Consider the following classes Number and NumberPair 1 public class Number 2 { 3 public int num; //public to access it from objects of other classes 4 5 public Number(int number) 6 { 7 num = number; 8 } 9 } 10 //--------------------------------------------------------- 11 public class NumberPair 12 { 13 private Number n1,n2; //fields of the class are n1 and n2 of type Number 14 15 public NumberPair() 16 { 17 n1 = new Number(5); 18 n2 = new Number(10); 19 } 20 21 22 public void swapObjects(Number number1, Number number2) 23 { 24 Number tmp = number1; 25 number1 = number2; 26 number2 = tmp; 27 } 28 29 public void test() 30 { 31 swapObjects(n1,n2); 32 } 33 } 1. Assume we create an instance NumberPair pair = new NumberPair(); What will be the value of pair.n1.num and pair.n2.num, after calling method pair.test()? Explain your answer! 2. Write a method swapFields(Number number1, Number number2) that swaps the values of the fields num of two Number obj
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Consider the following classes Number and NumberPair
1 public class Number
2 {
3 public int num; //public to access it from objects of other classes
4
5 public Number(int number)
6 {
7 num = number;
8 }
9 }
10 //---------------------------------------------------------
11 public class NumberPair
12 {
13 private Number n1,n2; //fields of the class are n1 and n2 of type Number
14
15 public NumberPair()
16 {
17 n1 = new Number(5);
18 n2 = new Number(10);
19 }
20
21
22 public void swapObjects(Number number1, Number number2)
23 {
24 Number tmp = number1;
25 number1 = number2;
26 number2 = tmp;
27 }
28
29 public void test()
30 {
31 swapObjects(n1,n2);
32 }
33 }
1. Assume we create an instance NumberPair pair = new NumberPair();
What will be the value of pair.n1.num and pair.n2.num, after calling method pair.test()? Explain your
answer!
2. Write a method swapFields(Number number1, Number number2) that swaps the values of the fields num of two
Number obj

Step by step
Solved in 3 steps with 3 images

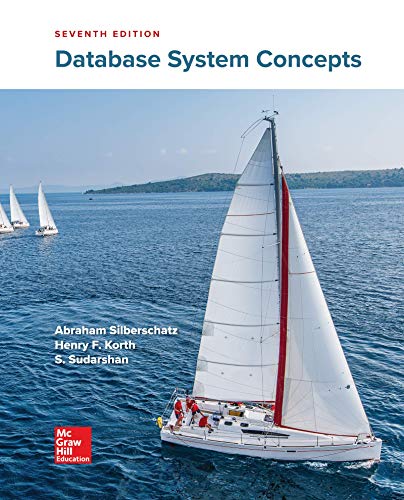
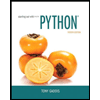
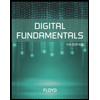
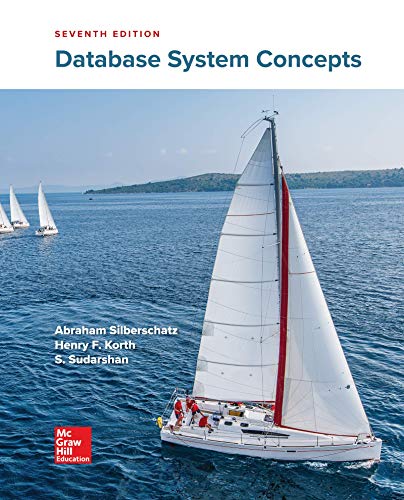
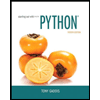
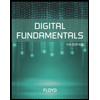
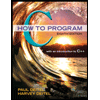
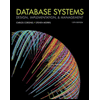
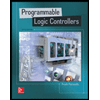