Write a program that simulates tossing a coin. //Prompt the user for how many times to toss the coin. //Code a method with no parameters that randomly returns either the String "heads"or the string "tails". //Call this method in main as many times as requested and report the results.
// Sharissa Sullivan
// COP 2250
// Chapter 6 Method
// Method with No permeters
package sullivan4;
import java.util.*;
public class Sullivan4_2 {
// Define a method for the flip of a coin - heads or tails
class main {
public static String toss()
{
// Create Random Number object for heads/tails toss
Random randomNumber = new Random();
// Generate a random number: heads = 0, tails = 1
int flip = randomNumber.nextInt(2);
// If statement to flag flips with heads or tails
if (flip == 0)
return "heads";
else
return "tails";
}
// Define the main method
public main(final String[] args) { // public static void main(String[] args)
// Create Scanner object
Scanner userinput = new Scanner(System.in);
// Prompt the user to enter how many times they want the coin to be tossed
System.out.println("How many times should I toss the coin ?");
// Scan the value the user enters
int scan = userinput.nextInt();
// Declare variables
int heads = 0;
int tails =0;
int count;
// Create loop for coin toss
for (count = 1; count <= scan ; count++)
{
// toss the coin
String result = toss();
// If statement for toss = heads add 1 to heads count
if (result.equals("heads"))
heads++;
// Else add 1 to tails count
else
tails++;
}
// Print the totals of heads and tails
System.out.println( "Results of "+ scan + "tosses. Heads:" + heads + ",Tails:" + tails);
}
}
}
//Write a program that simulates tossing a coin.
//Prompt the user for how many times to toss the coin.
//Code a method with no parameters that randomly returns either the String "heads"or the string "tails".
//Call this method in main as many times as requested and report the results. See Example outputs below.
***I am getting an error message saying ...Error: Main method not found in class sullivan4.Sullivan4_2, please define the main method as:
public static void main(String[] args)
or a JavaFX application class must extend javafx.application.Application *********
*****What is wrong with my code ?
![### Error Message in Java Console
**Error Message:**
```
Error: Main method not found in class sullivan4.Sullivan4_2, please define the main method as:
public static void main(String[] args)
or a JavaFX application class must extend javafx.application.Application
```
**Explanation:**
This error message indicates that the Java Virtual Machine (JVM) could not find the `main` method in the specified class `sullivan4.Sullivan4_2`. In Java applications, the `main` method serves as the entry point of the application. It must be defined exactly as `public static void main(String[] args)` for a standard Java program.
Alternatively, if this is meant to be a JavaFX application, the class should extend `javafx.application.Application`.
**Steps to Resolve:**
1. **Define the Main Method:**
- If you're creating a console-based application, make sure the `main` method is correctly defined in your class:
```java
public static void main(String[] args) {
// Your code here
}
```
2. **JavaFX Application:**
- If this is a JavaFX application, ensure your class extends `javafx.application.Application` and includes the `start` method:
```java
import javafx.application.Application;
import javafx.stage.Stage;
public class Sullivan4_2 extends Application {
@Override
public void start(Stage primaryStage) {
// Your code here
}
public static void main(String[] args) {
launch(args);
}
}
```
Make the necessary changes to your class based on the type of application you intend to create. If this error persists, verify your classpath and confirm that you are running the correct Java file.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc317763d-1fa7-4bbf-8337-a2804d7c778b%2F95abea03-411f-4acb-9df4-d45a349941f2%2F9o9ikp_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

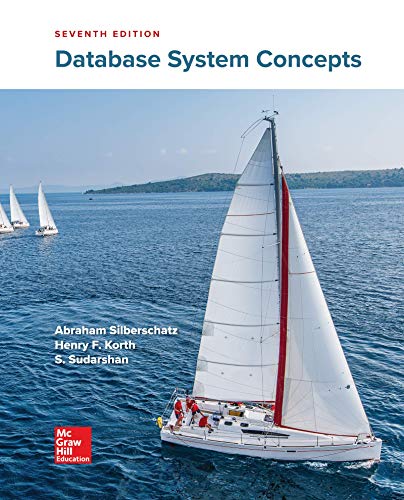
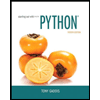
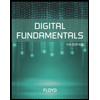
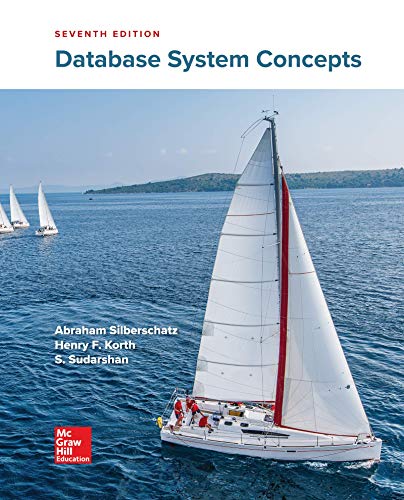
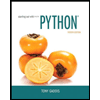
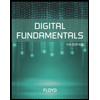
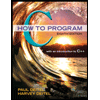
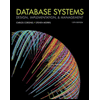
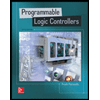