Structure of the MyBSTree Fields • a private field named root of type Node You may implement any additional fields that you may need. Structure of the MyBSTree Methods As described by the UML Class Diagram above, your MyBSTree class must implement the following methods: • a public method named insert that takes an object of type T as an argument and returns nothing • a public method named contians Item that takes an object of type T as an argument and return a boolean • a public method named getSize that takes no arguments and returns an int • a public method named print InOrder that takes no arguments and returns nothing • a public method named toString that takes no arguments and returns a String Note that these methods are declared in the ITree generic interface. You will be implementing these methods in this MyBSTree concrete class. You may implement any additional methods that you may need. You will also need to implement a nested inner class named Node inside of your MyBSTree class. Each Node object will store one piece of data in the binary search tree. The actual data value will be stored in the data field of the Node object. As these are binary Node objects, each Node will also store a reference to a left sub-node and a right sub-node. These references will be stored in the left and right fields. Structure of the Node Fields • a public field named data of type T • a public field named left of type Node • a public field named right of type Node You may implement any additional fields that you may need. Structure of the Node Methods • a public constructor that takes an argument of type T • a public method named insert that takes an argument of type T and returns nothing You may implement any additional methods that you may need. Additional Information MyBSTree 1. This concrete class will store its elements in a collection of linked binary Node objects. 2. insert method o Inserts a new item into the binary search tree in the correct location. • There should be no duplicate items in the tree. If an item is inserted and that item is already in the tree then this method should simply return without changing the state of the tree. 3. containsItem method o Returns true if the tree contains the specified item; otherwise returns false. 4. getSize method o Returns the number of nodes currently stored in this tree. 5. printInOrder method o Prints the items in the tree in a space separated list in ascending order. 6. toString method • Returns a String containing the items in the tree in ascending order and separated by a space. Node 1. Your BSTree class must contain a nested inner class named Node. This class must be declared to be package level (not private or public). 2. parameterized constructor method o initializes the data of the new Node with the argument value. 3. insert method o this is a recursive method that finds the insertion point and inserts a Node for the new item in the correct position in the sub- tree for which this Node is the root. Remember that no duplicate items can be stored in the tree.
Structure of the MyBSTree Fields • a private field named root of type Node You may implement any additional fields that you may need. Structure of the MyBSTree Methods As described by the UML Class Diagram above, your MyBSTree class must implement the following methods: • a public method named insert that takes an object of type T as an argument and returns nothing • a public method named contians Item that takes an object of type T as an argument and return a boolean • a public method named getSize that takes no arguments and returns an int • a public method named print InOrder that takes no arguments and returns nothing • a public method named toString that takes no arguments and returns a String Note that these methods are declared in the ITree generic interface. You will be implementing these methods in this MyBSTree concrete class. You may implement any additional methods that you may need. You will also need to implement a nested inner class named Node inside of your MyBSTree class. Each Node object will store one piece of data in the binary search tree. The actual data value will be stored in the data field of the Node object. As these are binary Node objects, each Node will also store a reference to a left sub-node and a right sub-node. These references will be stored in the left and right fields. Structure of the Node Fields • a public field named data of type T • a public field named left of type Node • a public field named right of type Node You may implement any additional fields that you may need. Structure of the Node Methods • a public constructor that takes an argument of type T • a public method named insert that takes an argument of type T and returns nothing You may implement any additional methods that you may need. Additional Information MyBSTree 1. This concrete class will store its elements in a collection of linked binary Node objects. 2. insert method o Inserts a new item into the binary search tree in the correct location. • There should be no duplicate items in the tree. If an item is inserted and that item is already in the tree then this method should simply return without changing the state of the tree. 3. containsItem method o Returns true if the tree contains the specified item; otherwise returns false. 4. getSize method o Returns the number of nodes currently stored in this tree. 5. printInOrder method o Prints the items in the tree in a space separated list in ascending order. 6. toString method • Returns a String containing the items in the tree in ascending order and separated by a space. Node 1. Your BSTree class must contain a nested inner class named Node. This class must be declared to be package level (not private or public). 2. parameterized constructor method o initializes the data of the new Node with the argument value. 3. insert method o this is a recursive method that finds the insertion point and inserts a Node for the new item in the correct position in the sub- tree for which this Node is the root. Remember that no duplicate items can be stored in the tree.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
***Please help with the code in java for MyBSTree and Main. I cannot use java.util for this assignment****

Transcribed Image Text:Structure of the MyBSTree Fields
• a private field named root of type Node
You may implement any additional fields that you may need.
