In c++ Please show me the Test output *You may not use any STL containers in your implementations Need help with the following functions: -LinkedList(const LinkedList& other_list) Constructs a container with a copy of each of the elements in another, in the same order. -LinkedList& operator=(const LinkedList& other_list) Replaces the contents of this list with a copy of each element in another, in the same order. -void resize(std::size_t n) resizes the list so that it contains n elements. -void resize(std::size_t n, const T &fill_values) resizes the list so that it contains n elements -void remove(const T &val) Removes from the container all the elements that compare equal to val - bool operator == (const LinkedList &another) Compares this list with another for equality - bool operator != (const LinkedList &another) Compares this list with another for equality CODE #include template class LinkedList { struct Node; private: struct Node { // Constructors T data; Node* next = nullptr; Node* prev = nullptr; }; Node* head_ = nullptr; Node* tail_ = nullptr; std::size_t size_ = 0; public: /** Constructs a list with a copy of each of the elements in `initial_list`, in the same order. */ LinkedList(std::initializer_list initial_list) { this->operator=(initial_list); } /**Constructs a container with a copy of each of the elements in other_list, in the same order**/ LinkedList(const LinkedList& other_list){ //TODO } /** Replaces the contents of this list with a copy of each element in `initial_list`. */ LinkedList& operator=(std::initializer_list initial_list) { // We should empty the list first, but for now... this->size_ = 0; for (auto&& val : initial_list) this->push_back(val); return *this; } /**Replaces the contents of this list with a copy of each element in other_list, in the same order.*/ LinkedList& operator=(const LinkedList& other_list){ //TODO } /**Compares this list with another for equality.*/ bool operator == (const LinkedList &another){ // TODO } /**Compares this list with another for equality.*/ bool operator != (const LinkedList &another){ // TODO } /** Destroys each of the contained elements, and deallocates all memory allocated by this list. */ ~LinkedList() { while (this->head_) { Node* old_head = this->head_; this->head_ = old_head->next; delete old_head; } } /**resizes the list so that it contains n elements.*/ void resize(std::size_t n){ //TODO } /**resizes the list so that it contains n elements*/ void resize(std::size_t n, const T &fill_values){ //TODO } /**Removes from the container all the elements that compare equal to val. */ void remove(const T &val){ //TODO } /**Returns the number of elements in the list*/ [[nodiscard]] size_t size() const{ return size_; } /**Returns whether the list container is empty */ [[nodiscard]] bool empty() const{ if(size_ == 0){ return true; } else{ return false; } } /** Appends a copy of `val` to this list. */ void push_back(const T& val) { Node* new_node = new Node{val}; if (this->size_ == 0) { this->head_ = this->tail_ = new_node; } else { this->tail_->next = new_node; new_node->prev = this->tail_; this->tail_ = new_node; } ++this->size_; } /** Prepends a copy of `val` to this list. */ void push_front(const T& val) { Node* new_node = new Node{val}; if (this->size_ == 0) { this->head_ = this->tail_ = new_node; } else { new_node->next = this->head_; this->head_->prev = new_node; this->head_ = new_node; } ++this->size_; } /**Deletes all values in this list.*/ void clear(){ Node *temp = head_; while (temp){ temp = temp->next; delete temp; } size_ = 0; } friend std::ostream& operator<<(std::ostream& out, const LinkedList& list) { for (Node* cur = list.head_; cur; cur = cur->next) { out << cur->data; } return out; } }; int main() { /***TESTING***/ LinkedList list{'H','E','L','L','O'}; std::cout< copyList = list; copyList.resize(10, '!'); // HELLO! std::cout << copyList << '\n'; copyList.remove('O'); //HELL! std::cout << copyList << '\n'; return 0; }
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
In c++
Please show me the Test output
*You may not use any STL containers in your implementations
Need help with the following functions:
-LinkedList(const LinkedList<T>& other_list)
Constructs a container with a copy of each of the elements in another, in the same order.
-LinkedList& operator=(const LinkedList& other_list)
Replaces the contents of this list with a copy of each element in another, in the same order.
-void resize(std::size_t n)
resizes the list so that it contains n elements.
