Implement a Doubly linked list to store a set of Integer numbers (no duplicate) Using Java • Instance variable • Constructor • Accessor and Update methods 1. Define a Node Class. a. Instance Variables # E element - (generics framework) # Node next (pointer) - refer to the next node (Self-referential) # Node prev (pointer) - refer to the previous node (Self-referential) b. Constructor c. Methods # E getElement() //Return the value of this node. # setElement(E e) // Set value to this node. # Node getNext() //Return the next pointer of this node. # setNext(Node n) //Set the next pointer to this node. # Node getPrev() //Return the pointer of this node. # setPrev(Node n) //Set the previous pointer to this node. # displayNode() //Display the value of this node.
Implement a Doubly linked list to store a set of Integer numbers (no duplicate) Using Java
• Instance variable
• Constructor
• Accessor and Update methods
1. Define a Node Class.
a. Instance Variables
# E element - (generics framework)
# Node next (pointer) - refer to the next node (Self-referential)
# Node prev (pointer) - refer to the previous node (Self-referential)
b. Constructor
c. Methods
# E getElement() //Return the value of this node.
# setElement(E e) // Set value to this node.
# Node getNext() //Return the next pointer of this node.
# setNext(Node n) //Set the next pointer to this node.
# Node getPrev() //Return the pointer of this node.
# setPrev(Node n) //Set the previous pointer to this node.
# displayNode() //Display the value of this node.

Step by step
Solved in 4 steps with 1 images

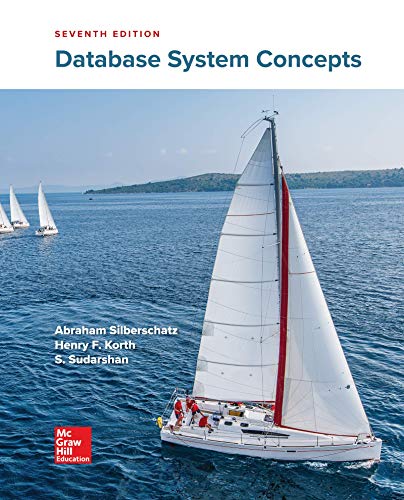
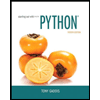
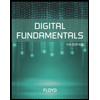
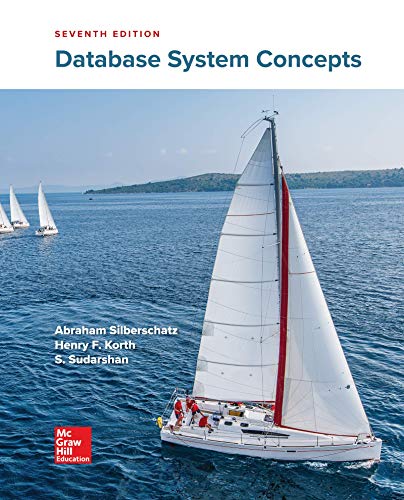
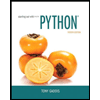
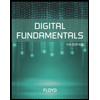
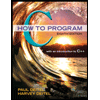
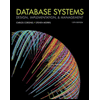
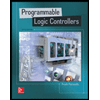