Structure of the MyBSTree Methods
As described by the UML Class Diagram above, your MyBSTree class must implement the following methods:
• a public method named insert that takes an object of type T as an argument and returns nothing
• a public method named contians Item that takes an object of type T as an argument and return a boolean
• a public method named getSize that takes no arguments and returns an int
• a public method named print InOrder that takes no arguments and returns nothing
• a public method named toString that takes no arguments and returns a String
Note that these methods are declared in the ITree generic interface. You will be implementing these methods in this MyBSTree
concrete class.
You may implement any additional methods that you may need.
You will also need to implement a nested inner class named Node inside of your MyBSTree class. Each Node object will store one piece of
data in the binary search tree. The actual data value will be stored in the data field of the Node object. As these are binary Node objects,
each Node will also store a reference to a left sub-node and a right sub-node. These references will be stored in the left and right fields.
Structure of the Node Fields
•
a public field named data of type T
• a public field named left of type Node
• a public field named right of type Node
You may implement any additional fields that you may need.
Structure of the Node Methods
• a public constructor that takes an argument of type T
• a public method named insert that takes an argument of type T and returns nothing
You may implement any additional methods that you may need.
Additional Information
MyBSTree
1. This concrete class will store its elements in a collection of linked binary Node objects.
2. insert method
o Inserts a new item into the binary search tree in the correct location.
o There should be no duplicate items in the tree. If an item is inserted and that item is already in the tree then this method should
simply return without changing the state of the tree.
3. contains Item method
• Returns true if the tree contains the specified item; otherwise returns false.
4. getSize method
• Returns the number of nodes currently stored in this tree.
5. printInOrder method
o Prints the items in the tree in a space separated list in ascending order.
6. toString method
• Returns a String containing the items in the tree in ascending order and separated by a space.
Node
1. Your BSTree class must contain a nested inner class named Node. This class must be declared to be package level (not private or
public).
2. parameterized constructor method
o initializes the data of the new Node with the argument value.
3. insert method
o this is a recursive method that finds the insertion point and inserts a Node for the new item in the correct position in the sub-
tree for which this Node is the root. Remember that no duplicate items can be stored in the tree.

Transcribed Image Text:• Write concrete classes that implement Java Interfaces according to specifications given in UML.
implement a nested inner class in Java.
• Implement a generic type in Java.
• Implement the functionality of a Binary Search tree data structure.
• Write a recursive method.
• Write a recursive search method for a Binary Search Tree.
• Write a recursive insert method for a Binary Search Tree.
• Write code to perform an in-order traversal of a Binary Search Tree.
You are not allowed to use any of the standard Java collection types for this assignment. Do not import any Java standard library
features from java.util.
Problem Description and Given Info
For this assignment you are given the following Java source code files:
• ITree.java (This file is complete - make no changes to this file)
• MyBSTree.java (You must complete this file)
• Main.java (You may use this file to write code to test your MyBSTree)
You must complete the public class named MyBSTree.java with fields and methods as defined below.
MyBSTree is a Java generic type. In the info given below, the identifier T denotes this generic type.
