Distinguish between the Java classes GenericServlet and HttpServlet using an example.
Q: Need help solving this issue. ShoppingList Class import java.util.Scanner; import…
A: There are some modifications required in the ShoppingList class. The changes are shown below:
Q: Using the picture, use Java to design and implement the class PascalTriangle that will generate a…
A: Algorithm: for (initialExpression; testExpression; updateExpression){ // body of the…
Q: private List tokens; private int currentTokenIndex; public Parser(List tokens) { this.tokens =…
A: here is a modified version of the Parser.java code that fixes some syntax errors and provides a…
Q: In java show, if possible, an example of a generic class that extends another class and implements…
A: A generic class is defined with the following format : class Name<T1, T2,....., Tn>{ /*. . .…
Q: Each row of the csv file contains the showtime, title, and rating of a movie. Assume data of the…
A: Solution: Given,
Q: Java Code: Below is Parser.java and there are errors. Look at the errors carefully and please fix…
A: There are some mistakes in the sample Java code provided for Parser.java that need to be fixed. It's…
Q: The Resident class maintains, for a set of addresses, the set of residents living at each address.…
A: addResident method has to first get the Set associated to that particular address and then add…
Q: Edit the following: package dao; import com.mongodb.client.MongoCollection; import…
A: Given code:- package dao; import com.mongodb.client.MongoCollection; import dto.BasePaymentDto;…
Q: 4.14 LAB: Ticketing service (Queue) Given main(), complete the program to add people to a queue. The…
A: Algorithm: START Initialize an empty queue called peopleInQueue. Prompt the user to enter a list…
Q: private Object expression(Node booleanCompare, HashMap variables2) { // TODO Auto-generated…
A: There are several errors in the provided Java code. Here is the corrected code in Step 2.
Q: Use java.util.Map, java.util.Set and given class Parition.java, write a Java method that receives a…
A: The program starts by importing the necessary java.util package.The Partition class is defined,…
Q: Input Your output Expected output Roxanne Hughes 443-555-2864 Juan Alberto Jr. 410-555-9385 Rachel…
A: Algorithm:Initialize head and current to null.For each of the three contacts:Prompt the user to…
Q: Complete the implementations of the abstract class LinkedList.java, which implements the List.java…
A: You are asked to create the implementations of LinkedList.java and LinkedOrderedList.java…
Q: Where are the test cases for interpreter.java? Write a test case for interpreter.java. For…
A: To write a test case for the Interpreter class, you'll need a testing framework like JUnit. In this…
Q: Draw a UML class diagram for the following code: import java.util.LinkedList; public class…
A: A UML class diagram is a way of looking at how classes are organized and how they relate to each…
Q: Implement the provided Queue interface ( fill out the implementation shell). Put your implementation…
A: Here's the implementation of the QueueImpl class: QueueImpl.java: package queue; import…
Q: Give an example of a program that uses the nongeneric version of a class from the Java Collection…
A: The Java Collections Framework is a set of classes and interfaces implementing complex collection…
Q: JAVA: Create generics class for validation and printing for the parking garage project
A: The code is given in the below step
Q: ShoppingList Class import java.util.Scanner; import java.util.LinkedList; public class…
A: Actually, java is a object oriented programming language. It is a platform independent.
Q: Implement a Doubly linked list to store a set of Integer numbers (no duplicate) Using Java •…
A: The items that make up a linked list are called nodes, and they are arranged in a linear data…
Q: import java.util.Scanner; public class SportsBus extends Bus { private int competitorArea;…
A: Below is the complete solution with explanation in detail for the given question about the…
Q: Java help!! Consider the generic singly linked list as defined in class via interface GenericList…
A: Generic implementation of singly linked list Program class EmptyListException extends…
Q: import java.text.DecimalFormat;import java.io.*;import java.util.*; public class Container {…
A: The question is asking to implement several classes in Java, namely Order, IOHandler, Container,…
Q: Consider class IntArrayStack that has two instance variables: int[] data and int top. Implement an…
A: As per our policy the programming language is not mentioned so i am providing the solution in Java.…
Q: import javax.swing.*; import java.awt.*; import java.util.List; import java.util.ArrayList; public…
A: In this question we have to write a Java code spread across multiple screenshots, which seemed to…
Q: In the given java code, below, help with the two methods. public boolean connected() and public…
A: The connected() method in the Graph class returns a boolean value indicating whether the graph is…
Q: write a program to implement the above interface using Lambda Expression to accept “ Hello World”…
A:
Q: Define a class StatePair with two generic types (Type1 and Type2), a constructor, mutators,…
A: Declare a generic class StatePair that takes two generic parameters Type1 and Type2, both of which…
Q: Explain how a RESTful service for the SimpleInterestCalculator may be created.
