Define a class StatePair with two generic types (Type1 and Type2), a constructor, mutators, accessors, and a printInfo() method. Three ArrayLists have been pre-filled with StatePair data in main(): ArrayList> zipCodeState: Contains ZIP code/state abbreviation pairs ArrayList> abbrevState: Contains state abbreviation/state name pairs ArrayList> statePopulation: Contains state name/population pairs After a ZIP code is read into the program, complete main() to retrieve the correct state abbreviation from the ArrayList zipCodeState. Then use the state abbreviation to retrieve the state name from the ArrayList abbrevState. Lastly, use the state name to retrieve the correct state name/population pair from the ArrayList statePopulation and output the pair. Ex: If the input is: 21044 the output is: Maryland: 6079602 StatePopulations.java import java.util.Scanner; import java.io.FileInputStream; import java.io.IOException; import java.util.ArrayList; public class StatePopulations { public static ArrayList> fillArray1(ArrayList> statePairs, Scanner inFS) { StatePair pair; int intValue; String stringValue; while (inFS.hasNextLine()) { intValue = inFS.nextInt(); stringValue = inFS.next(); pair = new StatePair (intValue, stringValue); statePairs.add(pair); } return statePairs; } public static ArrayList> fillArray2(ArrayList> statePairs, Scanner inFS) { StatePair pair; String stringValue1; String stringValue2; while (inFS.hasNextLine()) { stringValue1 = inFS.next(); inFS.nextLine(); stringValue2 = inFS.nextLine(); pair = new StatePair (stringValue1, stringValue2); statePairs.add(pair); } return statePairs; } public static ArrayList> fillArray3(ArrayList> statePairs, Scanner inFS) { StatePair pair; String stringValue; int intValue; while (inFS.hasNextLine()) { stringValue = inFS.nextLine(); intValue = inFS.nextInt(); inFS.nextLine(); pair = new StatePair (stringValue, intValue); statePairs.add(pair); } return statePairs; } public static void main(String[] args) throws IOException { Scanner scnr = new Scanner(System.in); FileInputStream fileByteStream = null; // File input stream Scanner inFS = null; // Scanner object int myZipCode; int i; ArrayList> zipCodeState = new ArrayList>() ArrayList> abbrevState = new ArrayList>(); ArrayList> statePopulation = new ArrayList>(); // Fill the three ArrayLists // Try to open zip_code_state.txt file fileByteStream = new FileInputStream("zip_code_state.txt"); inFS = new Scanner(fileByteStream); zipCodeState = fillArray1(zipCodeState, inFS); fileByteStream.close(); // close() may throw IOException if fails // Try to open abbreviation_state.txt file fileByteStream = new FileInputStream("abbreviation_state.txt"); inFS = new Scanner(fileByteStream); abbrevState = fillArray2(abbrevState, inFS); fileByteStream.close(); // close() may throw IOException if fails // Try to open state_population.txt file fileByteStream = new FileInputStream("state_population.txt"); inFS = new Scanner(fileByteStream); statePopulation = fillArray3(statePopulation, inFS); fileByteStream.close(); // close() may throw IOException if fails // Read in ZIP code from user myZipCode = scnr.nextInt(); for (i = 0; i < zipCodeState.size(); ++i) { // TODO: Using ZIP code, find state abbreviation } for (i = 0; i < abbrevState.size(); ++i) { // TODO: Using state abbreviation, find state name } for (i = 0; i < statePopulation.size(); ++i) { // TODO: Using state name, find population. Print pair info. } } } StatePair.java public class StatePair , Type2 extends Comparable> { private Type1 value1; private Type2 value2; // TODO: Define a constructor, mutators, and accessors // for StatePair // TODO: Define printInfo() method }
Define a class StatePair with two generic types (Type1 and Type2), a constructor, mutators, accessors, and a printInfo() method. Three ArrayLists have been pre-filled with StatePair data in main():
- ArrayList<StatePair<Integer, String>> zipCodeState: Contains ZIP code/state abbreviation pairs
- ArrayList<StatePair<String, String>> abbrevState: Contains state abbreviation/state name pairs
- ArrayList<StatePair<String, Integer>> statePopulation: Contains state name/population pairs
After a ZIP code is read into the program, complete main() to retrieve the correct state abbreviation from the ArrayList zipCodeState. Then use the state abbreviation to retrieve the state name from the ArrayList abbrevState. Lastly, use the state name to retrieve the correct state name/population pair from the ArrayList statePopulation and output the pair.