UML
ITree<T> <<interface>>
+ insert(item : T): void
+ contiansItem(item : T): boolean
+ getSize(): int
+ printlnOrder(): void
+ toString(): String
MyBSTree<T>
- root : Node
implements
Node <<nested inner class>>
+ data : T
+ left : Node
+ right: Node
+ Node(data: T)
+ insert(item : T)
UML CLass Diagram: MyBSTree
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
I have tried the code and get many errors:
![Failed to compile
MyBSTree.java:1: error: type argument T#1 is not within bounds of type-variable T#2
public class MyBSTree<T extends Comparable<? super T>> implements ITree<T> {
where T#1, T#2 are type-variables:
T#1 extends Comparable<? super T#1> declared in class MyBSTree
T#2 extends Comparable<T#2> declared in interface ITree
MyBSTree.java:18: warning: [rawtypes] found raw type: Node
this.left = new Node (item);
missing type arguments for generic class Node<T>
where T is a type-variable:
T extends Comparable<? super T> declared in class Node
MyBSTree.java:18: warning: [unchecked] unchecked call to Node (T) as
this.left = new Node (item);
where T is a type-variable:
T extends Comparable<? super T> declared in class Node
MyBSTree.java:18: warning: [unchecked] unchecked conversion
this.left = new Node (item);
required: Node<T>
found:
Node
where T is a type-variable:
T extends Comparable<? super T> declared in class Node
MyBSTree.java:20: warning: [rawtypes] found raw type: Node
this.right = new Node (item);
missing type arguments for generic class Node<T>
where T is a type-variable:
T extends Comparable<? super T> declared in class Node
MyBSTree.java:20: warning: [unchecked] unchecked call to Node (T) as a member of the raw type
this.right = new Node (item);
where T is a type-variable:
T extends Comparable<? super T> declared in class Node
MyBSTree.java:20: warning: [unchecked] unchecked conversion
this.right = new Node (item);
required: Node<T>
found:
Node
where T is a type-variable:
T extends Comparable<? super T> declared in class Node
MyBSTree.java:31: warning: [rawtypes] found raw type: Node
root = new Node (item);
missing type arguments for generic class Node<T>
where T is a type-variable:
T extends Comparable<? super T> declared in class Node
MyBSTree.java:31: warning: [unchecked] unchecked call to Node (T)
root = new Node (item);
where T is a type-variable:
T extends Comparable<? super T> declared in class Node
MyBSTree.java:31: warning: [unchecked] unchecked conversion
root = new Node (item);
a member of the raw type
required: Node<T>
found:
Node
where T is a type-variable:
T extends Comparable<? super T> declared in class MyBSTree
MyBSTree.java:33: warning: [rawtypes] found raw type: Node
Node temp = root, prev = null;
as a member of the raw type](https://content.bartleby.com/qna-images/question/1e26a685-55e2-4925-ba70-9f39a5b1116e/51d08876-706e-418a-9629-5d46558ad263/pt4rny2_thumbnail.png)
Transcribed Image Text:Failed to compile
MyBSTree.java:1: error: type argument T#1 is not within bounds of type-variable T#2
public class MyBSTree<T extends Comparable<? super T>> implements ITree<T> {
where T#1, T#2 are type-variables:
T#1 extends Comparable<? super T#1> declared in class MyBSTree
T#2 extends Comparable<T#2> declared in interface ITree
MyBSTree.java:18: warning: [rawtypes] found raw type: Node
this.left = new Node (item);
missing type arguments for generic class Node<T>
where T is a type-variable:
T extends Comparable<? super T> declared in class Node
MyBSTree.java:18: warning: [unchecked] unchecked call to Node (T) as
this.left = new Node (item);
where T is a type-variable:
T extends Comparable<? super T> declared in class Node
MyBSTree.java:18: warning: [unchecked] unchecked conversion
this.left = new Node (item);
required: Node<T>
found:
Node
where T is a type-variable:
T extends Comparable<? super T> declared in class Node
MyBSTree.java:20: warning: [rawtypes] found raw type: Node
this.right = new Node (item);
