public class PrintPrimes { private static boolean isDivisible (int i, int j) { if (j%i == 0) return true; else return false; } private static void printPrimes (int n) { int curPrime; // Value currently considered for primeness int numPrimes; // Number of primes found so far. boolean isPrime; // Is curPrime prime? int [] primes = new int [100]; // The list of prime numbers. // Initialize 2 into the list of primes. primes [0] = 2; numPrimes = 1; curPrime = 2; while (numPrimes < n) { curPrime++; // next number to consider ... isPrime = true; for (int i = 0; i <= numPrimes-1; i++) { // for each previous prime. if (isDivisible (primes[i], curPrime)) { // Found a divisor, curPrime is not prime. isPrime = false; break; // out of loop through primes. } } if (isPrime) { // save it! primes[numPrimes] = curPrime; numPrimes++; } } // End while // Print all the primes out. for (int i = 0; i <= numPrimes-1; i++) { System.out.println ("Prime: " + primes[i]); } } // end printPrimes public static void main (String []argv) { // Driver method for printPrimes // Read an integer from standard input, call printPrimes() int integer = 0; if (argv.length != 1) { System.out.println ("Usage: java PrintPrimes v1 "); return; } try { integer = Integer.parseInt (argv[0]); } catch (NumberFormatException e) { System.out.println ("Entry must be a integer, using 1."); integer = 1; } printPrimes (integer); } } Use the method printPrimes() for questions a–f below. (a) Draw the control flow graph for the printPrimes() method.(b) Consider test cases t1 = (n = 3) and t2 = (n = 5). Although thesetour the same prime paths in printPrimes(), they do notnecessarily find the same faults. Design a simple fault that t2would be more likely to discover than t1 would.(c) For printPrimes(), find a test case such that thecorresponding test path visits the edge that connects thebeginning of the while statement to the for statement withoutgoing through the body of the while loop.(d) List the test requirements for Node Coverage, Edge Coverage,and Prime Path Coverage.(e) List test paths that achieve Node Coverage but not EdgeCoverage on the graph.(f) List test paths that achieve Edge Coverage but not Prime PathCoverage on the graph
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
public class PrintPrimes { private static boolean isDivisible (int i, int j) { if (j%i == 0) return true; else return false; } private static void printPrimes (int n) { int curPrime; // Value currently considered for primeness int numPrimes; // Number of primes found so far. boolean isPrime; // Is curPrime prime? int [] primes = new int [100]; // The list of prime numbers. // Initialize 2 into the list of primes. primes [0] = 2; numPrimes = 1; curPrime = 2; while (numPrimes < n) { curPrime++; // next number to consider ... isPrime = true; for (int i = 0; i <= numPrimes-1; i++) { // for each previous prime. if (isDivisible (primes[i], curPrime)) { // Found a divisor, curPrime is not prime. isPrime = false; break; // out of loop through primes. } } if (isPrime) { // save it! primes[numPrimes] = curPrime; numPrimes++; } } // End while // Print all the primes out. for (int i = 0; i <= numPrimes-1; i++) { System.out.println ("Prime: " + primes[i]); } } // end printPrimes public static void main (String []argv) { // Driver method for printPrimes // Read an integer from standard input, call printPrimes() int integer = 0; if (argv.length != 1) { System.out.println ("Usage: java PrintPrimes v1 "); return; } try { integer = Integer.parseInt (argv[0]); } catch (NumberFormatException e) { System.out.println ("Entry must be a integer, using 1."); integer = 1; } printPrimes (integer); } }
Use the method printPrimes() for questions a–f below.
(a) Draw the control flow graph for the printPrimes() method.
(b) Consider test cases t1 = (n = 3) and t2 = (n = 5). Although these
tour the same prime paths in printPrimes(), they do not
necessarily find the same faults. Design a simple fault that t2
would be more likely to discover than t1 would.
(c) For printPrimes(), find a test case such that the
corresponding test path visits the edge that connects the
beginning of the while statement to the for statement without
going through the body of the while loop.
(d) List the test requirements for Node Coverage, Edge Coverage,
and Prime Path Coverage.
(e) List test paths that achieve Node Coverage but not Edge
Coverage on the graph.
(f) List test paths that achieve Edge Coverage but not Prime Path
Coverage on the graph
Unlock instant AI solutions
Tap the button
to generate a solution
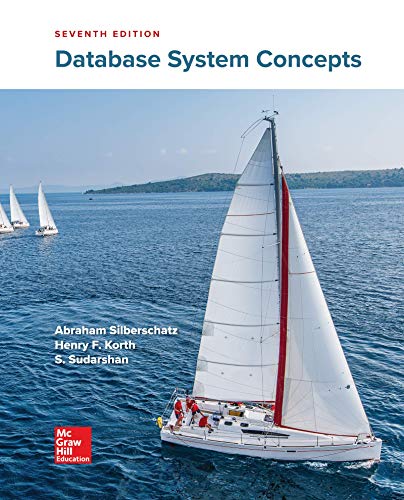
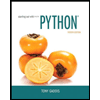
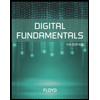
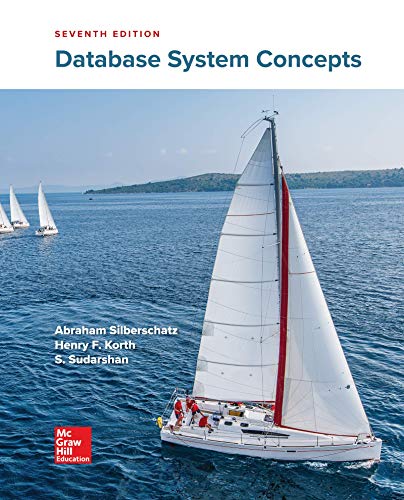
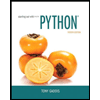
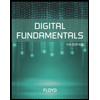
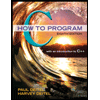
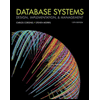
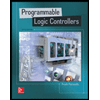