Need help in java please I am doing a program that uses the insertionsort() method. The output should look like this insertionsort() Test: BEFORE: [42, 23, 20, 46, 90, 53, 13, 24, 74, 100] BEFORE: [byzantine, iceberg, yellow, dark cyan, antique bronze, dark slate gray, telemagenta, jet, dark red, red salsa] AFTER: [13, 20, 23, 24, 42, 46, 53, 74, 90, 100] AFTER: [antique bronze, byzantine, dark cyan, dark red, dark slate gray, iceberg, jet, red salsa, telemagenta, yellow] Numbers Result Correct?: true Colors Result Correct?: true however I have this error and I'm unsure if I'm doing it right, I provided my code and the output I get please help me
Need help in java please I am doing a program that uses the insertionsort() method. The output should look like this insertionsort() Test: BEFORE: [42, 23, 20, 46, 90, 53, 13, 24, 74, 100] BEFORE: [byzantine, iceberg, yellow, dark cyan, antique bronze, dark slate gray, telemagenta, jet, dark red, red salsa] AFTER: [13, 20, 23, 24, 42, 46, 53, 74, 90, 100] AFTER: [antique bronze, byzantine, dark cyan, dark red, dark slate gray, iceberg, jet, red salsa, telemagenta, yellow] Numbers Result Correct?: true Colors Result Correct?: true however I have this error and I'm unsure if I'm doing it right, I provided my code and the output I get please help me
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Need help in java please
I am doing a program that uses the insertionsort() method. The output should look like this
insertionsort() Test:
BEFORE: [42, 23, 20, 46, 90, 53, 13, 24, 74, 100]
BEFORE: [byzantine, iceberg, yellow, dark cyan, antique bronze, dark slate gray, telemagenta, jet, dark red, red salsa]
AFTER: [13, 20, 23, 24, 42, 46, 53, 74, 90, 100]
AFTER: [antique bronze, byzantine, dark cyan, dark red, dark slate gray, iceberg, jet, red salsa, telemagenta, yellow]
Numbers Result Correct?: true
Colors Result Correct?: true
however I have this error and I'm unsure if I'm doing it right, I provided my code and the output I get please help me
![The image contains a Java code snippet intended to implement an insertion sort on an `ArrayList`. Here is a transcription of the code:
```java
public static <E extends Comparable<E>> void insertionsort(ArrayList<E> myList) {
/**
* need to fix the && issue
*/
for (int i = 1; i <= myList.size(); i++) {
int temp = (int) myList.get(i);
int j = i;
while (j > 0) && (myList.get(j - 1) > myList.size()[j]) {
int temp = myList.size[j];
myList.set(j, myList.get(j - 1));
myList.set(j - 1, temp);
j--;
}
}
}
//TODO: Implement Insertion Sort
```
### Explanation
**Code Structure:**
- The method `insertionsort` is defined as static and generic, requiring elements that extend `Comparable<E>`.
- The method accepts an `ArrayList<E>` called `myList`.
**Comments:**
- There is an inline comment indicating that a logical issue with the `&&` operator needs to be fixed.
**Logic:**
- A `for` loop initializes at 1 and runs till the size of `myList`.
- Inside the loop, a `while` loop attempts to reorder elements based on a comparison.
**Issues:**
- The code contains an issue where the `&&` operator is incorrectly used, and there is confusion between using size and indexing.
- There is a TODO comment acknowledging that the insertion sort implementation is incomplete.
This snippet requires debugging and refinement to properly implement the insertion sort algorithm.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F972a81e1-caa2-41dc-a3c7-a688283b6b6c%2F704d4e08-55d6-4433-8e37-be7b110c2bf4%2Flw8o31h_processed.png&w=3840&q=75)
Transcribed Image Text:The image contains a Java code snippet intended to implement an insertion sort on an `ArrayList`. Here is a transcription of the code:
```java
public static <E extends Comparable<E>> void insertionsort(ArrayList<E> myList) {
/**
* need to fix the && issue
*/
for (int i = 1; i <= myList.size(); i++) {
int temp = (int) myList.get(i);
int j = i;
while (j > 0) && (myList.get(j - 1) > myList.size()[j]) {
int temp = myList.size[j];
myList.set(j, myList.get(j - 1));
myList.set(j - 1, temp);
j--;
}
}
}
//TODO: Implement Insertion Sort
```
### Explanation
**Code Structure:**
- The method `insertionsort` is defined as static and generic, requiring elements that extend `Comparable<E>`.
- The method accepts an `ArrayList<E>` called `myList`.
**Comments:**
- There is an inline comment indicating that a logical issue with the `&&` operator needs to be fixed.
**Logic:**
- A `for` loop initializes at 1 and runs till the size of `myList`.
- Inside the loop, a `while` loop attempts to reorder elements based on a comparison.
**Issues:**
- The code contains an issue where the `&&` operator is incorrectly used, and there is confusion between using size and indexing.
- There is a TODO comment acknowledging that the insertion sort implementation is incomplete.
This snippet requires debugging and refinement to properly implement the insertion sort algorithm.
![### Transcription and Explanation
#### Code Implementation Section
- **Line 71**: This line comments on the task to be implemented, which is "Insertion Sort."
