Modify the Car class example and add the following: A default constructor that initializes strings to “N/A” and numbers to zero. An overloaded constructor that takes in initial values to set your fields (6 parameters). accel() method: a void method that takes a double variable as a parameter and increases the speed of the car by that amount. brake() method: a void method that sets the speed to zero. To test your class, you will need to instantiate at least two different Car objects in the main method in the CarDemo class. Each of your objects should use a different constructor. For the object that uses the default constructor, you will need to invoke the mutator methods to assign values to your fields. Invoke the displayFeatures() method for both objects and display the variables. Demonstrate the use of the accessor method getColor() by accessing the color of each car and printing it to the console. Use the method accel to increase the speed of the first car by 65 mph. Use the method brake to set the speed of the first car to 0. Add a third reference variable that refers to one of the existing objects. Invoke the getColor() method for both reference variables that refer to the same object. They should both have the same color. Use println to print the color for both variables.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Modify the Car class example and add the following:
- A default constructor that initializes strings to “N/A” and numbers to zero.
- An overloaded constructor that takes in initial values to set your fields (6
- parameters).
- accel() method: a void method that takes a double variable as a parameter and
increases the speed of the car by that amount.
- brake() method: a void method that sets the speed to zero.
- To test your class, you will need to instantiate at least two different Car objects in the main method in the CarDemo class.
- Each of your objects should use a different constructor. For the object that uses
the default constructor, you will need to invoke the mutator methods to assign
values to your fields.
- Invoke the displayFeatures() method for both objects and display the variables.
- Demonstrate the use of the accessor method getColor() by accessing the color of each car and printing it to the console.
- Use the method accel to increase the speed of the first car by 65 mph.
- Use the method brake to set the speed of the first car to 0.
- Add a third reference variable that refers to one of the existing objects.
- Invoke the getColor() method for both reference variables that refer to the same
object. They should both have the same color. Use println to print the color for
both variables.


Step by step
Solved in 3 steps with 5 images

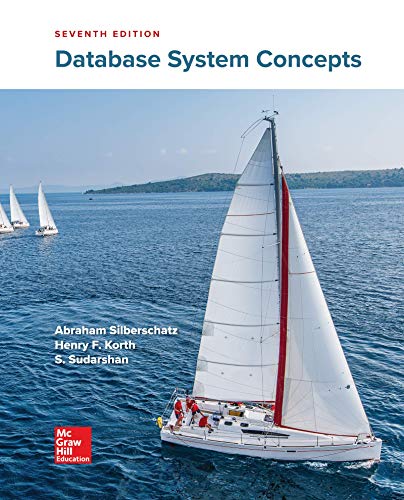
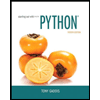
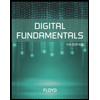
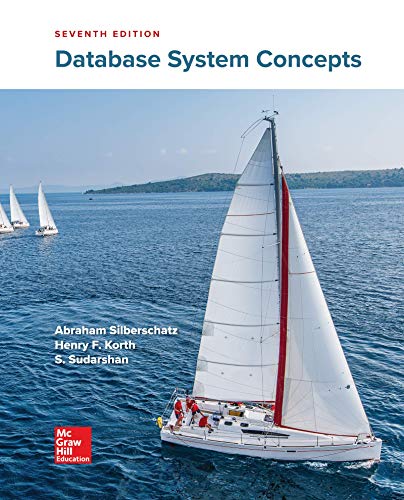
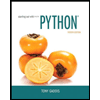
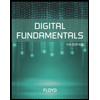
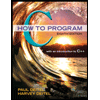
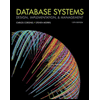
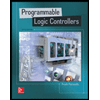