1. Add a field to the Hexagon.java class called side. It should be a double. 2. Write a no arguments constructor. Set side = 0.0 3. Write a constructor that accepts a double parameter. Set side equal to the value passed in. 4. Write accessor/muteator methods for the field side 5. Write a method (getPerimeter0) that returns a double and calculates the perimeter of the Hexagon. The formula for this is: perimeter = side * 6 6. Write a method (getDiagonalLength0) that returns a double that is the distance from one 'corner' to its opposite 'corner. The formula for this is: diagonalLength = side * 2 7. Write a method (getArea) which returns a double containing the surface area of the hexagon. The formula for this is (3/2 * sqrt(3) * side ^ 2). Take care with integer division here.
1. Add a field to the Hexagon.java class called side. It should be a double. 2. Write a no arguments constructor. Set side = 0.0 3. Write a constructor that accepts a double parameter. Set side equal to the value passed in. 4. Write accessor/muteator methods for the field side 5. Write a method (getPerimeter0) that returns a double and calculates the perimeter of the Hexagon. The formula for this is: perimeter = side * 6 6. Write a method (getDiagonalLength0) that returns a double that is the distance from one 'corner' to its opposite 'corner. The formula for this is: diagonalLength = side * 2 7. Write a method (getArea) which returns a double containing the surface area of the hexagon. The formula for this is (3/2 * sqrt(3) * side ^ 2). Take care with integer division here.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Java
class Main {
publicstaticvoid main(String[] args) {
/*
* Use this code to test your Hexagon class.
* Uncomment the items as you complete parts of the Hexagon class.
*/
Hexagon myHex = new Hexagon();
// Hexagon myOtherHex = new Hexagon(5);
// // print info about myHex
// System.out.println("Information about myHex");
// displayHex(myHex);
// System.out.println();
// // print info about myOtherHex
// System.out.println("Information about myOtherHex");
// displayHex(myOtherHex);
// System.out.println();
// myHex.setSide(3);
// // print info about myHex after changing side length
// System.out.println("Information about myHex with side length 3");
// displayHex(myHex);
}
publicstaticvoid displayHex(Hexagon h) {
// System.out.println("Side length: " + h.getSide());
// System.out.println("Perimeter: " + h.getPerimeter());
// System.out.println("Diagonal length: " + h.getDiagonalLength());
// System.out.println("Surface area: " + h.getArea());
}
}

Transcribed Image Text:A hexagon:
120°
60
Steps
1. Add a field to the Hexagon.java class called side. It should be a double.
2. Write a no arguments constructor. Set side = 0.0
3. Write a constructor that accepts a double parameter. Set side equal to the value passed in.
4. Write accessor/mutator methods for the field side
5. Write a method (getPerimeter0) that returns a double and calculates the perimeter of the Hexagon.
The formula for this is: perimeter = side * 6
6. Write a method (getDiagonallength() that returns a double that is the distance from one 'corner' to its
opposite 'corner'. The formula for this is: diagonallength = side * 2
7. Write a method (getArea0) which returns a double containing the surface area of the hexagon. The
formula for this is (3/2 * sqrt(3) * side ^ 2). Take care with integer division here.

Transcribed Image Text:Results
When complete, running the test code should generate the following output:
Information about myHex
side length: 0.0
Perimeter: 0.0
Diagonal length: 0.0
Surface area: 0.0
Information about myotherHex
side length: 5.0
Perimeter: s0.0
Diagonal length: 10.0
Surface area: 64.9519652858529
Information about myHex with side length s
side length: 3.0
Perimeter: 18.0
Diagonal length: 6.0
Surface area: 23.882685962179844
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
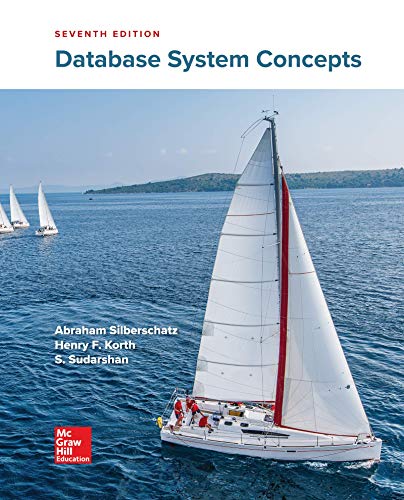
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
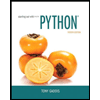
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
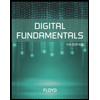
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
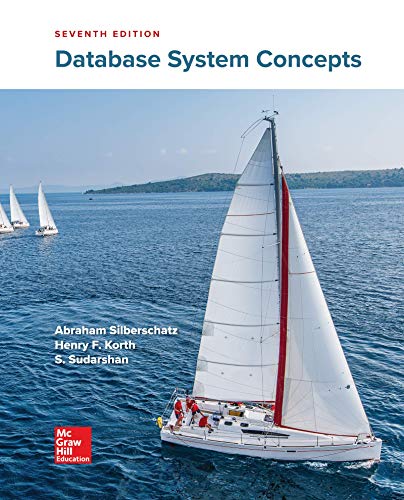
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
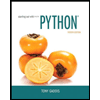
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
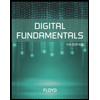
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
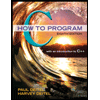
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
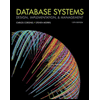
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
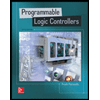
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education