Given an interface for LinkedList - Without using the java collections interface (ie do not import java.util.List, LinkedList, Stack, Queue...) - Create an implementation of LinkedList interface provided - For the implementation create a tester to verify the implementation
Simple linkedlist implementation
please help (looks like a lot but actually isnt, everything is already set up for you below to make it EVEN easier)
help in any part you can be clear
Given an interface for LinkedList
- Without using the java collections interface (ie do not import java.util.List,
LinkedList, Stack, Queue...)
- Create an implementation of LinkedList interface provided
- For the implementation create a tester to verify the implementation of that
data structure performs as expected
Build Bus Route – Linked List
- You’ll be provided a java package containing an interface, data structure,
implementation shell, test harness shell below.
- Your task is to:
o Implement the LinkedList interface (fill out the implementation shell)
o Put your implementation through its paces by exercising each of the
methods in the test harness
o Create a client (a class with a main) ‘BusClient’ which builds a bus
route by performing the following operations on your linked list:
o
§ Create (insert) 4 stations
§ List the stations
§ Check if a station is in the list (print result)
• Check for a station that exists, and one
that doesn’t
§ Remove a station
§ List the stations
§ Add a station before another station
§ List the stations
§ Add a station after another station
§ Print the stations
5 CLASSES TO BEGIN WITH BELOW (edit these classes to fulfill the easy requirements above)
import linkedList.LinkedList;
import linkedList.LinkedListImpl;
import queue.Queue;
import queue.QueueImpl;
import stack.Stack;
import stack.StackImpl;
public class BusClient {
public static void main(String[] args) {
// create implementation, then
System.out.println("-----L I S T T E S T------");
//listRunTestMethod...
}
}
----------
public interface LinkedList {
public Boolean isItemInList(String thisItem);
// true if it is, false if not
public Boolean addItem(String thisItem);
// true if added, false if it was already there, or an error
public Integer itemCount();
public void listItems();
public Boolean deleteItem(String thisItem);
// true if deleted, false if not there or error
public Boolean insertBefore(String newItem, String itemToInsertBefore);
public Boolean insertAfter(String newItem, String itemToInsertAfter);
//EC
public void sort();
// ascending alphanumeric sort; nothing fancy but ALTERS THE LIST, DOES NOT COPY.
}
// Implement this interface using class ListItem
// Also implement the tester in this package.
----------
public class LinkedListImpl implements LinkedList {
private ListItem head;
@Override
public Boolean isItemInList(String thisItem) {
// TODO Auto-generated method stub
return null;
}
@Override
public Boolean addItem(String thisItem) {
//get the last item:
ListItem lastItem = head;
if (head == null)
lastItem = null;
else {
lastItem = head;
//last node points to null
while (lastItem.next != null)
lastItem = lastItem.next;
}
// create a ListItem, if head is null, set the newItem
// to be the head
// else, set the head.next to the newItem
ListItem newItem = new ListItem(thisItem);
if (head == null) {
head = newItem;
} else {
//head.next = newItem;
lastItem.next = newItem;
}
return true;
}
@Override
public Integer itemCount() {
// TODO Auto-generated method stub
return null;
}
@Override
public void listItems() {
ListItem tmp = head;
while(tmp != null) {
System.out.println("current data: " + tmp.data);
tmp = tmp.next;
}
}
@Override
public Boolean deleteItem(String thisItem) {
// TODO Auto-generated method stub
return null;
}
@Override
public Boolean insertBefore(String newItem, String itemToInsertBefore) {
// TODO Auto-generated method stub
return null;
}
@Override
public Boolean insertAfter(String newItem, String itemToInsertAfter) {
// TODO Auto-generated method stub
return null;
}
@Override
public void sort() {
// TODO Auto-generated method stub
}
}
----------
public class LinkedListTester {
public static void main(String[] args) {
LinkedList l = new LinkedListImpl();
l.addItem("bearded dragon");
l.addItem("iguana");
l.addItem("turtle");
l.listItems();
}
}
----------
public class ListItem {
public String data;
public ListItem next;
public ListItem(String data) {
this.data = data;
this.next = null;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

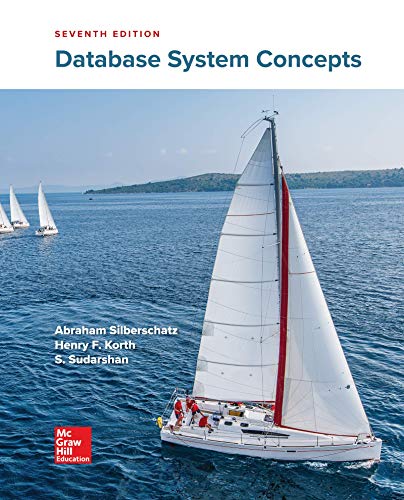
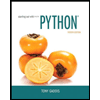
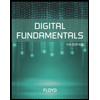
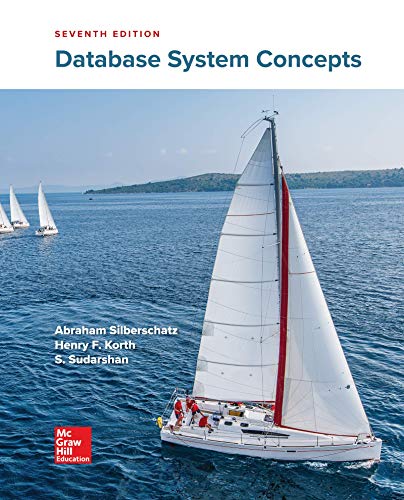
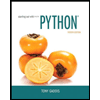
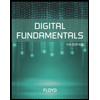
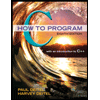
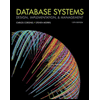
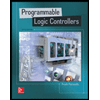