This is only stage 1 of 5 on a C++ coding lab I am struggling with. Here is my code so far: #include #include #include #include #include #include using namespace std; vector dictionary1 = {"airy", "aisle", "aisles", "ajar", "akimbo", "akin", "juveniles", "juxtapose", "knowledges", "known", "president", "tries", "trifle", "tugs", "wrongdoers", "wroth", "wyvern", "xenophon", "xylol", "yodle", "yurt", "zeugma", "ziggurat", "zootomy"}; vector dictionary2 = {"aback", "abased", "acknowledgers", "administers", "affair", "aforementioned", "aggrieving", "agitating", "agree", "airlines", "ajar", "basin", "bawdy", "cheap", "cheated", "examiner", "excel", "lewdness", "liberal", "mathematician", "ordered", "president", "sandwich", "swagger", "swarm", "vomit", "yell", "zero" "zodiac", "zoo"}; vector dictionary3 = {"ajar", "anachronism", "basin", "bawdy", "bleed", "bystander", "chariot", "cheap", "cheated", "clay", "contrive", "critiques", "databases", "derivative", "dog", "earthenware", "echo", "examiner", "excel", "fatiguing", "floppy", "goldsmith", "halt", "implies", "jam", "klutz", "lively", "malt", "meteor", "nonsense", "orphans", "paint", "playful", "railroad", "revolt", "shark", "spook", "syntax", "tablet", "thing", "ugly", "vigilant", "whirr", "yell", "zap", "zoo"}; void add_word(vector& dictionary, const string& word) { dictionary.push_back(word); sort(dictionary.begin(), dictionary.end()); } void delete_word(vector& dictionary, const string& word) { dictionary.erase(remove(dictionary.begin(), dictionary.end(), word), dictionary.end()); } int search_word(const vector& dictionary, const string& word) { for (size_t i = 0; i < dictionary.size(); ++i) { if (dictionary[i] == word) { return i; } } return -1; } void swap_words(vector& dictionary, const string& word1, const string& word2) { int idx1 = search_word(dictionary, word1); int idx2 = search_word(dictionary, word2); if (idx1 != -1 && idx2 != -1) { swap(dictionary[idx1], dictionary[idx2]); } } int main() { int dictionary_choice; string search, del; vector> dictionaries = {dictionary1, dictionary2, dictionary3}; cout << "Which Dictionary should be opened? Enter \"1\", \"2\", or \"3\": "; cin >> dictionary_choice; dictionary_choice--; int idx, del_idx; while (true) { cout << "\n--------------------------------------------\n" << "Options menu: \n" << "(1) Print words\n" << "(2) Find a word\n" << "(3) Find word, insert if found (assumes words are sorted alphabetically)\n" << "(4) Find word, delete if found \n" << "(5) Swap two words\n" << "(6) Sort words (Bubble Sort or Selection Sort)\n" << "(7) Find a word - Binary Search (assumes words are sorted alphabetically)\n" << "(8) Merge two dictionaries (will sort first)\n" << "(9) Write current dictionary to file\n" << "Enter a number from 1 to 9, or 0 to exit: "; int choice; cin >> choice;
This is only stage 1 of 5 on a C++ coding lab I am struggling with. Here is my code so far:
#include <iostream>
#include <
#include <fstream>
#include <string>
#include <algorithm>
#include <iterator>
using namespace std;