missing type arguments for generic class Node<T>
where T is a type-variable:
T extends Comparable<? super T> declared in class Node
MyBSTree.java:20: warning: [unchecked] unchecked call to Node (T) as a member of the raw type
this.right = new Node (item);
where T is a type-variable:
T extends Comparable<? super T> declared in class Node
MyBSTree.java:20: warning: [unchecked] unchecked conversion
this.right = new Node (item);
required: Node<T>
found:
Node
where T is a type-variable:
T extends Comparable<? super T> declared in class Node
MyBSTree.java:31: warning: [rawtypes] found raw type: Node
root = new Node (item);
missing type arguments for generic class Node<T>
where T is a type-variable:
T extends Comparable<? super T> declared in class Node
MyBSTree.java:31: warning: [unchecked] unchecked call to Node (T)
root = new Node (item);
where T is a type-variable:
T extends Comparable<? super T> declared in class Node
MyBSTree.java:31: warning: [unchecked] unchecked conversion
root = new Node (item);
a member of the raw type
required: Node<T>
found:
Node
where T is a type-variable:
T extends Comparable<? super T> declared in class MyBSTree
MyBSTree.java:33: warning: [rawtypes] found raw type: Node
Node temp = root, prev = null;
as a member of the raw type
![where T is a type-variable:
T extends Object declared in interface Comparable
MyBSTree.java:54: warning: [unchecked] unchecked call to compareTo (T) as
else if (temp.data.compareTo (item) < 0)
where T is a type-variable:
T extends Object declared in interface Comparable
Main.java:3: warning: [rawtypes] found raw type: ITree
ITree tree = new MyBSTree ();
missing type arguments for generic class ITree<T>
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
Main.java:3: warning: [rawtypes] found raw type: MyBSTree
ITree tree = new MyBSTree ();
missing type arguments for generic class MyBSTree<T>
where T is a type-variable:
T extends Comparable<? super T> declared in class MyBSTree
Main.java:4: warning: [unchecked] unchecked call to contains Item (T) as a member of the raw t
System.out.println (tree.containsItem (1)); // empty tree, 1 not present
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
Main.java:5: warning: [unchecked] unchecked call to insert (T) as a member of the raw type IT
tree.insert (2);
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
Main.java:6: warning: [unchecked] unchecked call to contains Item (T) as a member of the raw t
System.out.println (tree.contains Item (1)); // tree [2], 1 not present
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
Main.java:7: warning: [unchecked] unchecked call to insert (T) as a member of the raw type IT
tree.insert (1);
a member of the raw
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
Main.java:8: warning: [unchecked] unchecked call to contains Item (T) as a member of the raw t
System.out.println (tree.contains Item(1)); // tree [1, 2] 1 is present
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
Main.java:9: warning: [unchecked] unchecked call to insert (T) as a member of the raw type IT
tree.insert (5);
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
Main.java:10: warning: [unchecked] unchecked call to containsItem (T) as a member of the raw
System.out.println (tree.contains Item (4)); // tree [1, 2, 5] 4 is not present
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
Main.java:11: warning: [unchecked] unchecked call to insert (T) as a member of the raw type I
tree.insert (3);
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
Main.java:12: warning: [unchecked] unchecked call to containsItem (T) as a member of the raw
System.out.println (tree.contains Item (4)); // tree [1, 2, 3, 5] 4 is not present
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
Main.java:14: warning: [unchecked] unchecked call to insert (T) as a member of the raw type I
tree.insert (5);
// tree [1, 2, 3, 5] 5 is already present
