
else
{
//non letter
output += input[i];
}
}
return output;
}
string CaesarCipher::encode()
{
return CaesarCipher::_encode(this->input, this->key % 26);
}
string CaesarCipher::decode()
{
return CaesarCipher::_encode(this->input, 26 - (this->key % 26));
}
///////////////////////////////////////////////////////////////////////
int main()
{
/*
Encrypt a string entered by the user
Choose between two different encryption methods
Decrypt a string entered by the user
Choose between two different decryptions methods
Decrypt without knowing the encryption method (provide all possible outputs)
*/
string source = "";
int option;
int caesarKey;
string substitutionKey;
do
{
cout << "\nEnter the text>> " << flush;
getline(cin, source);
cin.ignore();
// cin.ignore(256, '\n');
cout << "Make your choice" << endl;
cout << "\t1. Mono-alphabetic substitution cipher, encrypt" << endl;
cout << "\t2. Mono-alphabetic substitution cipher, decrypt" << endl;
cout << "\t3. Caesar cipher, encrypt" << endl;
cout << "\t4. Caesar cipher, decrypt" << endl;
cout << "\t5. Decrypt without knowing the encryption method (provide all possible outputs)" << endl;
cout << "\t6. Exit" << endl;
cout << ">> ";
cin >> option;
cin.ignore();
string result;
switch (option)
{
case 1:
{
cout << "Enter key for substitution cipher: ";
getline(cin, substitutionKey);
cin.ignore();
SubstitutionCipher s(source, substitutionKey);
result = s.encode();
cout << endl
<< "The encryption string:\n"
<< result << "\n";
break;
}
case 2:
{
cout << "Enter key for substitution cipher: ";
getline(cin, substitutionKey);
cin.ignore();
SubstitutionCipher s(source, substitutionKey);
result = s.decode();
cout << endl
<< "The decryption string:\n"
<< result << "\n";
break;
}
case 3:
{
cout << "Enter key for caesar cipher: ";
cin >> caesarKey;
cin.ignore();
CaesarCipher t(source, caesarKey);
result = t.encode();
cout << endl
<< "The encryption string:\n"
<< result << "\n";
break;
}
case 4:
{
cout << "Enter key for caesar cipher: ";
cin >> caesarKey;
cin.ignore();
CaesarCipher t(source, caesarKey);
result = t.decode();
cout << endl
<< "The decryption string:\n"
<< result << "\n";
break;
}
case 5:
{
cout << "All permutations using Caesar cipher" << endl;
for (int i = 0; i < 25; i++) {
CaesarCipher t(source, i);
result = t.decode();
cout << result << endl;
}
// You can try all permutations of the string "abcdefghijklmnopqrstuvwxyz"
// for the mono-alphabetic substitution cipher
break;
}
case 6:
cout << endl
<< "Goodbye!" << endl;
break;
}
} while (option != 6);
return 0;
}

Step by step
Solved in 2 steps with 4 images

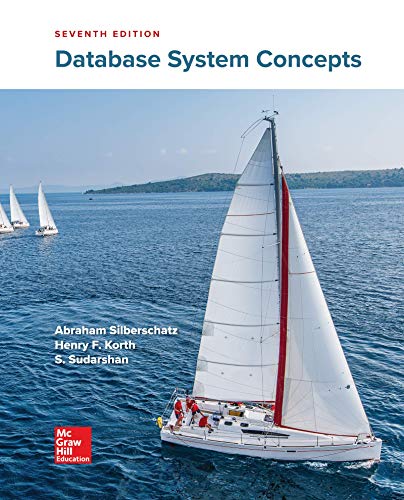
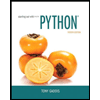
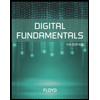
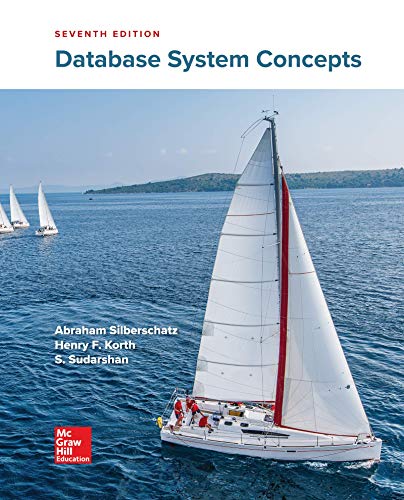
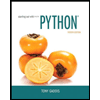
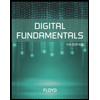
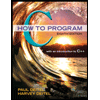
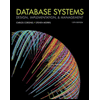
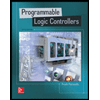