Create an abstract class called vehicle. The class should declare the following variables: an instance variable that describes the model - String an instance variable that describes the number - String an instance variable that describes the maker - String an instance variable that describes the price - double an instance variable that describes the year – integer Provide a toString() method that returns the information stored in the above variables. Create the getter and setter methods for each instance variable except price. Provide the necessary constructors. Include an abstract method setPrice(double price) to determine the price for a vehicle. Include an abstract method getColor() to return the color of the vehicle.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Create an abstract class called vehicle. The class should declare the following variables:
- an instance variable that describes the model - String
- an instance variable that describes the number - String
- an instance variable that describes the maker - String
- an instance variable that describes the price - double
- an instance variable that describes the year – integer
Provide a toString() method that returns the information stored in the above variables.
Create the getter and setter methods for each instance variable except price. Provide the necessary constructors. Include an abstract method setPrice(double price) to determine the price for a vehicle. Include an abstract method getColor() to return the color of the vehicle.
Create two subclasses called Car and Truck .
These subclasses should override the abstract methods setPrice and getColor of class vehicle.
Use the following rule for setting the price for a vehicle:
-
- car will have a 30% discount per each vehicle
- truck will have a 25 % discount per each vehicle).
Write a driver program (another class with main method) that uses the above hierarchy. In your driver program you must implement an interaction with the user.
- Use showInputDialog method to let the user input vehicle information.
- Use showMessageDialog method to display vehicle information including price and type for both car and truck vehicles.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

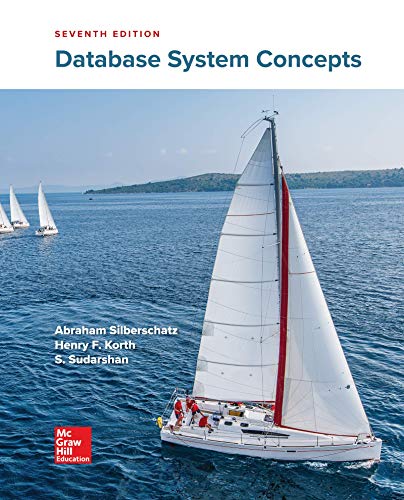
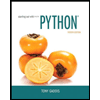
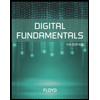
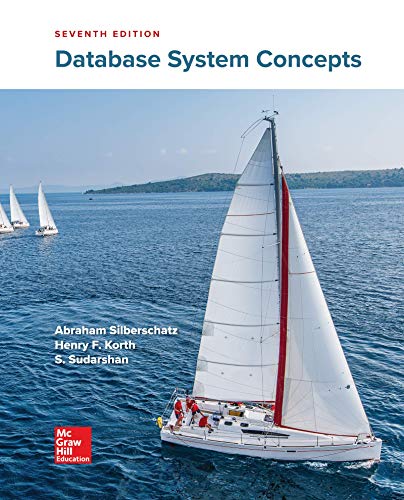
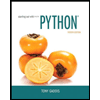
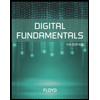
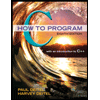
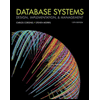
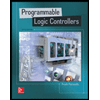