A: REST stands for Representative State Transfer. It is a software style developed by Roy Fielding in…
Q: Given main(), complete the program to add people to a queue. The program should read in a list of…
A: Answer :
Q: I need a test harness for the following code: LinkedListImpl.java package linkedList;…
A: The LinkedList class in Java is a part of the Java Collections Framework and provides a linked list…


Step by step
Solved in 2 steps with 1 images

- Given the code: import java.util.HashMap; import java.util.LinkedList; import java.util.Scanner; public class Controller { privateHashMap<String,LinkedList<Stock>>stockMap; publicController(){ stockMap=newHashMap<>(); Scannerinput=newScanner(System.in); do{ // Prompt for stock name or option to quit System.out.print("Enter stock name or 3 to quit: "); StringstockName=input.next(); if(stockName.equals("3")){ break;// Exit if user inputs '3' } // Get or create a list for the specified stock LinkedList<Stock>stockList=stockMap.computeIfAbsent(stockName,k->newLinkedList<>()); // Prompt to buy or sell System.out.print("Input 1 to buy, 2 to sell: "); intcontrolNum=input.nextInt(); System.out.print("How many stocks: "); intquantity=input.nextInt(); if(controlNum==1){ // Buying stocks System.out.print("At what price: "); doubleprice=input.nextDouble(); buyStock(stockList,stockName,quantity,price); }else{ // Selling stocks System.out.print("Press…Implement a generic priority queue in Java and test it with an abstract Cryptid class and two concrete Cryptid classes, Yeti and Bigfoot. Create a code package called pq and put all the code for this assignment in it. Write the abstract class Cryptid. A Cryptid has a name (a String) and a public abstract void attack() method. Name should be protected. The class implements Comparable<Cryptid>. The compareTo method returns a negative int if the instance Cryptid's name (that is, the name of the Cryptid you called the method on) is earlier in lexicographic order than the name of the other Cryptid (the one passed in as an argument; it returns a positive int if the other Cryptid's name is earlier in lex order, and returns 0 if they are equal (that is, if they have all the same characters.) This part is easy; just have the method call String's compareTo() method on the instance Cryptid's name, with the other Cryptid's name as the argument, and return the result. Extend Cryptid…write a program to implement the above interface using Lambda Expression to accept “ Hello World” and print it 10 times. write a program to implement the above interface using Inner Class to accept “ Hello World” and print it 10 times This is my code interface interf1 { publicvoidrepeat(Strings1); } public class Main { public static void main(String[] args) { interf1 obj = (s1)-> { //using lambda expression for(int i=0;i<10;i++) { System.out.println(s1); } }; obj.repeat("Hello World"); } } public class Assignment { public static void main(String[] args) { interf1 obj = new interf1() { //inner class public void repeat(String s1){ for(int i=0;i<10;i++){ System.out.println(s1); } } }; obj.repeat("Hello World"); //function call } } But i keep getting this error Exception in thread "main" java.lang.NoClassDefFoundError:…
- Write new Java class called Catalog so that it contains an ArrayList of Article objects.Implement comparator interface so that the catalog can be sorted by name or by volume.Given the following code in Java and attached images( containing related code): //Controller (ONLY EDIT THE Controller.java file) import java.util.LinkedList; import java.util.Scanner; public class Controller { public Controller() { LinkedList<Stock> googList = new LinkedList<Stock>(); LinkedList<Stock> amazList = new LinkedList<Stock>(); Scanner input = new Scanner(System.in); do { System.out.print("Enter 1 for Google stock or 2 for Amazon, 3 to quit: "); int stockSelect = input.nextInt(); if(stockSelect == 3) break; System.out.print("Input 1 to buy, 2 to sell: "); int controlNum = input.nextInt(); System.out.print("How many stocks: "); int quantity = input.nextInt(); if(controlNum == 1) { System.out.print("At what price: "); double price =…I'm trying to