i want to add the givens in the code last questions green to explain and answe
main Application
public class Application {
public static void main(String[] args) {
//Create library object
Library l = new Library();
//Add books
l.add(new Kids("KidsBook1", "author1", 18, 5));
l.add(new Kids("KidsBook2", "author2", 15, 7));
l.add(new Kids("KidsBook3", "author3", 10, 9));
l.add(new Scientific("ScientificBook1", "author1", 12));
l.add(new Scientific("ScientificBook2", "author2", 13));
//Borrower objects
Borrower b1 = new Borrower("Borrower1", 1, 10, 2);
Borrower b2 = new Borrower("Borrower2", 2, 6, 5);
//Borrower1 borrow 2 books
if (b1.getMaxBookNum() <= b1.getSerialNumbers().size()) {
System.out.println("Reached maximum borrowings!!!");
} else {
if (l.borrow(18, b1)) {
b1.borrow(18);
}
}
if (b1.getMaxBookNum() <= b1.getSerialNumbers().size()) {
System.out.println("Reached maximum borrowings!!!");
} else {
if (l.borrow(15, b1)) {
b1.borrow(15);
}
}
//Borrower2 borrow 1 book
if (b2.getMaxBookNum() <= b2.getSerialNumbers().size()) {
System.out.println("Reached maximum borrowings!!!");
} else {
if (l.borrow(10, b2)) {
b2.borrow(10);
}
}
//Return 1 book
l.returnBook(18, b1);
//Borrow check
if (b1.getMaxBookNum() <= b1.getSerialNumbers().size()) {
System.out.println("Reached maximum borrowings!!!");
} else {
if (l.borrow(12, b1)) {
b1.borrow(12);
}
}
//Borrow check
if (b2.getMaxBookNum() <= b2.getSerialNumbers().size()) {
System.out.println("Reached maximum borrowings!!!");
} else {
if (l.borrow(18, b2)) {
b2.borrow(18);
}
}
}
}
class Book
public class Book {
//Attributes
private String name, author;
private int serialNum, age;
// Default Constructor
public Book() {
name = author = "";
serialNum = age = 0;
}
//Parameterized constructor
public Book(String name, String author, int serialNum, int age) {
this.name = name;
this.author = author;
this.serialNum = serialNum;
this.age = age;
}
//Getters and setters
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public int getSerialNum() {
return serialNum;
}
public void setSerialNum(int serialNum) {
this.serialNum = serialNum;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
@Override
public String toString() {
return "[name=" + name + ", author=" + author + ", serialNum=" + serialNum + ", age=" + age + "]";
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
Book other = (Book) obj;
if (age != other.age) {
return false;
}
if (author == null) {
if (other.author != null) {
return false;
}
} else if (!author.equals(other.author)) {
return false;
}
if (name == null) {
if (other.name != null) {
return false;
}
} else if (!name.equals(other.name)) {
return false;
}
if (serialNum != other.serialNum) {
return false;
}
return true;
}
}
Scientific
public class Scientific extends Book {
//Default constructor
public Scientific() {
super();
}
//Parameterized constructor
public Scientific(String name, String author, int serialNum) {
super(name, author, serialNum, 18);
}
}
Kids
public class Kids extends Book {
//Default constructor
public Kids() {
super();
}
//Parameterized constructor
public Kids(String name, String author, int serialNum, int age) {
super(name, author, serialNum, age);
}
}
Borrower
public class Borrower {
//Attributes
private String name;
private int id, age, maxBookNum;
private ArrayList<Integer> serialNumbers;
//Default constructor
public Borrower() {
name = "";
id = age = maxBookNum = 0;
serialNumbers = new ArrayList<Integer>();
}
//Parameterized constructor
public Borrower(String name, int id, int age, int maxNum) {
this.name = name;
this.id = id;
this.age = age;
this.maxBookNum = maxNum;
serialNumbers = new ArrayList<Integer>();
}
//Setters and getters
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public int getMaxBookNum() {
return maxBookNum;
}
public void setMaxBookNum(int maxBookNum) {
this.maxBookNum = maxBookNum;
}
public ArrayList<Integer> getSerialNumbers() {
return serialNumbers;
}
public void borrow(int sn) {
serialNumbers.add(sn);
}
public void bookReturn(int sn) {
serialNumbers.remove(serialNumbers.indexOf(sn));
}
}
i want to add the givens in the code
last questions green to explain and answer


Step by step
Solved in 2 steps

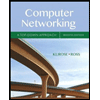
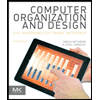
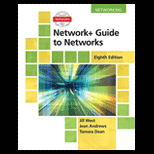
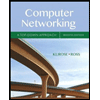
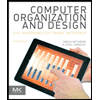
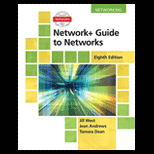
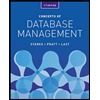
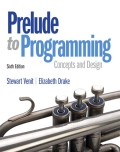
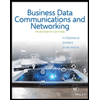