this program you need to implement four methods with the following signatures: printArr(int[] a) add(int[] a, int[] b) subtract(int[] a, int[] b) negate(int[] a) 1. Method printArr(a) should print out all elements of a given int array in one line. 2. Method add(a, b) should take two int arrays (assume they have the same length) and return a new array each element of which will be the sum of the corresponding elements in a and b 3. Method subtract(a, b) is similar to add(), except it returns (a - b) array 4. Method negate(a)takes an int array and returns a new array in which every element is multiplied by -1. */ public class ArrayOperations{ public static void main(String[] args){ //The main only has the testing code. //You don't have to change it. int [] a = { 1, 2, 3, 4, 5}; int [] b = {10, 1, 3, 5, 7}; System.out.print("a = "); printArr(a);//Should print each element of a (in one line) System.out.print("b = "); printArr(b);//Should print each element of b (in one line) int [] arr = add(a,b); System.out.print("a + b = "); printArr( arr );//Should print out the array a+b System.out.print("a - b = "); printArr(subtract(a,b));//Should print out the array a-b System.out.print("b - a = "); printArr(subtract(b,a));//Should print out the array b-a System.out.print("-a = "); printArr(negate(a));//Should print out the array -a (negativ
/*
In this program you need to implement four methods with the following signatures:
printArr(int[] a)
add(int[] a, int[] b)
subtract(int[] a, int[] b)
negate(int[] a)
public class ArrayOperations{
public static void main(String[] args){
//The main only has the testing code.
//You don't have to change it.
int [] a = { 1, 2, 3, 4, 5};
int [] b = {10, 1, 3, 5, 7};
System.out.print("a = ");
printArr(a);//Should print each element of a (in one line)
System.out.print("b = ");
printArr(b);//Should print each element of b (in one line)
int [] arr = add(a,b);
System.out.print("a + b = ");
printArr( arr );//Should print out the array a+b
System.out.print("a - b = ");
printArr(subtract(a,b));//Should print out the array a-b
System.out.print("b - a = ");
printArr(subtract(b,a));//Should print out the array b-a
System.out.print("-a = ");
printArr(negate(a));//Should print out the array -a (negative a)
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

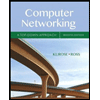
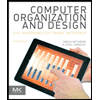
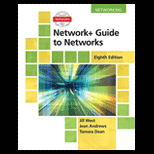
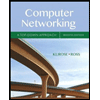
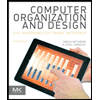
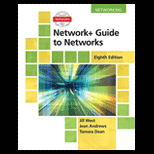
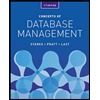
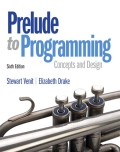
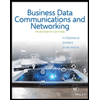