Create a class named Employee including a constructor and the necessary accessor andmutator methods. The class should keep the following information in fields: 1. Employee Name Employee Number in the format NNN-X, where each N is a digit and X is aletter represents department, which can be one of the following: H - Human Resources · A - Accounting · P - Production · S - Shipping Example: 902-A = an employee from Accounting department Hire Date Next, write a class named ProductionWorker that inherits from the Employee class. Includea constructor and the necessary accessor and mutator methods. The ProductionWorker classshould keep the following information in fields: Shift Number which is an integer and can be one of the following: 1 - represents Morning Shift 2 - represents Swing Shift 3 - represents Night Shift Hourly Pay Rate You are required to write an application that reads input of employees information from a text file (Information.txt) and decide from the employee number his/her department and the working shift the employee is assigned. A list of the employees and their information should be displayed onto console as well as written to a text file named Department.txt. Input: The input file will be named Information.txt. One record (line) will contain theemployee name, employee number, hire date, shift number and pay rate each separated byspaces (a non-production worker should be identified via zero number for the shift number). A sample text file is below. Susan 902-A 05/16/2009 1 16.25 Alex 823-S 06/21/2000 2 17.50 Ahmad 788-H 12/1/2003 0 Output: Output should consist of a listing of the employee name, employee number,employee's department, hire date, shift name and pay rate This information should bedisplayed on the screen as well as written to a text file named Department.txt in the format shown below: Name Employee No Department Hire Date Shift Name Pay Rate ------------------------------------------------------------------------------------------------------------------------------- Susan 902-A Accounting 05/16/2009 Morning Shift RM16.25 Alex 823-S Shipping 06/21/2000 Swing Shift RM17.50 Ahmad 788-H Human Resource 12/1/2003
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Create a class named Employee including a constructor and the necessary accessor andmutator methods. The class should keep the following information in fields:
1. Employee Name
- Employee Number in the format NNN-X, where each N is a digit and X is aletter represents department, which can be one of the following:
- H - Human Resources
· A - Accounting
· P - Production
· S - Shipping
Example: 902-A = an employee from Accounting department
- Hire Date
Next, write a class named ProductionWorker that inherits from the Employee class. Includea constructor and the necessary accessor and mutator methods. The ProductionWorker classshould keep the following information in fields:
- Shift Number which is an integer and can be one of the following:
1 - represents Morning Shift
2 - represents Swing Shift
3 - represents Night Shift
- Hourly Pay Rate
You are required to write an application that reads input of employees information from a text file (Information.txt) and decide from the employee number his/her department and the working shift the employee is assigned. A list of the employees and their information should be displayed onto console as well as written to a text file named Department.txt.
Input: The input file will be named Information.txt. One record (line) will contain theemployee name, employee number, hire date, shift number and pay rate each separated byspaces (a non-production worker should be identified via zero number for the shift number). A sample text file is below.
Susan 902-A 05/16/2009 1 16.25
Alex 823-S 06/21/2000 2 17.50
Ahmad 788-H 12/1/2003 0
Output: Output should consist of a listing of the employee name, employee number,employee's department, hire date, shift name and pay rate This information should bedisplayed on the screen as well as written to a text file named Department.txt in the format shown below:
Name Employee No Department Hire Date Shift Name Pay Rate
-------------------------------------------------------------------------------------------------------------------------------
Susan 902-A Accounting 05/16/2009 Morning Shift RM16.25
Alex 823-S Shipping 06/21/2000 Swing Shift RM17.50
Ahmad 788-H Human Resource 12/1/2003

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

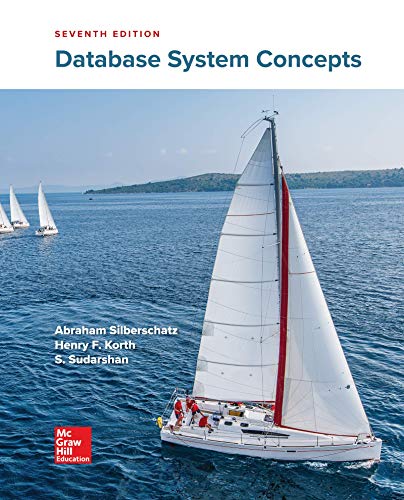
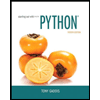
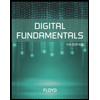
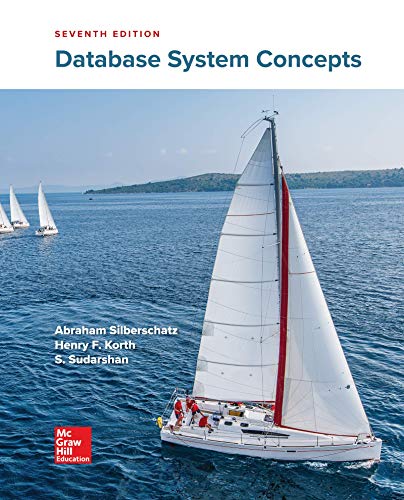
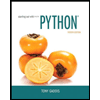
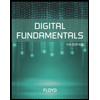
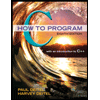
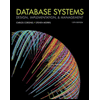
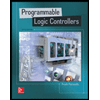