Create a class that represents an employee. This class will have three constructors to initialize variables. If the constructor doesn't provide a parameter for a field, make it either "(not set)" or "0" as appropriate. Name: Employee Fields: - name : String - idNumber : int - department : String - position : String Methods: + Employee() + Employee(name : String, idNumber : int) + Employee(name : String, idNumber : int, department : String, position : String) + getName() : String + getDepartment() : String + getPosition() : String + getIdNumber() : int use test case as result
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Create a class that represents an employee. This class will have three constructors to initialize variables. If the constructor doesn't provide a parameter for a field, make it either "(not set)" or "0" as appropriate.
Name: Employee
Fields: - name : String - idNumber : int - department : String - position : String
Methods: + Employee() + Employee(name : String, idNumber : int) + Employee(name : String, idNumber : int, department : String, position : String) + getName() : String + getDepartment() : String + getPosition() : String + getIdNumber() : int
use test case as result


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

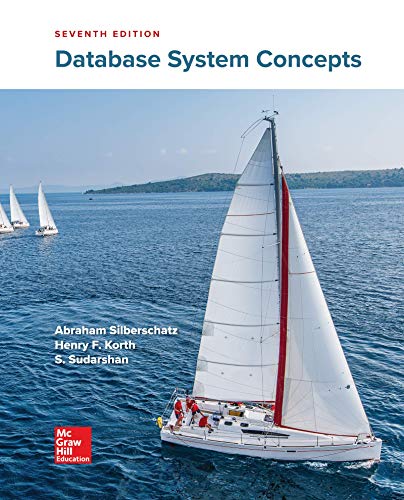
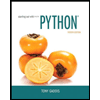
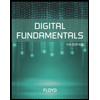
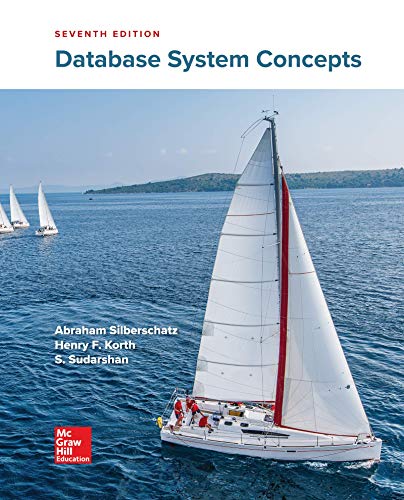
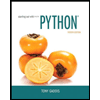
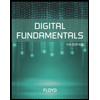
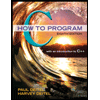
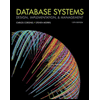
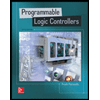