-void resize(std::size_t n, const T &fill_values)
resizes the list so that it contains n elements
-void remove(const T &val)
Removes from the container all the elements that compare equal to val
- bool operator == (const LinkedList &another)
Compares this list with another for equality
- bool operator != (const LinkedList &another)
Compares this list with another for equality
CODE
#include <iostream>
template <typename T>
class LinkedList {
struct Node;
private:
struct Node {
// Constructors
T data;
Node* next = nullptr;
Node* prev = nullptr;
};
Node* head_ = nullptr;
Node* tail_ = nullptr;
std::size_t size_ = 0;
public:
/** Constructs a list with a copy of each of the elements in `initial_list`, in the same order. */
LinkedList(std::initializer_list<T> initial_list) {
this->operator=(initial_list);
}
/**Constructs a container with a copy of each of the elements in other_list, in the same order**/
LinkedList(const LinkedList<T>& other_list){
//TODO
}
/** Replaces the contents of this list with a copy of each element in `initial_list`. */
LinkedList& operator=(std::initializer_list<T> initial_list) {
// We should empty the list first, but for now...
this->size_ = 0;
for (auto&& val : initial_list)
this->push_back(val);
return *this;
}
/**Replaces the contents of this list with a copy of each element in other_list, in the same order.*/
LinkedList& operator=(const LinkedList& other_list){
//TODO
}
/**Compares this list with another for equality.*/
/**Compares this list with another for equality.*/
/** Destroys each of the contained elements, and deallocates all memory allocated by this list. */
~LinkedList() {
while (this->head_) {
Node* old_head = this->head_;
this->head_ = old_head->next;
delete old_head;
}
}
/**resizes the list so that it contains n elements.*/
void resize(std::size_t n){
//TODO
}
/**resizes the list so that it contains n elements*/
void resize(std::size_t n, const T &fill_values){
//TODO
}
/**Removes from the container all the elements that compare equal to val. */
void remove(const T &val){
//TODO
}
/**Returns the number of elements in the list*/
[[nodiscard]] size_t size() const{
return size_;
}
/**Returns whether the list container is empty */
[[nodiscard]] bool empty() const{
if(size_ == 0){
return true;
} else{
return false;
}
}
/** Appends a copy of `val` to this list. */
void push_back(const T& val) {
Node* new_node = new Node{val};
if (this->size_ == 0) {
this->head_ = this->tail_ = new_node;
} else {
this->tail_->next = new_node;
new_node->prev = this->tail_;
this->tail_ = new_node;
}
++this->size_;
}
/** Prepends a copy of `val` to this list. */
void push_front(const T& val) {
Node* new_node = new Node{val};
if (this->size_ == 0) {
this->head_ = this->tail_ = new_node;
} else {
new_node->next = this->head_;
this->head_->prev = new_node;
this->head_ = new_node;
}
++this->size_;
}
/**Deletes all values in this list.*/
void clear(){
Node *temp = head_;
while (temp){
temp = temp->next;
delete temp;
}
size_ = 0;
}
friend std::ostream& operator<<(std::ostream& out, const LinkedList& list) {
for (Node* cur = list.head_; cur; cur = cur->next) {
out << cur->data;
}
return out;
}
};
int main() {
/***TESTING***/
LinkedList<char> list{'H','E','L','L','O'};
std::cout<<list<<'\n';
LinkedList<char> copyList = list;
copyList.resize(10, '!'); // HELLO!
std::cout << copyList << '\n';
copyList.remove('O'); //HELL!
std::cout << copyList << '\n';
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

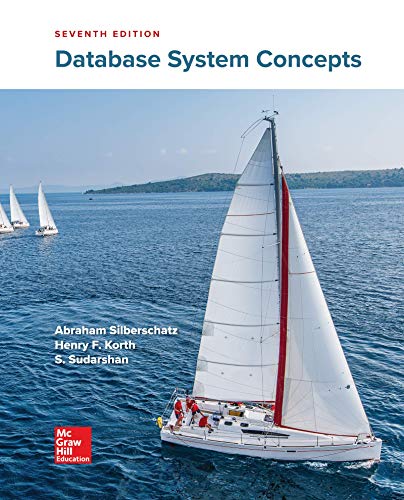
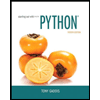
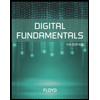
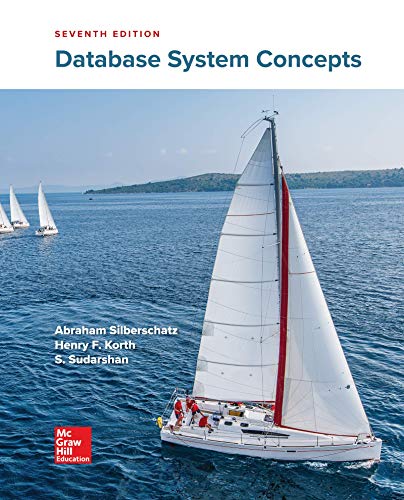
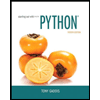
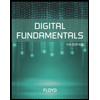
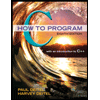
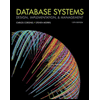
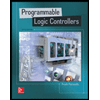