vector<string> dictionary1 = {"airy", "aisle", "aisles", "ajar", "akimbo", "akin", "juveniles", "juxtapose", "knowledges", "known", "president", "tries", "trifle", "tugs", "wrongdoers", "wroth", "wyvern", "xenophon", "xylol", "yodle", "yurt", "zeugma", "ziggurat", "zootomy"};
vector<string> dictionary2 = {"aback", "abased", "acknowledgers", "administers", "affair", "aforementioned", "aggrieving", "agitating", "agree", "airlines", "ajar", "basin", "bawdy", "cheap", "cheated", "examiner", "excel", "lewdness", "liberal", "mathematician", "ordered", "president", "sandwich", "swagger", "swarm", "vomit", "yell", "zero" "zodiac", "zoo"};
vector<string> dictionary3 = {"ajar", "anachronism", "basin", "bawdy", "bleed", "bystander", "chariot", "cheap", "cheated", "clay", "contrive", "critiques", "
void add_word(vector<string>& dictionary, const string& word) {
dictionary.push_back(word);
sort(dictionary.begin(), dictionary.end());
}
void delete_word(vector<string>& dictionary, const string& word) {
dictionary.erase(remove(dictionary.begin(), dictionary.end(), word), dictionary.end());
}
int search_word(const vector<string>& dictionary, const string& word) {
for (size_t i = 0; i < dictionary.size(); ++i) {
if (dictionary[i] == word) {
return i;
}
}
return -1;
}
void swap_words(vector<string>& dictionary, const string& word1, const string& word2) {
int idx1 = search_word(dictionary, word1);
int idx2 = search_word(dictionary, word2);
if (idx1 != -1 && idx2 != -1) {
swap(dictionary[idx1], dictionary[idx2]);
}
}
int main() {
int dictionary_choice;
string search, del;
vector<vector<string>> dictionaries = {dictionary1, dictionary2, dictionary3};
cout << "Which Dictionary should be opened? Enter \"1\", \"2\", or \"3\": ";
cin >> dictionary_choice;
dictionary_choice--;
int idx, del_idx;
while (true) {
cout << "\n--------------------------------------------\n"
<< "Options menu: \n"
<< "(1) Print words\n"
<< "(2) Find a word\n"
<< "(3) Find word, insert if found (assumes words are sorted alphabetically)\n"
<< "(4) Find word, delete if found \n"
<< "(5) Swap two words\n"
<< "(6) Sort words (Bubble Sort or Selection Sort)\n"
<< "(7) Find a word - Binary Search (assumes words are sorted alphabetically)\n"
<< "(8) Merge two dictionaries (will sort first)\n"
<< "(9) Write current dictionary to file\n"
<< "Enter a number from 1 to 9, or 0 to exit: ";
int choice;
cin >> choice;



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

I wanted to put an example of one of the tests it was supposed to pass but there wasn't enough room. Can you adjust the code so the output is what the expected output is? I can't seem to do that without messing up the code even more.
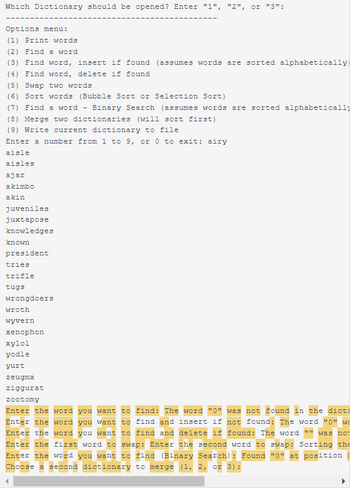
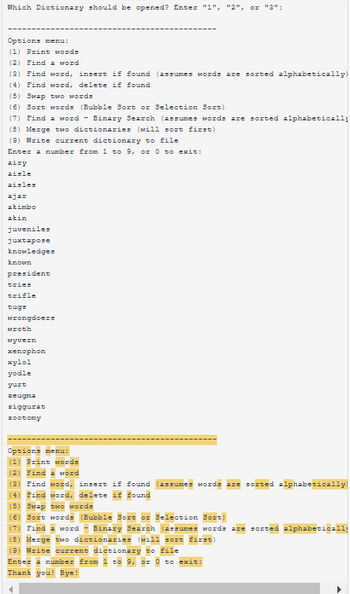
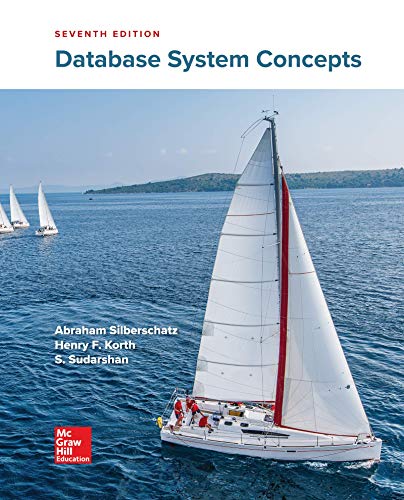
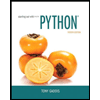
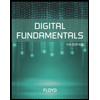
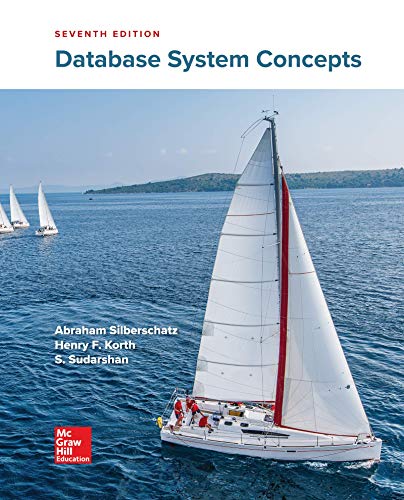
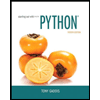
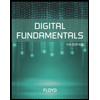
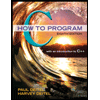
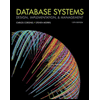
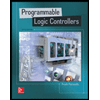