Consider the following classes: public class Animal { public Animal(String itsName) { name = itsName; } public void setName(String itsName) { name = itsName; } public String getName() { return name; } private String name; } public class Dog extends Animal { public Dog(String itsName) { super(itsName + “Dog”); sound = “Bark!”; } public void setSound(String itsSound) { sound = itsSound; } public String getSound() { return (getName(0) + sound); } public String getName(int choice) { if(choice > 0) return super.getName(); else return “AnyDog”; } public String getName() { return super.getName() + “OK”; } private String sound; } In addition, the following lines of code has been placed in a main class public static void main(String[] args) { Animal a1 = new Animal(“Tweety”); Animal a2 = new Dog(“Rover”); Dog d1 = new Dog(“Spot”); . . . . } Give the values of the following expressions. If an expression is erroneous, state why. getName() getName() getName(0)
- Consider the following classes:
public class Animal
{ public Animal(String itsName) { name = itsName; }
public void setName(String itsName) { name = itsName; }
public String getName() { return name; }
private String name;
}
public class Dog extends Animal
{ public Dog(String itsName)
{ super(itsName + “Dog”);
sound = “Bark!”;
}
public void setSound(String itsSound) { sound = itsSound; }
public String getSound() { return (getName(0) + sound); }
public String getName(int choice)
{ if(choice > 0) return super.getName();
else return “AnyDog”; }
public String getName() { return super.getName() + “OK”; }
private String sound;
}
In addition, the following lines of code has been placed in a main class
public static void main(String[] args)
{ Animal a1 = new Animal(“Tweety”);
Animal a2 = new Dog(“Rover”);
Dog d1 = new Dog(“Spot”);
. . . . }
Give the values of the following expressions. If an expression is erroneous, state why.
- getName()
- getName()
- getName(0)
- (new Animal()).getName()
- getName()
- getName(1)
- ((Animal)d1).getName()
- ((Dog)a1).getName()
- d1 instanceof Animal
- a1 instanceof Dog
- getSound()
- getSound()
- getSound()
- ((Dog)a2).getSound()
- ((Animal) d1).getSound()

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

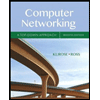
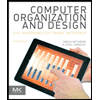
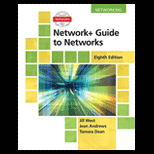
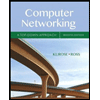
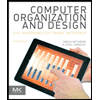
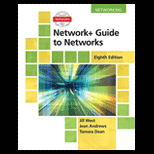
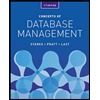
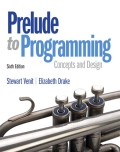
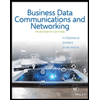