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
1 error
28 warnings](https://content.bartleby.com/qna-images/question/1e26a685-55e2-4925-ba70-9f39a5b1116e/51d08876-706e-418a-9629-5d46558ad263/0lz9wx_thumbnail.png)
Transcribed Image Text:where T is a type-variable:
T extends Object declared in interface Comparable
MyBSTree.java:54: warning: [unchecked] unchecked call to compareTo (T) as
else if (temp.data.compareTo (item) < 0)
where T is a type-variable:
T extends Object declared in interface Comparable
Main.java:3: warning: [rawtypes] found raw type: ITree
ITree tree = new MyBSTree ();
missing type arguments for generic class ITree<T>
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
Main.java:3: warning: [rawtypes] found raw type: MyBSTree
ITree tree = new MyBSTree ();
missing type arguments for generic class MyBSTree<T>
where T is a type-variable:
T extends Comparable<? super T> declared in class MyBSTree
Main.java:4: warning: [unchecked] unchecked call to contains Item (T) as a member of the raw t
System.out.println (tree.containsItem (1)); // empty tree, 1 not present
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
Main.java:5: warning: [unchecked] unchecked call to insert (T) as a member of the raw type IT
tree.insert (2);
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
Main.java:6: warning: [unchecked] unchecked call to contains Item (T) as a member of the raw t
System.out.println (tree.contains Item (1)); // tree [2], 1 not present
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
Main.java:7: warning: [unchecked] unchecked call to insert (T) as a member of the raw type IT
tree.insert (1);
a member of the raw
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
Main.java:8: warning: [unchecked] unchecked call to contains Item (T) as a member of the raw t
System.out.println (tree.contains Item(1)); // tree [1, 2] 1 is present
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
Main.java:9: warning: [unchecked] unchecked call to insert (T) as a member of the raw type IT
tree.insert (5);
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
Main.java:10: warning: [unchecked] unchecked call to containsItem (T) as a member of the raw
System.out.println (tree.contains Item (4)); // tree [1, 2, 5] 4 is not present
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
Main.java:11: warning: [unchecked] unchecked call to insert (T) as a member of the raw type I
tree.insert (3);
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
Main.java:12: warning: [unchecked] unchecked call to containsItem (T) as a member of the raw
System.out.println (tree.contains Item (4)); // tree [1, 2, 3, 5] 4 is not present
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
Main.java:14: warning: [unchecked] unchecked call to insert (T) as a member of the raw type I
tree.insert (5);
// tree [1, 2, 3, 5] 5 is already present
where T is a type-variable:
T extends Comparable<T> declared in interface ITree
1 error
28 warnings
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
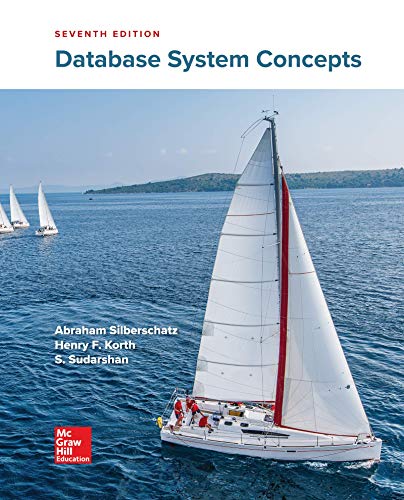
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
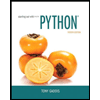
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
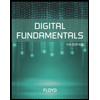
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
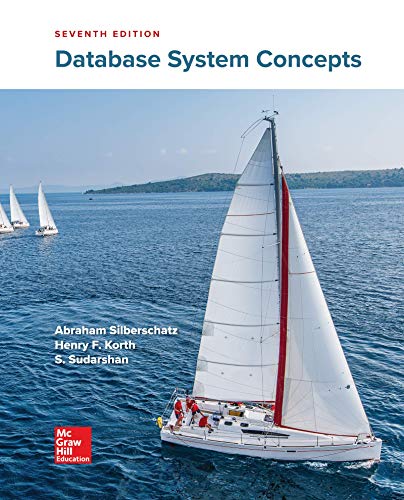
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
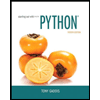
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
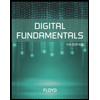
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
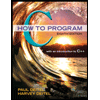
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
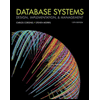
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
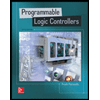
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education