I'm trying to create a test harness for my LinkedList. I provided the code below. LinkedListImpl.java package linkedList; public abstract class LinkedListImpl implements LinkedList { private ListItem firstItem; public LinkedListImpl() { this.firstItem = null; } @Override public Boolean isItemInList(String thisItem) { ListItem currentItem = firstItem; while (currentItem != null) { if (currentItem.data.equals(thisItem)) { return true; } currentItem = currentItem.next; } return false; } @Override public Boolean addItem(String thisItem) { if (isItemInList(thisItem)) { return false; } else { ListItem newItem = new ListItem(thisItem); if (firstItem == null) { firstItem = newItem; } else { ListItem currentItem = firstItem; while (currentItem.next != null) { currentItem = currentItem.next; } currentItem.next = newItem; } return true; } } @Override public Integer itemCount() { Integer count = 0; ListItem currentItem = firstItem; while (currentItem != null) { count++; currentItem = currentItem.next; } return count; } @Override public void listItems() { if (firstItem == null) { System.out.println("The list is empty."); } else { ListItem currentItem = firstItem; while (currentItem != null) { System.out.println(currentItem.data); currentItem = currentItem.next; } } } @Override public Boolean deleteItem(String thisItem) { if (firstItem == null) { return false; } else if (firstItem.data.equals(thisItem)) { firstItem = firstItem.next; return true; } else { ListItem currentItem = firstItem; while (currentItem.next != null) { if (currentItem.next.data.equals(thisItem)) { currentItem.next = currentItem.next.next; return true; } currentItem = currentItem.next; } return false; } } @Override public Boolean insertBefore(String newItem, String itemToInsertBefore) { if (firstItem == null) { return false; } else if (firstItem.data.equals(itemToInsertBefore)) { ListItem newItemNode = new ListItem(newItem); newItemNode.next = firstItem; firstItem = newItemNode; return true; } else { ListItem currentItem = firstItem; while (currentItem.next != null) { if (currentItem.next.data.equals(itemToInsertBefore)) { ListItem newItemNode = new ListItem(newItem); newItemNode.next = currentItem.next; currentItem.next = newItemNode; return true; } currentItem = currentItem.next; } return false; } } } LinkedListTest.java package linkedList; public class LinkedListTester { public static void main(String[] args) { } }
I'm trying to create a test harness for my LinkedList. I provided the code below.
LinkedListImpl.java
package linkedList;
public abstract class LinkedListImpl implements LinkedList {
private ListItem firstItem;
public LinkedListImpl() {
this.firstItem = null;
}
@Override
public Boolean isItemInList(String thisItem) {
ListItem currentItem = firstItem;
while (currentItem != null) {
if (currentItem.data.equals(thisItem)) {
return true;
}
currentItem = currentItem.next;
}
return false;
}
@Override
public Boolean addItem(String thisItem) {
if (isItemInList(thisItem)) {
return false;
} else {
ListItem newItem = new ListItem(thisItem);
if (firstItem == null) {
firstItem = newItem;
} else {
ListItem currentItem = firstItem;
while (currentItem.next != null) {
currentItem = currentItem.next;
}
currentItem.next = newItem;
}
return true;
}
}
@Override
public Integer itemCount() {
Integer count = 0;
ListItem currentItem = firstItem;
while (currentItem != null) {
count++;
currentItem = currentItem.next;
}
return count;
}
@Override
public void listItems() {
if (firstItem == null) {
System.out.println("The list is empty.");
} else {
ListItem currentItem = firstItem;
while (currentItem != null) {
System.out.println(currentItem.data);
currentItem = currentItem.next;
}
}
}
@Override
public Boolean deleteItem(String thisItem) {
if (firstItem == null) {
return false;
} else if (firstItem.data.equals(thisItem)) {
firstItem = firstItem.next;
return true;
} else {
ListItem currentItem = firstItem;
while (currentItem.next != null) {
if (currentItem.next.data.equals(thisItem)) {
currentItem.next = currentItem.next.next;
return true;
}
currentItem = currentItem.next;
}
return false;
}
}
@Override
public Boolean insertBefore(String newItem, String itemToInsertBefore) {
if (firstItem == null) {
return false;
} else if (firstItem.data.equals(itemToInsertBefore)) {
ListItem newItemNode = new ListItem(newItem);
newItemNode.next = firstItem;
firstItem = newItemNode;
return true;
} else {
ListItem currentItem = firstItem;
while (currentItem.next != null) {
if (currentItem.next.data.equals(itemToInsertBefore)) {
ListItem newItemNode = new ListItem(newItem);
newItemNode.next = currentItem.next;
currentItem.next = newItemNode;
return true;
}
currentItem = currentItem.next;
}
return false;
}
}
}
LinkedListTest.java
package linkedList;
public class LinkedListTester {
public static void main(String[] args) {
}
}

Step by step
Solved in 3 steps

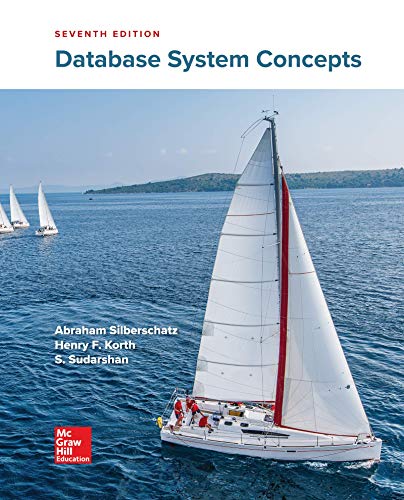
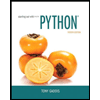
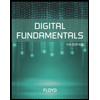
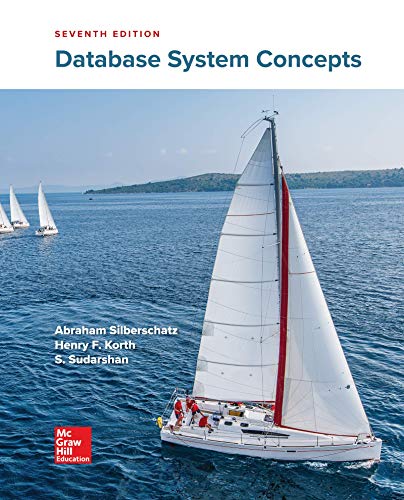
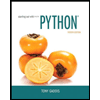
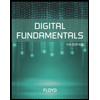
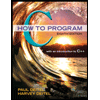
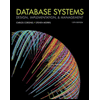
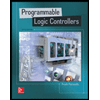