Task 1: Implement the Order Class Read the Java API (doc/1ab6/Order.html). Create a new class named Order. Define private instance variables: orderld, customerName, productName, quantity, and unitPrice. Implement a constructor to initialize these variables. Implement a method getTotalAmount() that calculates and returns the total amount of the order (quantity* unit price). Implement the toString() method to provide a string representation of the order details. Make sure to use proper encapsulation and rounding of values. ● Task 2: Implement the IOHandler Class Read the Java API (doc/1ab6/IOHandler.html). Create a new class named IOHandler. ● Implement a static method readFile(String fileName) that reads the content of a file and returns it as a list of strings. Implement a static method writeFile(String fileName, List content) that writes the provided content to a file. Properly handle potential FileNotFoundException and IOException with informative error messages. Task 3: Implement the Container Class ● Read the Java API (doc/1ab6/Container.html). Create a new class named Container. Implement methods: add(Object object), remove(), and getSize() for adding, removing, and querying the size of objects in the container. Use appropriate data structures and logic to achieve these functionalities. ● Task 4: Implement the Queue Class (Extends Container) Read the Java API (doc/1ab6/Queue.html). ● Create a new class named Queue that extends the Container class. Implement a constructor that reads orders from a file and adds orders with a total amount above or equal to 1500 to the queue. Override the add(Object obj) method to add orders only if their total amount is above or equal to 1500. Implement the remove() method to remove and return orders from the front of the queue. Implement the top() method to return the order at the front of the queue without removing it. Implement the getSize() method to return the number of orders in the queue. ● ● Task 5: Implement the Stack Class (Extends Container) ● Create a new class named Stack that extends the Container class. Implement a constructor that reads orders from a file and adds orders with a total amount below 1500 to the stack. Override the add(Object obj) method to add orders only if their total amount is below 1500. ● Implement the remove() method to remove and return orders from the top of the stack. Implement the top() method to return the order at the top of the stack without removing it.
import java.text.DecimalFormat;
import java.io.*;
import java.util.*;
public class Container {
private List<Object> objects = new ArrayList<>(); implement the tasks for the following code.
public void add(Object object) {
objects.add(object);
}
public Object remove() {
if (!objects.isEmpty()) {
return objects.remove(objects.size() - 1);
}
return null;
}
public int getSize() {
return objects.size();
}
public Order top() {
if (!objects.isEmpty() && objects.get(objects.size() - 1) instanceof Order) {
return (Order) objects.get(objects.size() - 1);
}
return null;
}
}
class Queue extends Container {
public Queue(List<Order> orders) {
for (Order order : orders) {
if (order.getTotalAmount() >= 1500) {
super.add(order);
}
}
}
@Override
public void add(Object obj) {
if (((Order) obj).getTotalAmount() >= 1500) {
super.add(obj);
}
}
public Order remove() {
if (getSize() > 0) {
Order order = (Order) super.top();
super.remove();
return order;
}
return null;
}
public Order top() {
if (getSize() > 0) {
return (Order) super.top();
}
return null;
}
}
class Stack extends Container {
public Stack(List<Order> orders) {
for (Order order : orders) {
if (order.getTotalAmount() < 1500) {
super.add(order);
}
}
}
@Override
public void add(Object obj) {
if (((Order) obj).getTotalAmount() < 1500) {
super.add(obj);
}
}
public Object remove() {
if (getSize() > 0) {
Order order = (Order) super.top();
super.remove();
return order;
}
return null;
}
public Order top() {
if (getSize() > 0) {
return (Order) super.top();
}
return null;
}
}
class IOHandler {
public static List<String> readFile(String fileName) {
List<String> content = new ArrayList<>();
try (BufferedReader br = new BufferedReader(new FileReader(fileName))) {
String line;
while ((line = br.readLine()) != null) {
content.add(line);
}
} catch (FileNotFoundException e) {
System.err.println("File not found: " + fileName);
} catch (IOException e) {
System.err.println("Error reading file: " + e.getMessage());
}
return content;
}
public static void writeFile(String fileName, List<String> content) {
try (BufferedWriter bw = new BufferedWriter(new FileWriter(fileName))) {
for (String line : content) {
bw.write(line);
bw.newLine();
}
} catch (IOException e) {
System.err.println("Error writing to file: " + e.getMessage());
}
}
}
class Order {
private int orderId;
private String customerName;
private String productName;
private int quantity;
private double unitPrice;
public Order(int orderId, String customerName, String productName, int quantity, double unitPrice) {
this.orderId = orderId;
this.customerName = customerName;
this.productName = productName;
this.quantity = quantity;
this.unitPrice = unitPrice;
}
public double getTotalAmount() {
return quantity * unitPrice;
}
@Override
public String toString() {
DecimalFormat df = new DecimalFormat("#.##");
return "Order ID: " + orderId +
"\nCustomer Name: " + customerName +
"\nProduct Name: " + productName +
"\nQuantity: " + quantity +
"\nUnit Price: $" + df.format(unitPrice) +
"\nTotal Amount: $" + df.format(getTotalAmount());
}
}


Unlock instant AI solutions
Tap the button
to generate a solution
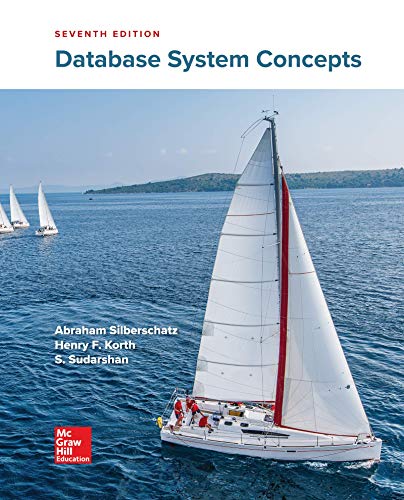
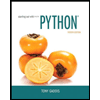
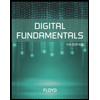
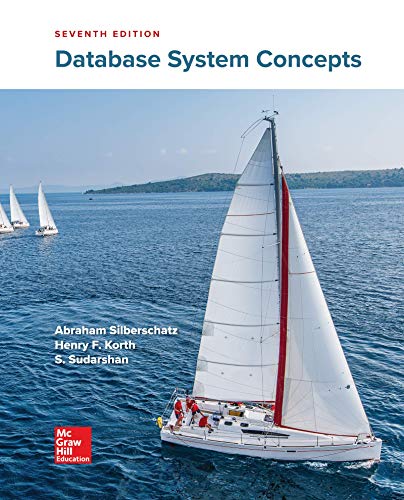
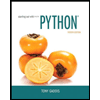
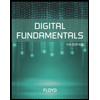
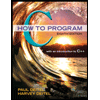
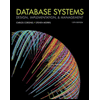
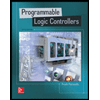