Write 3 functions: Write int readNumbersIntoArray(int numbers[], int size); // Reads up to size numbers or a non-numeric and puts them in the array. // Returns how many numbers read. Write int minOrMax(int numbers[], int size, bool Max); // Returns the minimum or the maximum value in the array depending on "Max". // If "Max" is true, then it returns the maximum. // If "Max" is false, then it returns the minimum. Write double trimmedAvg(int numbers[], int size); // Returns the trimmed average of the array. // A trimmed average is a normal average but leaves out the first instance of // the smallest and largest value in the array when doing the calculation. // HINT: use calls to minOrMax() to get the largest and smallest number. Write int main(): // Prompt the user for between 3 and 20 numbers and use readNumbersIntoArray() to // read them into an array. Print an error message and exit if less than three numbers. // Print out the min, max, and trimmedAverage of the array using calls to minOrMax() and // trimmedAvg(). User input is shown in bold below: // Sample run 1: Enter from 3 to 20 numbers followed by a non-numeric: 1 2 q Must be between 3 and 20 numbers! // Sample run 2: Enter from 3 to 20 numbers followed by a non-numeric: -8 2 3 -2 57 q Minimum value in the array: -8 Maximum value in the array: 57 Trimmed average of the array: 1 // Sample run 3: Enter from 3 to 20 numbers followed by a non-numeric: 3, -22, 5, 68, 21, -6, 2 q Minimum value in the array: -22 Maximum value in the array: 68 Trimmed average of the array: 5 Write program C++ program with comments.
Write 3 functions:
Write
int readNumbersIntoArray(int numbers[], int size);
// Reads up to size numbers or a non-numeric and puts them in the array.
// Returns how many numbers read.
Write
int minOrMax(int numbers[], int size, bool Max);
// Returns the minimum or the maximum value in the array depending on "Max".
// If "Max" is true, then it returns the maximum.
// If "Max" is false, then it returns the minimum.
Write
double trimmedAvg(int numbers[], int size);
// Returns the trimmed average of the array.
// A trimmed average is a normal average but leaves out the first instance of // the smallest and largest value in the array when doing the calculation.
// HINT: use calls to minOrMax() to get the largest and smallest number.
Write
int main():
// Prompt the user for between 3 and 20 numbers and use readNumbersIntoArray() to
// read them into an array. Print an error message and exit if less than three numbers.
// Print out the min, max, and trimmedAverage of the array using calls to minOrMax() and
// trimmedAvg().
User input is shown in bold below:
// Sample run 1: Enter from 3 to 20 numbers followed by a non-numeric:
1 2 q
Must be between 3 and 20 numbers! // Sample run 2:
Enter from 3 to 20 numbers followed by a non-numeric:
-8 2 3 -2 57 q
Minimum value in the array: -8
Maximum value in the array: 57
Trimmed average of the array: 1
// Sample run 3: Enter from 3 to 20 numbers followed by a non-numeric:
3, -22, 5, 68, 21, -6, 2 q
Minimum value in the array: -22
Maximum value in the array: 68
Trimmed average of the array: 5
Write program C++ program with comments.

Step by step
Solved in 4 steps with 2 images

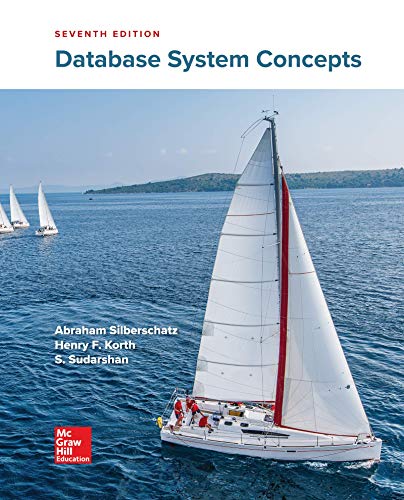
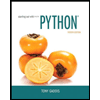
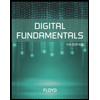
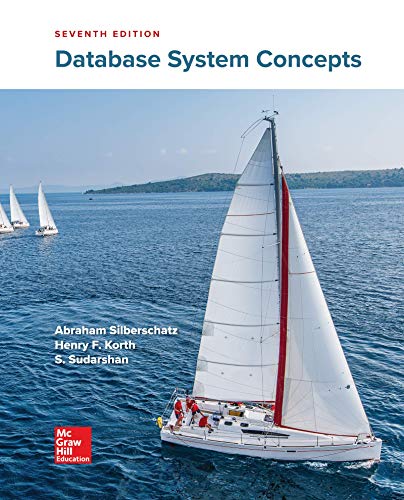
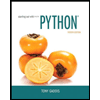
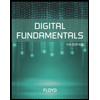
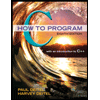
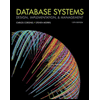
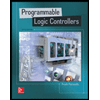