create a test harness for my LinkedList. I provided the code below. LinkedListImpl.java package linkedList; public abstract class LinkedListImpl implements LinkedList { private ListItem firstItem; public LinkedListImpl() { this.firstItem = null; } @Override public Boolean isItemInList(String thisItem) { ListItem currentItem = firstItem; while (currentItem != null) { if (currentItem.data.equals(thisItem)) { return true; } currentItem = currentItem.next; } return false; } @Override public Boolean addItem(String thisItem) { if (isItemInList(thisItem)) { return false; } else { ListItem newItem = new ListItem(thisItem); if (firstItem == null) { firstItem = newItem; } else { ListItem currentItem = firstItem; while (currentItem.next != null) { currentItem = currentItem.next; } currentItem.next = newItem; } return true; } } @Override public Integer itemCount() { Integer count = 0; ListItem currentItem = firstItem; while (currentItem != null) { count++; currentItem =…
- Use java Implement a phone book using a linked list structure. In this phone book , you are going to store a name,lastname,email,and phone number Implement the folowing methods by using this class public class PhoneBookSinglyLinkedList { public PhoneBookNode head; public PhoneBookNode tail; public int size; public boolean isEmpty() {//todo implement this} public int size() {//todo implement this} public void printPhoneBook() {//todo implement this} public void add(Contact contact) {//todo implement this} public PhoneBookNode findByFirstName(String firstName) {//todo implement this} public List findAllByLastName(String lastName) {//todo implement this} public void deleteByFirstName(String firstName) {//todo implement this} public void deleteAllMatchingLastName(String lastName) {//todo implement this} public List findAll() {//todo implement this}import java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…import java.util.HashMap; import java.util.Map; public class LinearSearchMap { // Define a method that takes in a map and a target value as parameters public static boolean linearSearch(Map<String, Integer> map, int target) { // Iterate through each entry in the map for () { // Check if the value of the current entry is equal to the target if () { // If the value is equal to the target, return true } } // If no entry with the target value is found, return false } public static void main(String[] args) { // Create a HashMap of strings and integers Map<String, Integer> numbers = new HashMap<>(); // Populate the HashMap with key-value pairs numbers.put("One", 1); numbers.put("Two", 2); numbers.put("Three", 3); numbers.put("Four", 4); numbers.put("Five", 5); // Set the target value to search for…
- The Java classes GenericServlet and HttpServlet may be differentiated from one another with the assistance of an example.in java please.Create a method from the implementation perspective. Create a method where it takes in a linked chain of values and adds them in order to the front. method header:public void addToFront(ANode<T> first)BuiltInFunctionDefinitionNode.java has an error so make sure to fix it. BuiltInFunctionDefinitionNode.java import java.util.HashMap; import java.util.function.Function; public class BuiltInFunctionDefinitionNode extends FunctionDefinitionNode { private Function<HashMap<String, InterpreterDataType>, String> execute; private boolean isVariadic; public BuiltInFunctionDefinitionNode(Function<HashMap<String, InterpreterDataType>, String> execute, boolean isVariadic) { this.execute = execute; this.isVariadic = isVariadic; } public String execute(HashMap<String, InterpreterDataType> parameters) { return this.execute.apply(parameters); } }
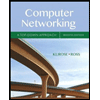
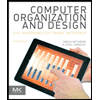
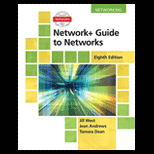
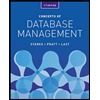
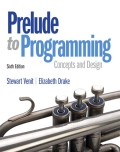
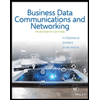
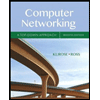
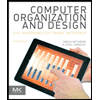
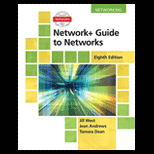
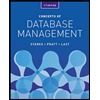
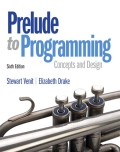
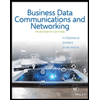