Please write the code for the removeBad function. Will thumbs up if correct!
Please write the code for the removeBad function. Will thumbs up if correct!
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please write the code for the removeBad function. Will thumbs up if correct!
![```cpp
#include <list>
#include <vector>
#include <algorithm>
#include <iostream>
#include <cassert>
using namespace std;
vector<int> destroyedOnes;
class Movie
{
public:
Movie(int r) : m_rating(r) {
destroyedOnes.push_back(m_rating);
}
int rating() const { return m_rating; }
private:
int m_rating;
};
// Remove the movies in li with a rating below 50 and destroy them. It is acceptable
// if the order of the remaining movies is not the same as in the original list.
void removeBad(list<Movie*>& li)
{
}
void test() {
int a[8] = { 85, 80, 30, 70, 20, 15, 90, 10 };
list<Movie*> x;
for (int k = 0; k < 8; k++)
x.push_back(new Movie(a[k]));
assert(x.size() == 8 && x.front()->rating() == 85 && x.back()->rating() == 10);
removeBad(x);
assert(x.size() == 4 && destroyedOnes.size() == 4);
vector<int> v;
for (list<Movie*>::iterator p = x.begin(); p != x.end(); p++)
{
Movie* mp = *p;
v.push_back(mp->rating());
}
sort(v.begin(), v.end());
int expect[4] = { 70, 80, 85, 90 };
for (int k = 0; k < 4; k++)
assert(v[k] == expect[k]);
sort(destroyedOnes.begin(), destroyedOnes.end());
int expectGone[4] = { 10, 15, 20, 30 };
for (int k = 0; k < 4; k++)
assert(destroyedOnes[k] == expectGone[k]);
}
int main() {
test();
cout << "Passed" << endl;
return 0;
}
```
### Explanation of Code Structure:
1. **Includes and Namespace**:
- The code begins by including necessary libraries for list and vector manipulation, input/output operations, and assertions.
- The namespace `std` is used to avoid directly prefixing standard library types and functions.
2. **Global Vector `destroyedOnes](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2e51c4f9-7707-4851-92b0-3f7eb5342ba2%2F2e8f0a41-cd02-4015-a6f3-cdc4bfd0b50d%2Fholzukb_processed.png&w=3840&q=75)
Transcribed Image Text:```cpp
#include <list>
#include <vector>
#include <algorithm>
#include <iostream>
#include <cassert>
using namespace std;
vector<int> destroyedOnes;
class Movie
{
public:
Movie(int r) : m_rating(r) {
destroyedOnes.push_back(m_rating);
}
int rating() const { return m_rating; }
private:
int m_rating;
};
// Remove the movies in li with a rating below 50 and destroy them. It is acceptable
// if the order of the remaining movies is not the same as in the original list.
void removeBad(list<Movie*>& li)
{
}
void test() {
int a[8] = { 85, 80, 30, 70, 20, 15, 90, 10 };
list<Movie*> x;
for (int k = 0; k < 8; k++)
x.push_back(new Movie(a[k]));
assert(x.size() == 8 && x.front()->rating() == 85 && x.back()->rating() == 10);
removeBad(x);
assert(x.size() == 4 && destroyedOnes.size() == 4);
vector<int> v;
for (list<Movie*>::iterator p = x.begin(); p != x.end(); p++)
{
Movie* mp = *p;
v.push_back(mp->rating());
}
sort(v.begin(), v.end());
int expect[4] = { 70, 80, 85, 90 };
for (int k = 0; k < 4; k++)
assert(v[k] == expect[k]);
sort(destroyedOnes.begin(), destroyedOnes.end());
int expectGone[4] = { 10, 15, 20, 30 };
for (int k = 0; k < 4; k++)
assert(destroyedOnes[k] == expectGone[k]);
}
int main() {
test();
cout << "Passed" << endl;
return 0;
}
```
### Explanation of Code Structure:
1. **Includes and Namespace**:
- The code begins by including necessary libraries for list and vector manipulation, input/output operations, and assertions.
- The namespace `std` is used to avoid directly prefixing standard library types and functions.
2. **Global Vector `destroyedOnes
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
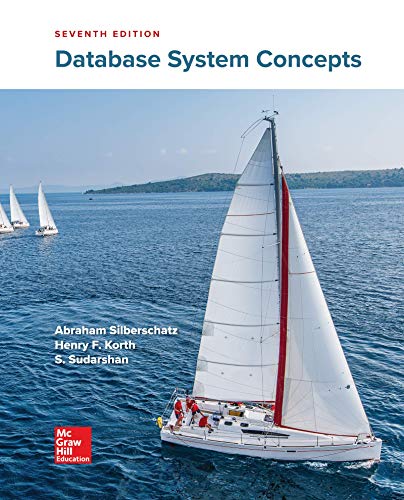
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
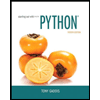
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
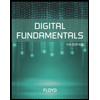
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
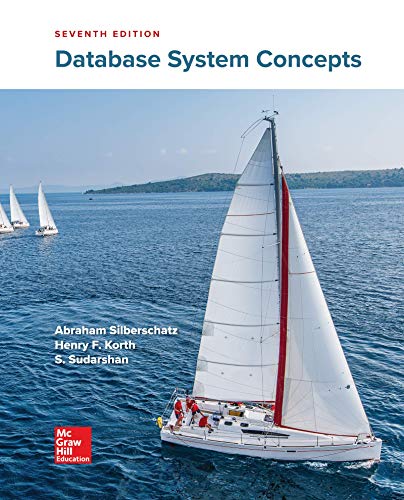
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
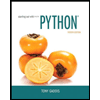
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
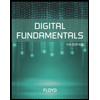
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
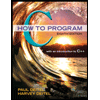
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
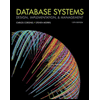
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
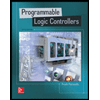
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education