Finclude
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:### Code Analysis
**Objective:**
Determine if the provided C++ code will execute without any errors.
**Code:**
```cpp
#include <iostream>
using namespace std;
void copy (int& a, int& b, int& c)
{
a *= 2;
b *= 2;
c *= 2;
}
int main()
{
int x = 1, y = 3, z = 7;
copy(x, y, z);
cout << "x=" << x << ", y=" << y << ", z=" << z;
return 0;
}
```
**Explanation:**
1. **Includes and Namespaces:**
- The code begins with including the `iostream` library, necessary for input and output operations.
- The `using namespace std;` line allows the use of standard functions without prefixing them with `std::`.
2. **Function Definition:**
- The `copy` function takes three reference parameters (`int& a, int& b, int& c`), allowing it to modify the arguments passed to it.
- Inside the function, each argument is multiplied by 2 (`a *= 2; b *= 2; c *= 2;`).
3. **Main Function:**
- Three integers `x`, `y`, and `z` are initialized to 1, 3, and 7, respectively.
- The `copy` function is called with `x`, `y`, and `z` as arguments. Because these are passed by reference, their values will be modified by the function.
- After the function call, `cout` is used to print the modified values of `x`, `y`, and `z`.
4. **Output:**
- The expected output would be `x=2, y=6, z=14` since each variable is multiplied by 2 in the `copy` function.
**Conclusion:**
The code will run without any errors. The use of reference parameters in the `copy` function means the changes to `x`, `y`, and `z` are reflected outside the function, and the output will display the updated values.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
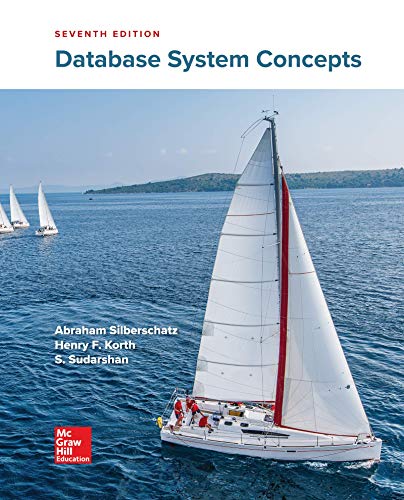
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
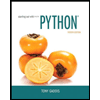
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
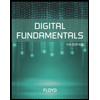
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
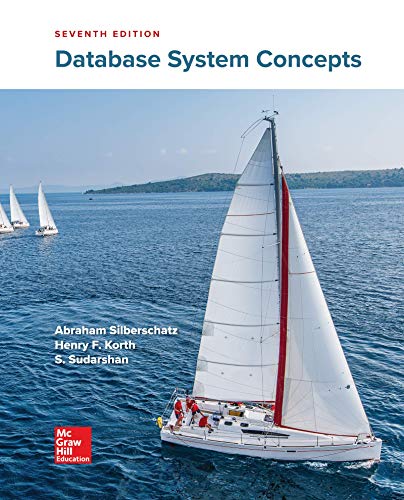
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
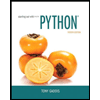
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
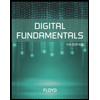
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
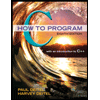
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
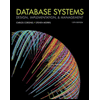
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
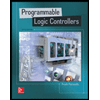
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education