Ex: If the input is: 21044
the output is: Maryland: 6079602
StatePopulations.java
import java.util.Scanner;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.ArrayList;
public class StatePopulations {
public static ArrayList<StatePair<Integer, String>> fillArray1(ArrayList<StatePair<Integer, String>> statePairs,
Scanner inFS) {
StatePair<Integer, String> pair;
int intValue;
String stringValue;
while (inFS.hasNextLine()) {
intValue = inFS.nextInt();
stringValue = inFS.next();
pair = new StatePair<Integer, String> (intValue, stringValue);
statePairs.add(pair);
}
return statePairs;
}
public static ArrayList<StatePair<String, String>> fillArray2(ArrayList<StatePair<String, String>> statePairs,
Scanner inFS) {
StatePair<String, String> pair;
String stringValue1;
String stringValue2;
while (inFS.hasNextLine()) {
stringValue1 = inFS.next();
inFS.nextLine();
stringValue2 = inFS.nextLine();
pair = new StatePair<String, String> (stringValue1, stringValue2);
statePairs.add(pair);
}
return statePairs;
}
public static ArrayList<StatePair<String, Integer>> fillArray3(ArrayList<StatePair<String, Integer>> statePairs,
Scanner inFS) {
StatePair<String, Integer> pair;
String stringValue;
int intValue;
while (inFS.hasNextLine()) {
stringValue = inFS.nextLine();
intValue = inFS.nextInt();
inFS.nextLine();
pair = new StatePair<String, Integer> (stringValue, intValue);
statePairs.add(pair);
}
return statePairs;
}
public static void main(String[] args) throws IOException {
Scanner scnr = new Scanner(System.in);
FileInputStream fileByteStream = null; // File input stream
Scanner inFS = null; // Scanner object
int myZipCode;
int i;
ArrayList<StatePair<Integer, String>> zipCodeState = new ArrayList<StatePair<Integer, String>>()
ArrayList<StatePair<String, String>> abbrevState = new ArrayList<StatePair<String, String>>();
ArrayList<StatePair<String, Integer>> statePopulation = new ArrayList<StatePair<String, Integer>>();
// Fill the three ArrayLists
// Try to open zip_code_state.txt file
fileByteStream = new FileInputStream("zip_code_state.txt");
inFS = new Scanner(fileByteStream);
zipCodeState = fillArray1(zipCodeState, inFS);
fileByteStream.close(); // close() may throw IOException if fails
// Try to open abbreviation_state.txt file
fileByteStream = new FileInputStream("abbreviation_state.txt");
inFS = new Scanner(fileByteStream);
abbrevState = fillArray2(abbrevState, inFS);
fileByteStream.close(); // close() may throw IOException if fails
// Try to open state_population.txt file
fileByteStream = new FileInputStream("state_population.txt");
inFS = new Scanner(fileByteStream);
statePopulation = fillArray3(statePopulation, inFS);
fileByteStream.close(); // close() may throw IOException if fails
// Read in ZIP code from user
myZipCode = scnr.nextInt();
for (i = 0; i < zipCodeState.size(); ++i) {
// TODO: Using ZIP code, find state abbreviation
}
for (i = 0; i < abbrevState.size(); ++i) {
// TODO: Using state abbreviation, find state name
}
for (i = 0; i < statePopulation.size(); ++i) {
// TODO: Using state name, find population. Print pair info.
}
}
}
StatePair.java
public class StatePair <Type1 extends Comparable<Type1>, Type2 extends Comparable<Type2>> {
private Type1 value1;
private Type2 value2;
// TODO: Define a constructor, mutators, and accessors
// for StatePair
// TODO: Define printInfo() method
}

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 5 images

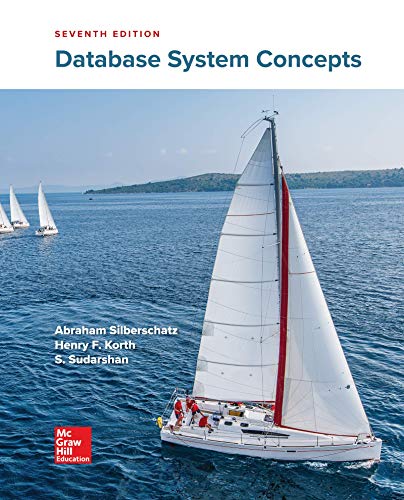
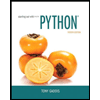
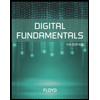
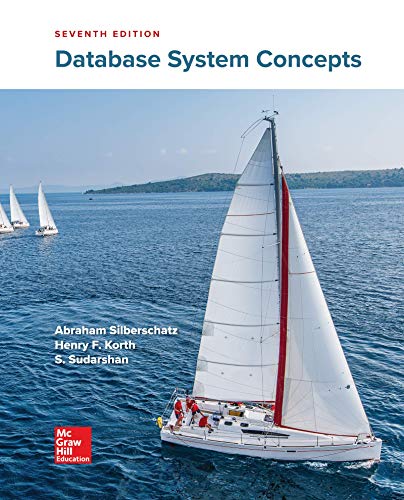
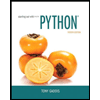
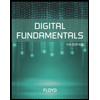
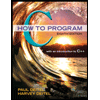
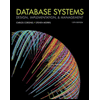
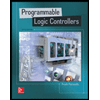