Write a Java program that asks the user to enter an array of integers in the main The program will ask the user for the number of integer elements to be put in the array, and then ask the user for each element of the array. The program then calls a method named isSorted() that accepts an array of integers and returns true if the list is in sorted (increasing) order and false otherwise. For example, if arrays named arr1 and arr2 store [10, 20, 30, 41, 56] and [2, 5, 3, 12, 10] respectively, the calls sorted(arr1) and isSorted(arr2) should return true and false respectively. Assume the array has at least one integer element. A one-element array is considered to be sorted.
. DO NOT REJECT THE QUESTION. ----
Write a Java program that asks the user to enter an array of integers in the main The program will ask the user for the number of integer elements to be put in the array, and then ask the user for each element of the array. The program then calls a method named isSorted() that accepts an array of integers and returns true if the list is in sorted (increasing) order and false otherwise. For example, if arrays named arr1 and arr2 store [10, 20, 30, 41, 56] and [2, 5, 3, 12, 10] respectively, the calls sorted(arr1) and isSorted(arr2) should return true and false respectively. Assume the array has at least one integer element. A one-element array is considered to be sorted.

Code:
import java.util.Scanner; imported libraby for accepting input
public class Ary { declared class - same as filename
public static boolean isSorted(int[] ar) { declared method
for (int i = 0; i < ar.length - 1; i++) { declared for loop to read each element
if (ar[i] > ar[i + 1]) { if condition to check number should not be more than next i.e its not sorted
return false; returns the false signal to the method we call
}
}
return true; returns true signal to method we call
}
public static void main(String[] args) {
int n; declared n to store size of array
int Aray[]; declared array
Scanner sc = new Scanner(System.in); created scanner object to take input from user
System.out.println("Enter number of elements to insert in array: "); shows message
n = sc.nextInt(); store's the inputed value to left side variable n
Aray = new int[n]; allocates the n-size memory to array
for(int i=0;i<n;i++) initialising a for-loop to enter element 1 by 1 in array
{
System.out.println("Enter "+(i+1)+ " element: "); shows which element we are entering
Aray[i] = sc.nextInt(); accepts element and store in left side Aray[i], value of i will increase by 1 each time
} for loop ends here after value of i = 5
System.out.println("\n" + isSorted(Aray)); prints result of this method(true / false)
}
}
Step by step
Solved in 3 steps with 4 images

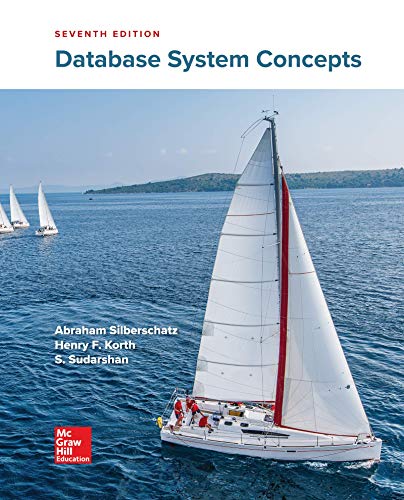
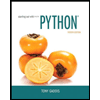
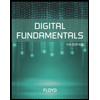
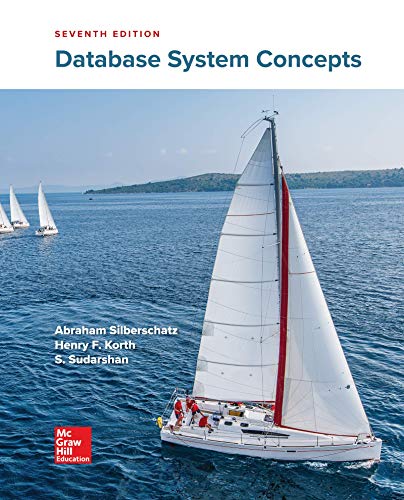
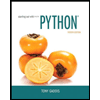
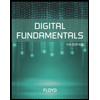
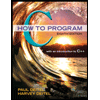
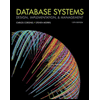
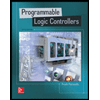