Consider the following definition of the class MyClass: public class MyClass
Exercise 1
Consider the following definition of the class MyClass:
public class MyClass
{
private static int count = 0;
private int x;
public MyClass(int i)
{
x = i;
}
public void incrementCount()
{
count++;
}
public void printX()
{
System.out.println("Value of x : " + x);
}
public static void printCount()
{
System.out.println("Value of count : " + count);
}
}
public class MyClassDemo
{
public static void main(String[] args)
{
MyClass myObject1 = new MyClass(5);
MyClass myObject2 = new MyClass(7);
}
}
What is the output of the following Java code? (Assume that following statements are written inside main)
myObject1.printX();
myObject1.incrementCount();
MyClass.incrementCount();
myObject1.printCount();
myObject2.printCount();
myObject2.printX();
myObject1.setX(14);
myObject1.incrementCount();
myObject1.printX();
myObject1.printCount();
myObject2.printCount();
----------------------------
Exercise 2 - Give the output of this code
public class Circle { // Save as "Circle.java"
// private instance variable, not accessible from outside this class
private double radius;
private String color;
// Constructors (overloaded)
/** Constructs a Circle instance with default value for radius and color */
public Circle() { // 1st (default) constructor
radius = 1.0;
color = "red";
}
/** Constructs a Circle instance with the given radius and default color */
public Circle(double r) { // 2nd constructor
radius = r;
color = "red";
}
/** Returns the radius */
public double getRadius() {
return radius;
}
/** Returns the area of this Circle instance */
public double getArea() {
return radius*radius*Math.PI;
}
}
-----
public class TestCircle { // Save as "TestCircle.java"
public static void main(String[] args) {
Circle c1 = new Circle();
System.out.println("The circle has radius of "
+ c1.getRadius() + " and area of " + c1.getArea());
Circle c2 = new Circle(2.0);
System.out.println("The circle has radius of "
+ c2.getRadius() + " and area of " + c2.getArea());
}
}
--------------------------
Exercise 3
Write a program that would print the information (name, year of joining, address) of three employees by creating a class named 'Employee'. The output should be as follows:
Name Year of joining Address
Robert 1994 Liloan
Sam 2000 Danao
John 1999 Cebu City

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

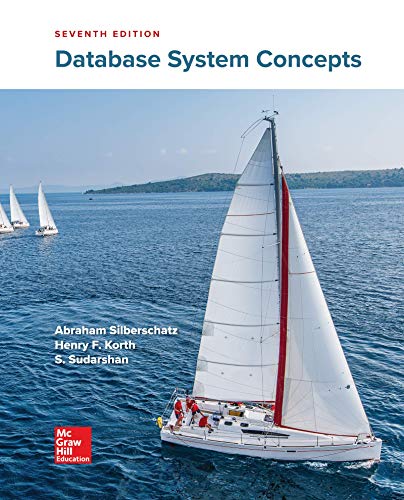
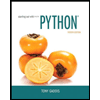
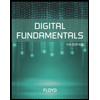
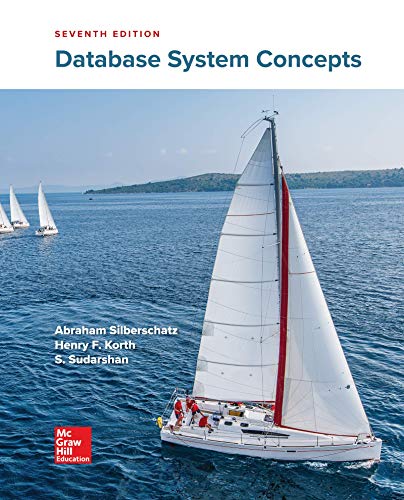
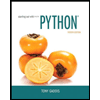
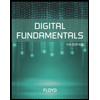
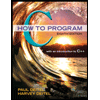
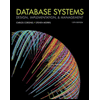
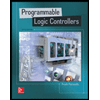