#### Console Output
- **Insertion Sort Test:**
- **BEFORE** (Numeric Array): `[60, 34, 65, 15, 77, 18, 42, 8, 65, 30]`
- **BEFORE** (Color Names Array): `[tuscan brown, china pink, bright yellow, zaffre, japanese carmine, lemon meringue, persimmon, rose pompadour, space cadet, sweet brown]`
#### Error Message
- **Error**: `java.lang.Error: Unresolved compilation problem:`
- **Detail**: `Syntax error on token "&&", invalid OnlySynchronized`
### Analysis
- **Task**: The comment at the top indicates that the task is to implement the "Insertion Sort" algorithm in the code.
- **Arrays to be Sorted**: The console is displaying two arrays that are intended to be sorted:
1. A numerical array of integers.
2. An array of color names in string format.
- **Compilation Error**: The code execution has resulted in a syntax error specifically related to an incorrect usage of "&&". This suggests there’s an issue in the code which needs to be fixed to proceed with the sorting implementation.
### Steps to Resolve
1. **Code Review**: Examine the Java code to locate the incorrect usage of "&&". This likely involves looking for a conditional statement or a method where "&&" is used incorrectly.
2. **Correct Syntax**: Ensure that all conditional statements are properly structured, using "&&" in line with Java's syntax rules.
3. **Implement Insertion Sort**: Once the syntax error is resolved, proceed with implementing the insertion sort on the provided arrays.
This overview covers the necessary information for an educational audience about transcribing what is needed and how to handle the code issues encountered.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F972a81e1-caa2-41dc-a3c7-a688283b6b6c%2F704d4e08-55d6-4433-8e37-be7b110c2bf4%2Fon4cmb_processed.png&w=3840&q=75)
Transcribed Image Text:### Transcription and Explanation
#### Code Implementation Section
- **Line 71**: This line comments on the task to be implemented, which is "Insertion Sort."
#### Console Output
- **Insertion Sort Test:**
- **BEFORE** (Numeric Array): `[60, 34, 65, 15, 77, 18, 42, 8, 65, 30]`
- **BEFORE** (Color Names Array): `[tuscan brown, china pink, bright yellow, zaffre, japanese carmine, lemon meringue, persimmon, rose pompadour, space cadet, sweet brown]`
#### Error Message
- **Error**: `java.lang.Error: Unresolved compilation problem:`
- **Detail**: `Syntax error on token "&&", invalid OnlySynchronized`
### Analysis
- **Task**: The comment at the top indicates that the task is to implement the "Insertion Sort" algorithm in the code.
- **Arrays to be Sorted**: The console is displaying two arrays that are intended to be sorted:
1. A numerical array of integers.
2. An array of color names in string format.
- **Compilation Error**: The code execution has resulted in a syntax error specifically related to an incorrect usage of "&&". This suggests there’s an issue in the code which needs to be fixed to proceed with the sorting implementation.
### Steps to Resolve
1. **Code Review**: Examine the Java code to locate the incorrect usage of "&&". This likely involves looking for a conditional statement or a method where "&&" is used incorrectly.
2. **Correct Syntax**: Ensure that all conditional statements are properly structured, using "&&" in line with Java's syntax rules.
3. **Implement Insertion Sort**: Once the syntax error is resolved, proceed with implementing the insertion sort on the provided arrays.
This overview covers the necessary information for an educational audience about transcribing what is needed and how to handle the code issues encountered.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
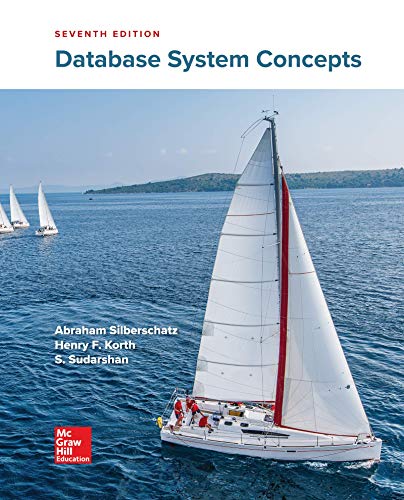
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
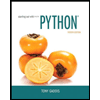
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
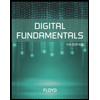
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
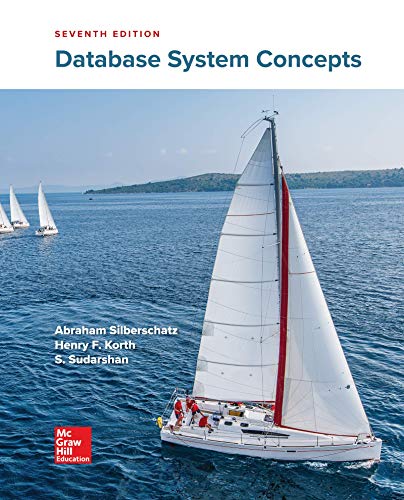
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
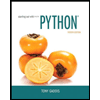
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
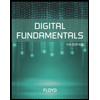
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
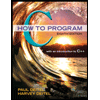
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
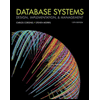
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
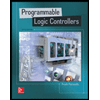
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education