ys in creating your classes. Java language. void insert(Object, int index): Add the object at the specified index. void append(Object): Add the object at the end of the list. Object remove(int index): Remove and return the object at the specified index. int size() String toString() boolean isEmpty() int getIndexOf(Object): Returns -1 if not found boolean contains(Object): Return
Use arrays in creating your classes. Java language.
- void insert(Object, int index): Add the object at the specified index.
- void append(Object): Add the object at the end of the list.
- Object remove(int index): Remove and return the object at the specified index.
- int size()
- String toString()
- boolean isEmpty()
- int getIndexOf(Object): Returns -1 if not found
- boolean contains(Object): Return true if the object is in the list. Use equals of the object for comparison.
- boolean equals(Object): Compare sizes and elements in the data structure.
- Object get(int index): Returns the object at index specified.
System.out.println("ArrayList Tests");
// todo: make more tests here
ArrayList a = new ArrayList();
System.out.println("Check empty array isEmpty:" + a.isEmpty());
a.insert('B', 0);
a.insert('a', 0);
a.insert('t', 1);
System.out.println("Check non-empty array isEmpty:" + a.isEmpty());
System.out.println(a.toString());
while (a.isEmpty() == false) {
System.out.println(a.remove(0));
}
// Fill over initial capacity and check that it grows
for (int i = 0; i < 110; i++)
{
a.append(new Integer(i));
}
System.out.println("Size of array after 110 adds: "+ a.size());
System.out.println("Value of last element: "+ a.get(a.size()-1));
System.out.println("Insert past end of list");
a.insert('z', 200);
System.out.println("Insert negative index");
a.insert('z', -3);
System.out.println("Remove past end of list");
a.remove(200);

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

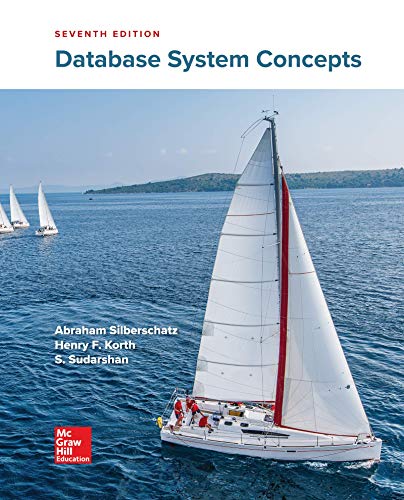
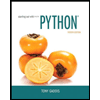
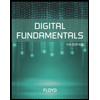
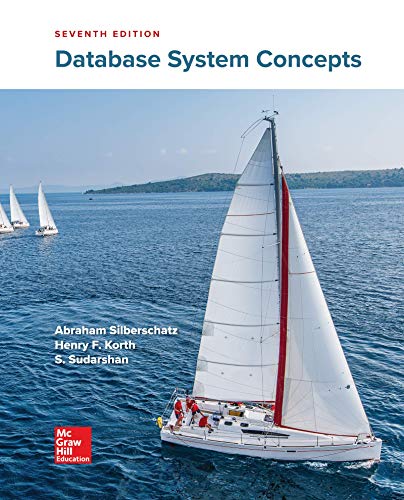
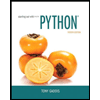
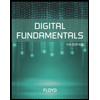
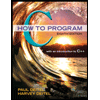
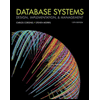
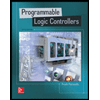