1 - Student class Make a class student (in student.py) that stores the following information for a student: Name (name) Student number (student_nr) Points per assignment (points_per_assignment) Exam grade (exam_grade) a) Behind each point of information is the name of the parameter to the initializer method. Store this information from the parameters in the object attributes with the same name. b) Add a method course_points() which returns the number of course points the student has gotten. Example: mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9)print(mary.course_points()) > 121 The calculation of the course points is explained in the course overview, course setup slides (Links to an external site.) and the first lectureLinks to an external site.. c) Add a method grade() which returns a the final grade of the student, rounded to nearest half (upwards, 6.75 -> 7). As per regulations, a 5.5 becomes a 6. If the student did not pass both the assignments (>= 95 course points) and the exam (exam grade >= 5.5), then the grade is "NVD" (niet voldaan = not fulfilled). For example: mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9)print(mary.grade()) > 9.5joe = Student("Joe", 15789688, [10, 9, 8, 10, 5, 10], 5)print(joe.grade()) > "NVD" d) Add a method result() which gives the final result of the student for the VU administration. For example: mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9)print(mary.result()) > Mary (15789613) has scored 9.5 on X_401096joe = Student("Joe", 15789688, [10, 9, 8, 10, 5, 10], 5)print(joe.result()) > Joe (15789688) has scored NVD on X_401096 e) Add a method passes() that returns if a student passes the course. mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9)print(mary.passes()) > Truejoe = Student("Joe", 15789688, [10, 9, 8, 10, 5, 10], 5)print(joe.passes()) > False f) Implement the method a.scores_higher_than(b) that determines if student a scores higher than student b. A student scores higher than another student if their grade is higher (any grade is higher than "NVD"). If students a and b have the same grade, then neither does a score higher than b, nor the other way around. mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9)joe = Student("Joe", 15789688, [10, 9, 8, 10, 5, 10], 5)joe.scores_higher_than(mary)> False g) Make a class Class (as in a class of students, not a class as in a class of objects) that stores several Student objects. Store a list of students in an attribute called students. The initializer method should initialize the class as an empty class (no students). Implement a method add_student() that takes an object of class Student and adds it to class. Implement method pass_rate() that determines the fraction of students that pass the class (have a grade of >= 6).
1 - Student class
Make a class student (in student.py) that stores the following information for a student:
- Name (name)
- Student number (student_nr)
- Points per assignment (points_per_assignment)
- Exam grade (exam_grade)
a) Behind each point of information is the name of the parameter to the initializer method. Store this information from the parameters in the object attributes with the same name.
b) Add a method course_points() which returns the number of course points the student has gotten. Example:
mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9)
print(mary.course_points())
> 121
The calculation of the course points is explained in the course overview, course setup slides (Links to an external site.) and the first lectureLinks to an external site..
c) Add a method grade() which returns a the final grade of the student, rounded to nearest half (upwards, 6.75 -> 7). As per regulations, a 5.5 becomes a 6. If the student did not pass both the assignments (>= 95 course points) and the exam (exam grade >= 5.5), then the grade is "NVD" (niet voldaan = not fulfilled). For example:
mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9)
print(mary.grade())
> 9.5
joe = Student("Joe", 15789688, [10, 9, 8, 10, 5, 10], 5)
print(joe.grade())
> "NVD"
d) Add a method result() which gives the final result of the student for the VU administration. For example:
mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9)
print(mary.result())
> Mary (15789613) has scored 9.5 on X_401096
joe = Student("Joe", 15789688, [10, 9, 8, 10, 5, 10], 5)
print(joe.result())
> Joe (15789688) has scored NVD on X_401096
e) Add a method passes() that returns if a student passes the course.
mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9)
print(mary.passes())
> True
joe = Student("Joe", 15789688, [10, 9, 8, 10, 5, 10], 5)
print(joe.passes())
> False
f) Implement the method a.scores_higher_than(b) that determines if student a scores higher than student b. A student scores higher than another student if their grade is higher (any grade is higher than "NVD"). If students a and b have the same grade, then neither does a score higher than b, nor the other way around.
mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9)
joe = Student("Joe", 15789688, [10, 9, 8, 10, 5, 10], 5)
joe.scores_higher_than(mary)
> False
g) Make a class Class (as in a class of students, not a class as in a class of objects) that stores several Student objects. Store a list of students in an attribute called students. The initializer method should initialize the class as an empty class (no students). Implement a method add_student() that takes an object of class Student and adds it to class. Implement method pass_rate() that determines the fraction of students that pass the class (have a grade of >= 6).

As per our guidelines, we are supposed to answer only 1st three parts. Kindly repost the remaining questions separately.
Algorithm:
- Start
- Create a class named Student with name, student_nr, points_per_assignment, and exam_grade as its attributes
- Define a method course_points() that returns the number of course points the student has gotten
- Define a method named grade() that returns the final grade of the student, rounded to the nearest half (upwards, 6.75 -> 7). If the student did not pass both the assignments (>= 95 course points) and the exam (exam grade >= 5.5), then the grade is "NVD"
- Inside the main method, create objects of Student class and then call the methods accordingly and print the returned values
- Stop
Step by step
Solved in 4 steps with 2 images

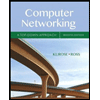
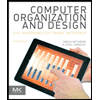
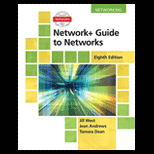
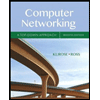
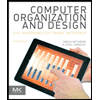
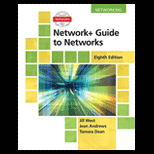
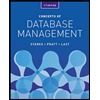
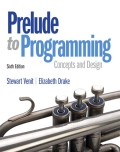
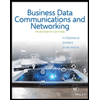